Find an equal point in a string of brackets
Last Updated :
19 Sep, 2023
Given a string of brackets, the task is to find an index k which decides the number of opening brackets is equal to the number of closing brackets.
The string must be consists of only opening and closing brackets i.e. ‘(‘ and ‘)’.
An equal point is an index such that the number of opening brackets before it is equal to the number of closing brackets from and after.
Examples:
Input: str = “(())))(“
Output: 4
Explanation: After index 4, string splits into (()) and ))(. The number of opening brackets in the first part is equal to the number of closing brackets in the second part.
Input: str = “))”
Output: 2
Explanation: As after 2nd position i.e. )) and “empty” string will be split into these two parts. So, in this number of opening brackets i.e. 0 in the first part is equal to the number of closing brackets in the second part i.e. also 0.
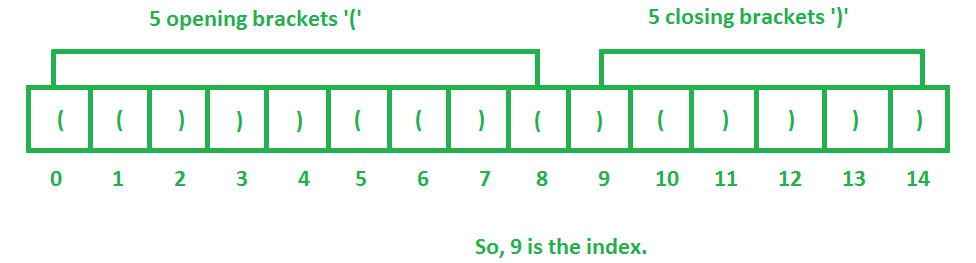
Asked in: Amazon
Approach 1:
- Store the number of opening brackets that appear in the string up to every index, it must start from starting index.
- Similarly, Store the number of closing brackets that appear in the string up to each and every index but it should be done from the last index.
- Check if any index has the same value as opening and closing brackets.
Below is the implementation of the above approach:
C++
#include<bits/stdc++.h>
using namespace std;
int findIndex(string str)
{
int len = str.length();
int open[len+1], close[len+1];
int index = -1;
memset (open, 0, sizeof (open));
memset (close, 0, sizeof (close));
open[0] = 0;
close[len] = 0;
if (str[0]== '(' )
open[1] = 1;
if (str[len-1] == ')' )
close[len-1] = 1;
for ( int i = 1; i < len; i++)
{
if ( str[i] == '(' )
open[i+1] = open[i] + 1;
else
open[i+1] = open[i];
}
for ( int i = len-2; i >= 0; i--)
{
if ( str[i] == ')' )
close[i] = close[i+1] + 1;
else
close[i] = close[i+1];
}
if (open[len] == 0)
return len;
if (close[0] == 0)
return 0;
for ( int i=0; i<=len; i++)
if (open[i] == close[i])
index = i;
return index;
}
int main()
{
string str = "(()))(()()())))" ;
cout << findIndex(str);
return 0;
}
|
Java
public class GFG
{
static int findIndex(String str)
{
int len = str.length();
int open[] = new int [len+ 1 ];
int close[] = new int [len+ 1 ];
int index = - 1 ;
open[ 0 ] = 0 ;
close[len] = 0 ;
if (str.charAt( 0 )== '(' )
open[ 1 ] = 1 ;
if (str.charAt(len- 1 ) == ')' )
close[len- 1 ] = 1 ;
for ( int i = 1 ; i < len; i++)
{
if ( str.charAt(i) == '(' )
open[i+ 1 ] = open[i] + 1 ;
else
open[i+ 1 ] = open[i];
}
for ( int i = len- 2 ; i >= 0 ; i--)
{
if ( str.charAt(i) == ')' )
close[i] = close[i+ 1 ] + 1 ;
else
close[i] = close[i+ 1 ];
}
if (open[len] == 0 )
return len;
if (close[ 0 ] == 0 )
return 0 ;
for ( int i= 0 ; i<=len; i++)
if (open[i] == close[i])
index = i;
return index;
}
public static void main(String[] args)
{
String str = "(()))(()()())))" ;
System.out.println(findIndex(str));
}
}
|
Python3
def findIndex( str ):
l = len ( str )
open = [ 0 ] * (l + 1 )
close = [ 0 ] * (l + 1 )
index = - 1
open [ 0 ] = 0
close[l] = 0
if ( str [ 0 ] = = '(' ):
open [ 1 ] = 1
if ( str [l - 1 ] = = ')' ):
close[l - 1 ] = 1
for i in range ( 1 , l):
if ( str [i] = = '(' ):
open [i + 1 ] = open [i] + 1
else :
open [i + 1 ] = open [i]
for i in range (l - 2 , - 1 , - 1 ):
if ( str [i] = = ')' ):
close[i] = close[i + 1 ] + 1
else :
close[i] = close[i + 1 ]
if ( open [l] = = 0 ):
return len
if (close[ 0 ] = = 0 ):
return 0
for i in range (l + 1 ):
if ( open [i] = = close[i]):
index = i
return index
str = "(()))(()()())))"
print (findIndex( str ))
|
C#
using System;
class GFG
{
static int findIndex( string str)
