Flutter – Autocomplete Widget
Last Updated :
07 Jun, 2023
In this article, we will learn how to develop an autocomplete feature in Flutter that allows users to quickly search and select items from a large set of data. To demonstrate this feature, we will walk through a demo program step-by-step. This functionality can be achieved using the autocomplete widget in Flutter. A sample video is given below to get an idea about what we are going to do in this article.
Step By Step Implementation
Step 1: Open your VS-code create a Flutter Application And Installing Module using the following steps:
- Open the Command Palette (Ctrl+Shift+P (Cmd+Shift+P on macOS)).
- Select the Flutter: New Project command and press Enter.
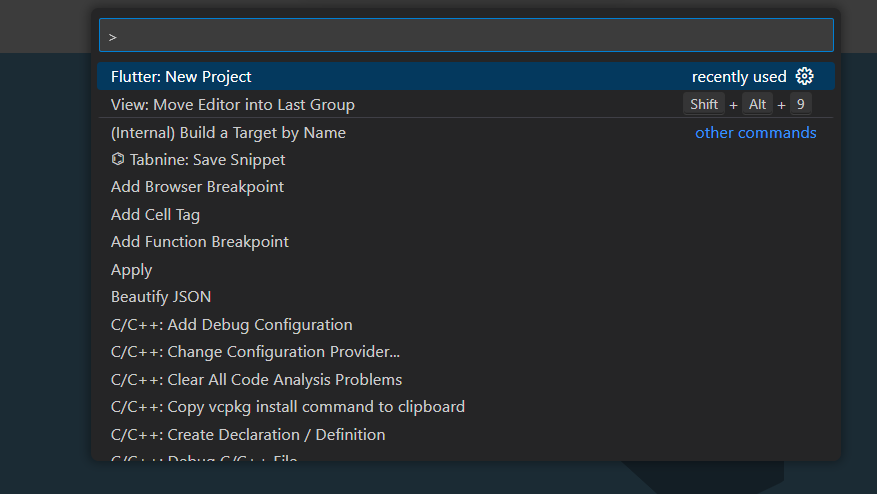
Â
Select Application and press Enter.
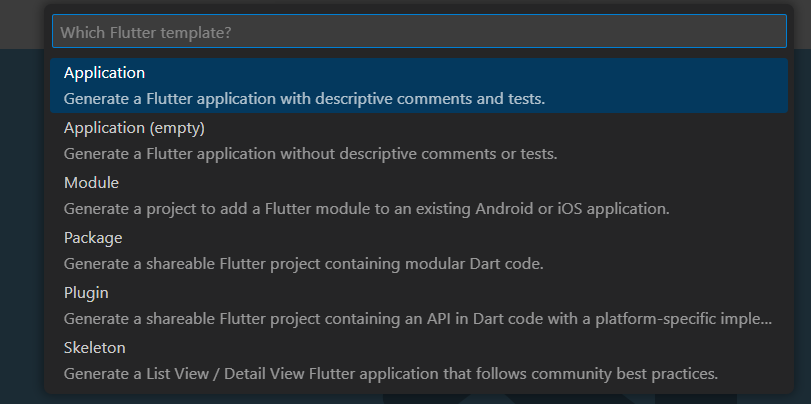
Â
- Select a Project location.
- Enter your desired Project name.

Â
Now your project structure looks like this:
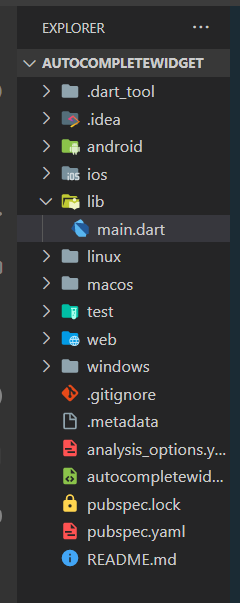
Â
Step 2: Create a stateless widget by writing the following code inside your main.dart file
Dart
import 'package:flutter/material.dart' ;
void main() {
runApp( const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Autocomplete widget Demo' ,
theme: ThemeData(
primarySwatch: Colors.deepPurple,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
static const List<String> _fruitOptions = <String>[
'apple' ,
'banana' ,
'orange' ,
'mango' ,
'grapes' ,
'watermelon' ,
'kiwi' ,
'strawberry' ,
'sugarcane' ,
];
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text( "AutoComplete widget" ),
),
body: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
const Text( "enter Fruit name:" ),
Autocomplete<String>(
optionsBuilder: (TextEditingValue fruitTextEditingValue) {
if (fruitTextEditingValue.text == '' ) {
return const Iterable<String>.empty();
}
return _fruitOptions.where((String option) {
return option
.contains(fruitTextEditingValue.text.toLowerCase());
});
},
onSelected: (String value) {
debugPrint( 'You just selected $value' );
},
),
],
),
);
}
}
|
Now let’s understand the complete code:
import 'package:flutter/material.dart';
We import flutter/material.dart package so that we can implement the material design, we can use widgets like stateless widgets, materialApp, etc.
void main() {
runApp(const MyApp());
}
Every flutter code has an entry point from the main function, inside the main function we call the runApp method provided by ‘flutter/material.dart’ Â package and we pass the widget MyApp as a parameter.
