Find the Solidity and Equivalent Diameter of an Image Object Using OpenCV Python
Last Updated :
30 Dec, 2022
In this article, we will see how we can find the solidity and the equivalent diameter of an object present in an image with help of Python OpenCV.
Function to Find Solidity
The solidity of an image is the measurement of the overall concavity of a particle. We can define the solidity of an object as the ratio of the contour area to its convex hull area. The Contour area and convex hull area should be determined first in order to compute the solidity.
Python3
def find_solidity(count):
contourArea = cv2.contourArea(count)
convexHull = cv2.convexHull(count)
contour_hull_area = cv2.contourArea(convexHull)
solidity = float (contourArea) / contour_hull_area
return solidity
|
Function to Find Equivalent Diameter
Also, the diameter of the circle whose area is equal to the contour area is known as the Equivalent Diameter. The Contour is an outline representing or bounding the shape or form of something.
Python3
def find_equi_diameter(count):
area = cv2.contourArea(count)
equi_diameter = np.sqrt( 4 * area / np.pi)
return equi_diameter
|
Steps to find the Solidity and the Equivalent Diameter:
- First, we need to import python’s OpenCV library and NumPy library.
- Use the function cv2.imread() to read the desired image.
- Create a binary image by applying thresholding on the grayscale image.
- Use the cv2.findContours() function to find the contours in the image.
- Compute the Solidity and the Equivalent Diameter for that we will priorly calculate the contour area and convex hull area.
- Finally, print the Solidity and the Equivalent Diameter.
Solidity and Equivalent Diameter of an Image with Single Object
We will determine the solidity and equivalent diameter of a single object in the picture using below Python program. The solidity and equivalent diameter values for the item will be printed on the console.
Python3
import cv2
import numpy as np
img = cv2.imread(r "C:\Users\siddh\Downloads\star2.png" )
grayScaleImg = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
ret, thresh = cv2.threshold(grayScaleImg, 40 , 255 , 0 )
contours, hierarchy = cv2.findContours(thresh,
cv2.RETR_TREE,
cv2.CHAIN_APPROX_SIMPLE)
print (f "{len(contours)} objects detected" )
count = contours[ 0 ]
Solidity = find_solidity(count)
Solidity = round (Solidity, 2 )
equi_diameter = find_equi_diameter(count)
equi_diameter = round (equi_diameter, 2 )
print ( "Solidity - " , Solidity)
print ( "Equivalent Diameter - " , equi_diameter)
|
Input Image:
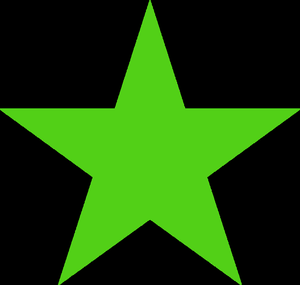
star.png
Output:
1 objects detected
Solidity - 0.48
Equivalent Diameter - 511.62
Solidity and Equivalent Diameter of an Image with Multiple Objects
We will determine the solidity and equivalent diameter of multiple objects in the picture using below Python program. The solidity and equivalent diameter values for the item will be printed on the console.
Python3
import cv2
import numpy as np
img = cv2.imread(r "C:\Users\siddh\Downloads\multshapes2.png" )
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
ret, thresh = cv2.threshold(gray, 100 , 255 , 0 )
contours, hierarchy = cv2.findContours(thresh, 1 , 2 )
print ( "Number of objects detected:" , len (contours))
|
Now we will iteratively find the solidity and the equivalent diameter for each of the objects which are detected in the image.
Python3
for i, cnt in enumerate (contours):
Solidity = find_solidity(cnt)
Solidity = round (Solidity, 2 )
equi_diameter = find_equi_diameter(cnt)
equi_diameter = round (equi_diameter, 2 )
print (f "Solidity of object {i+1}: " , Solidity)
print (f "Equivalent Diameter of object {i+1}: " , equi_diameter)
|
input Image:
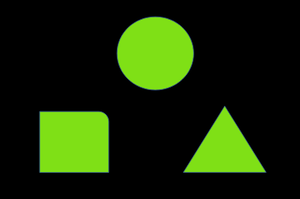
multiple_shapes.png
Output:
Number of objects detected: 3
Solidity of object 1: 1.0
Equivalent Diameter of object 1: 90.93
Solidity of object 2: 0.98
Equivalent Diameter of object 2: 73.14
Solidity of object 3: 0.99
Equivalent Diameter of object 3: 93.43
Share your thoughts in the comments
Please Login to comment...