Find path traveling which bishop traverse each black cell atleast once
Last Updated :
06 Apr, 2023
Given two integers X and Y such that X+Y is even, Which denotes the initial position of the bishop on a chessboard of 8 x 8 dimension. Then the task is to output a continuous path with not more than 32 coordinates(total black cells on board) such that traveling on that path bishop covers all the cells (i, j) having even sum (i + j = even). These cells need to be covered at least once over the course of travel, return the number of coordinates taken in the path, and the coordinates of the path to be followed.
Note: A single cell can be traversed more than once.
Examples:
Input: X = 5, Y = 3
Output:
19
5 3
4 4
1 1
8 8
7 7
8 6
3 1
1 3
6 8
5 7
4 8
1 5
5 1
8 4
7 3
8 2
7 1
1 7
2 8
Explanation:
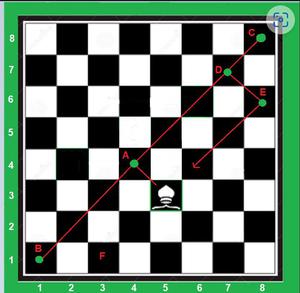
Graphical Explanation of input case 1
Initially, the bishop is placed at (5, 3). Then it moves as follows: A (4, 4) -> B (1, 1) -> C (8, 8 ) -> D (7, 7) -> E (8, 6) -> F (3, 1) and so on. So, In total there are 19 moves, Which are shown in the output. Following this output coordinates all the cells (i, j) having even sum, ie. (i + j) = even, will be traversed. Formally, all black cells will be traversed.
Input: X =1, Y = 1
Output:
17
1 1
8 8
7 7
8 6
. . . . and so on.
Explanation: In total there will be 17 moves, and the starting of the path is as: (1, 1) -> (8, 8) -> (7, 7) -> (8, 6) and so on . . . .
Approach: Implement the idea below to solve the problem:
The problem is observation based and can be solved by using those observations. There exists a common path that applies for all the inputs of X and Y. By thinking about this problem deeply, we can observe a common path.
Steps were taken to solve the problem:
- Create a method commonPath() containing the below co-ordinates:
- (1, 1)
(8, 8)
(7, 7)
(8, 6)
(3, 1)
(1, 3)
(6, 8)
(5, 7)
(4, 8)
(1, 5)
(5, 1)
(8, 4)
(7, 3)
(8, 2)
(7, 1)
(1, 7)
(2, 8)
- If ( X == Y && X == 1 ) then print all the points of the common_path() method.
- Else if ( X == Y && X != 1 ) then print 18 coordinates, Formally (X, Y) with all the points of the common_path() method.
- Else print 19 coordinates, Formally (X, Y), ( (X+Y)/2, (X+Y)/2 ) all the points of common_path() method.
Below is the code to implement the approach:
C++
#include<iostream>
using namespace std;
void pathPrinter( int X, int Y)
{
if (X == Y && X == 1) {
cout << 17 << endl;
}
else if (X == Y && X != 1) {
cout << 18 << endl;
cout << X << " " << Y << endl;
}
else {
cout << 19 << endl;
cout << X << " " << Y << endl;
int d = (X + Y) / 2;
cout << d << " " << d << endl;
}
}
void commonPath()
{
cout << "1 1" << endl;
cout << "8 8" << endl;
cout << "7 7" << endl;
cout << "8 6" << endl;
cout << "3 1" << endl;
cout << "1 3" << endl;
cout << "6 8" << endl;
cout << "5 7" << endl;
cout << "4 8" << endl;
cout << "1 5" << endl;
cout << "5 1" << endl;
cout << "8 4" << endl;
cout << "7 3" << endl;
cout << "8 2" << endl;
cout << "7 1" << endl;
cout << "1 7" << endl;
cout << "2 8" << endl;
}
int main()
{
int X = 6;
int Y = 2;
pathPrinter(X, Y);
commonPath();
}
|
Java
import java.io.*;
import java.lang.*;
import java.util.*;
class GFG {
public static void main(String[] args)
{
int X = 6 ;
int Y = 2 ;
pathPrinter(X, Y);
}
static void pathPrinter( int X, int Y)
{
if (X == Y && X == 1 ) {
System.out.println( 17 );
commonPath();
}