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Autocomplete widget Demo',
theme: ThemeData(
primarySwatch: Colors.deepPurple,
),
home: const MyHomePage(),
);
}
}
Now we create our stateless widget MyApp by extending the stateless widget class provided by the material.dart package, then we override the build method implemented in statelessWidget class and return the materialApp widget with the required parameters.
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
static const List<String> _fruitOptions = <String>[
'apple',
'banana',
'orange',
'mango',
'grapes',
'watermelon',
'kiwi',
'strawberry',
'sugarcane',
];
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text("AutoComplete widget"),
),
body: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
const Text("enter Fruit name:"),
Autocomplete<String>(
optionsBuilder: (TextEditingValue fruitTextEditingValue) {
if (fruitTextEditingValue.text == '') {
return const Iterable<String>.empty();
}
return _fruitOptions.where((String option) {
return option
.contains(fruitTextEditingValue.text.toLowerCase());
});
},
onSelected: (String value) {
debugPrint('You just selected $value');
},
),
],
),
);
}
}
For creating the HomeScreen we write the stateless widget called MyHomePage, inside the MyHomePage we use the Autocomplete Widget that takes OptionsBuilder and OnSelected as parameters.
Step 3: Run the following code by selecting the Android emulator or on your physical device by connecting it.
Output Screenshots:
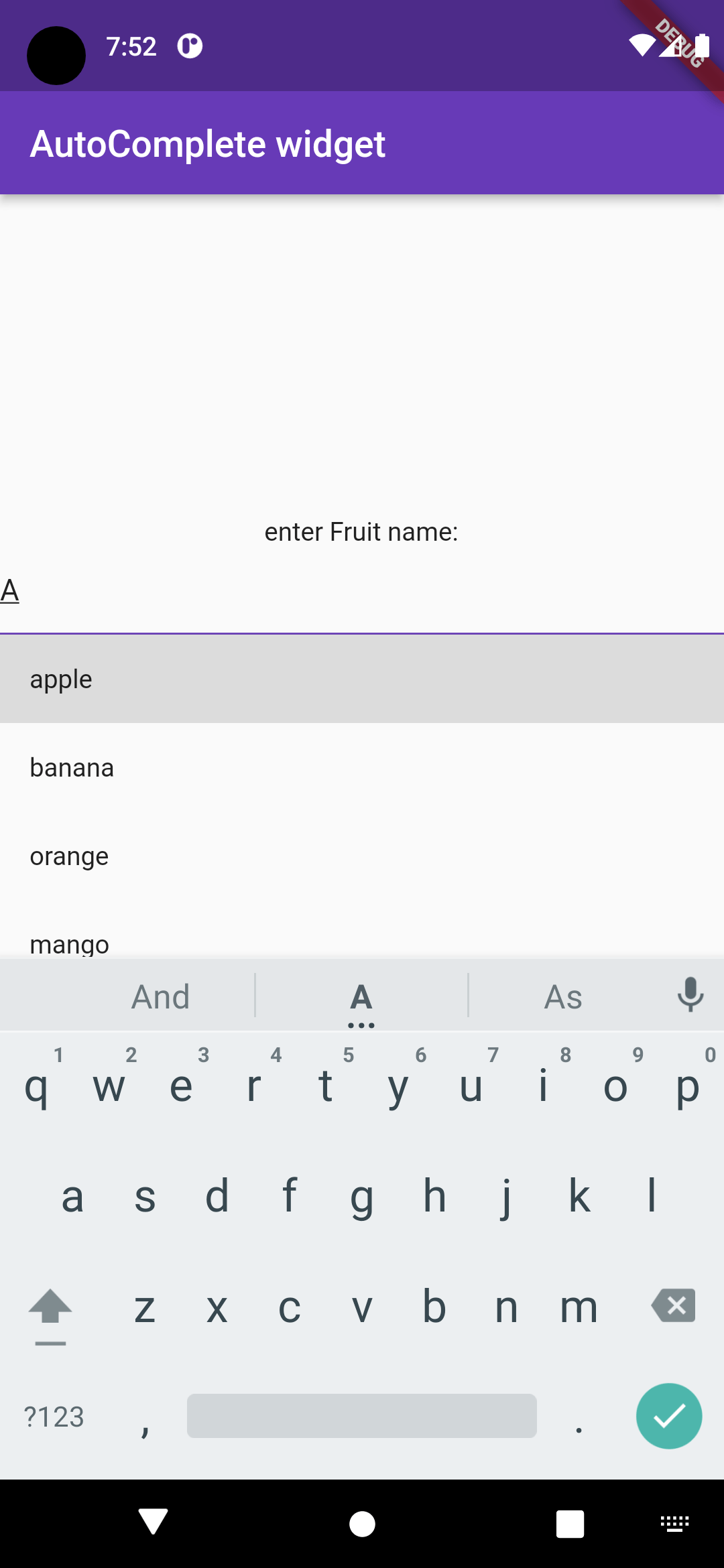
Â
Â
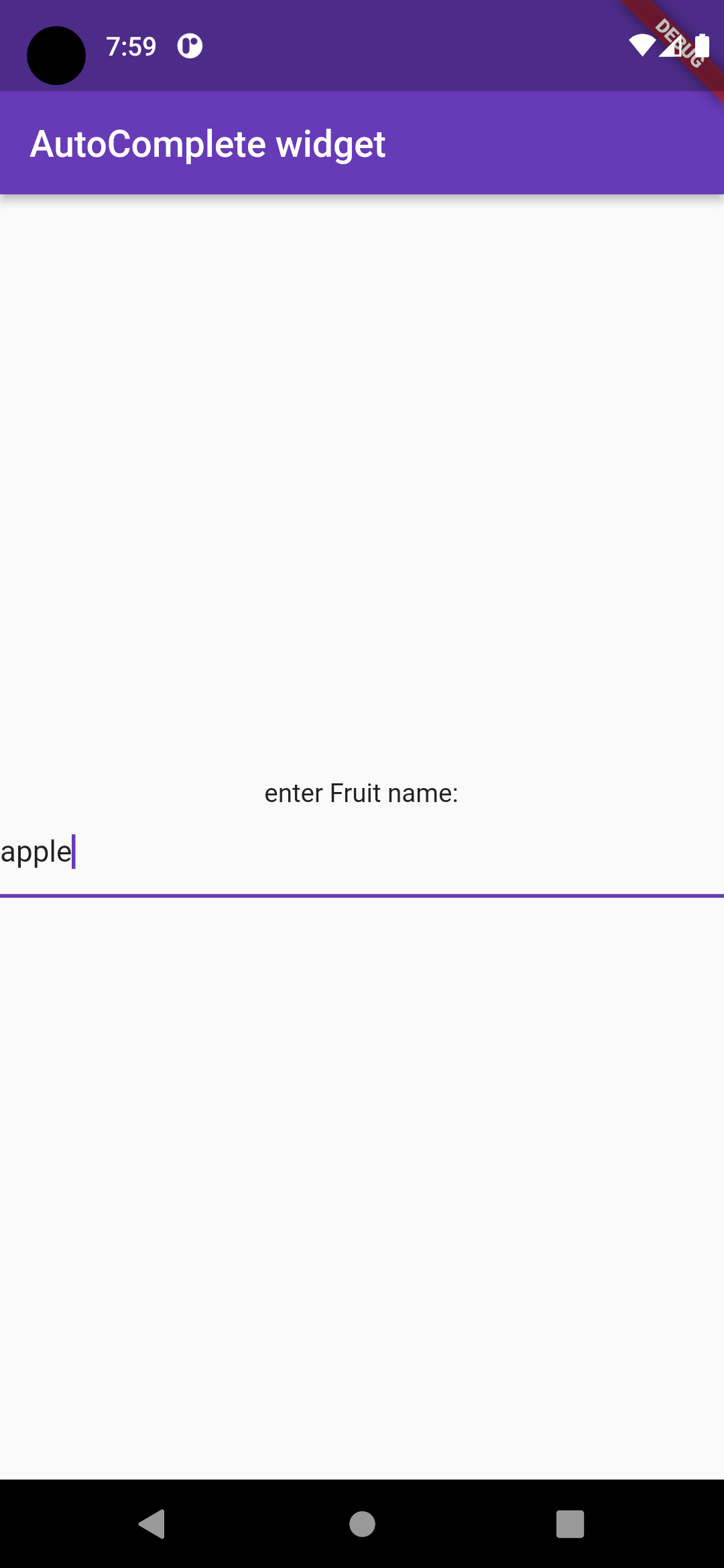
Â
Output Videos:
Share your thoughts in the comments
Please Login to comment...