else if (X == Y && X != 1 ) {
System.out.println( 18 );
System.out.println(X + " " + Y);
commonPath();
}
else {
System.out.println( 19 );
System.out.println(X + " " + Y);
int d = (X + Y) / 2 ;
System.out.println(d + " " + d);
commonPath();
}
}
public static void commonPath()
{
System.out.println( 1 + " " + 1 );
System.out.println( 8 + " " + 8 );
System.out.println( 7 + " " + 7 );
System.out.println( 8 + " " + 6 );
System.out.println( 3 + " " + 1 );
System.out.println( 1 + " " + 3 );
System.out.println( 6 + " " + 8 );
System.out.println( 5 + " " + 7 );
System.out.println( 4 + " " + 8 );
System.out.println( 1 + " " + 5 );
System.out.println( 5 + " " + 1 );
System.out.println( 8 + " " + 4 );
System.out.println( 7 + " " + 3 );
System.out.println( 8 + " " + 2 );
System.out.println( 7 + " " + 1 );
System.out.println( 1 + " " + 7 );
System.out.println( 2 + " " + 8 );
}
}
|
Python3
def pathPrinter(X, Y):
if X = = Y and X = = 1 :
print ( 17 )
elif X = = Y and X ! = 1 :
print ( 18 )
print (X, Y)
else :
print ( 19 )
print (X, Y)
d = (X + Y) / / 2
print (d, d)
def commonPath():
print ( "1 1" )
print ( "8 8" )
print ( "7 7" )
print ( "8 6" )
print ( "3 1" )
print ( "1 3" )
print ( "6 8" )
print ( "5 7" )
print ( "4 8" )
print ( "1 5" )
print ( "5 1" )
print ( "8 4" )
print ( "7 3" )
print ( "8 2" )
print ( "7 1" )
print ( "1 7" )
print ( "2 8" )
X = 6
Y = 2
pathPrinter(X, Y)
commonPath()
|
C#
using System;
public class GFG {
static void pathPrinter( int X, int Y)
{
if (X == Y && X == 1) {
Console.WriteLine(17);
commonPath();
}
else if (X == Y && X != 1) {
Console.WriteLine(18);
Console.WriteLine(X + " " + Y);
commonPath();
}
else {
Console.WriteLine(19);
Console.WriteLine(X + " " + Y);
int d = (X + Y) / 2;
Console.WriteLine(d + " " + d);
commonPath();
}
}
public static void commonPath()
{
Console.WriteLine(1 + " " + 1);
Console.WriteLine(8 + " " + 8);
Console.WriteLine(7 + " " + 7);
Console.WriteLine(8 + " " + 6);
Console.WriteLine(3 + " " + 1);
Console.WriteLine(1 + " " + 3);
Console.WriteLine(6 + " " + 8);
Console.WriteLine(5 + " " + 7);
Console.WriteLine(4 + " " + 8);
Console.WriteLine(1 + " " + 5);
Console.WriteLine(5 + " " + 1);
Console.WriteLine(8 + " " + 4);
Console.WriteLine(7 + " " + 3);
Console.WriteLine(8 + " " + 2);
Console.WriteLine(7 + " " + 1);
Console.WriteLine(1 + " " + 7);
Console.WriteLine(2 + " " + 8);
}
static public void Main()
{
int X = 6;
int Y = 2;
pathPrinter(X, Y);
}
}
|
Javascript
function pathPrinter(X, Y) {
if (X === Y && X === 1) {
console.log(17);
} else if (X === Y && X !== 1) {
console.log(18);
console.log(X + " " + Y);
} else {
console.log(19);
console.log(X + " " + Y);
let d = Math.floor((X + Y) / 2);
console.log(d + " " + d);
}
}
function commonPath() {
console.log( "1 1" );
console.log( "8 8" );
console.log( "7 7" );
console.log( "8 6" );
console.log( "3 1" );
console.log( "1 3" );
console.log( "6 8" );
console.log( "5 7" );
console.log( "4 8" );
console.log( "1 5" );
console.log( "5 1" );
console.log( "8 4" );
console.log( "7 3" );
console.log( "8 2" );
console.log( "7 1" );
console.log( "1 7" );
console.log( "2 8" );
}
function main() {
let X = 6;
let Y = 2;
pathPrinter(X, Y);
commonPath();
}
main();
|
Output
19
6 2
4 4
1 1
8 8
7 7
8 6
3 1
1 3
6 8
5 7
4 8
1 5
5 1
8 4
7 3
8 2
7 1
1 7
2 8
Time Complexity: O(1)
Auxiliary Space: O(1)
Share your thoughts in the comments
Please Login to comment...