{
int len = str.Length;
int [] open = new int [len + 1];
int [] close = new int [len + 1];
int index = -1;
open[0] = 0;
close[len] = 0;
if (str[0] == '(' )
open[1] = 1;
if (str[len - 1] == ')' )
close[len - 1] = 1;
for ( int i = 1; i < len; i++)
{
if (str[i] == '(' )
open[i + 1] = open[i] + 1;
else
open[i + 1] = open[i];
}
for ( int i = len - 2; i >= 0; i--)
{
if (str[i] == ')' )
close[i] = close[i + 1] + 1;
else
close[i] = close[i + 1];
}
if (open[len] == 0)
return len;
if (close[0] == 0)
return 0;
for ( int i = 0; i <= len; i++)
if (open[i] == close[i])
index = i;
return index;
}
public static void Main()
{
string str = "(()))(()()())))" ;
Console.Write(findIndex(str));
}
}
|
PHP
<?php
function findIndex( $str )
{
$len = strlen ( $str );
$open = array (0, $len + 1, NULL);
$close = array (0, $len + 1, NULL);
$index = -1;
$open [0] = 0;
$close [ $len ] = 0;
if ( $str [0] == '(' )
$open [1] = 1;
if ( $str [ $len - 1] == ')' )
$close [ $len - 1] = 1;
for ( $i = 1; $i < $len ; $i ++)
{
if ( $str [ $i ] == '(' )
$open [ $i + 1] = $open [ $i ] + 1;
else
$open [ $i + 1] = $open [ $i ];
}
for ( $i = $len - 2; $i >= 0; $i --)
{
if ( $str [ $i ] == ')' )
$close [ $i ] = $close [ $i + 1] + 1;
else
$close [ $i ] = $close [ $i + 1];
}
if ( $open [ $len ] == 0)
return $len ;
if ( $close [0] == 0)
return 0;
for ( $i = 0; $i <= $len ; $i ++)
if ( $open [ $i ] == $close [ $i ])
$index = $i ;
return $index ;
}
$str = "(()))(()()())))" ;
echo (findIndex( $str ));
?>
|
Javascript
<script>
function findIndex(str)
{
let len = str.length;
let open = new Array(len + 1);
let close = new Array(len + 1);
for (let i = 0; i < len + 1; i++)
{
open[i] = 0;
close[i] = 0;
}
let index = -1;
open[0] = 0;
close[len] = 0;
if (str[0] == '(' )
open[1] = 1;
if (str[len - 1] == ')' )
close[len - 1] = 1;
for (let i = 1; i < len; i++)
{
if (str[i] == '(' )
open[i + 1] = open[i] + 1;
else
open[i + 1] = open[i];
}
for (let i = len - 2; i >= 0; i--)
{
if (str[i] == ')' )
close[i] = close[i + 1] + 1;
else
close[i] = close[i + 1];
}
if (open[len] == 0)
return len;
if (close[0] == 0)
return 0;
for (let i = 0; i <= len; i++)
if (open[i] == close[i])
index = i;
return index;
}
let str = "(()))(()()())))" ;
document.write(findIndex(str));
</script>
|
Time Complexity: O(N), where N is the size of the given string
Auxiliary Space: O(N)
Approach 2:
- Count the total number of closed brackets in the string and store in a variable, let’s say cnt_close.
- So count of open brackets is length of (string – count) of closed brackets.
- Traverse string again but now keep count of open brackets in string, let’s say cnt_open.
- Now while traversing, let index be i, so count of closed brackets till that index will be (i+1 – cnt_open).
- Hence, we can check for what index, the count of open brackets in first part equals that of count of closed brackets in second part.
- Equation becomes cnt_close – (i+1 – cnt_open) = cnt_open, we have to find i.
- After evaluating above equation we can see cnt_open gets cancelled on both sides so no need.
Implementation:
C++
#include <bits/stdc++.h>
using namespace std;
int findIndex(string str)
{
int cnt_close = count(str.begin(), str.end(), ')' );
for ( int i = 0; str[i] != '\0' ; i++)
if (cnt_close == i)
return i;
return str.size();
}
int main()
{
string str = "(()))(()()())))" ;
cout << findIndex(str);
return 0;
}
|
Java
public class GFG {
static int findIndex(String str)
{
int len = str.length();
int cnt_close = 0 ;
for ( int i = 0 ; i < len; i++)
if (str.charAt(i) == ')' )
cnt_close++;
for ( int i = 0 ; i < len; i++)
if (cnt_close == i)
return i;
return len;
}
public static void main(String[] args)
{
String str = "(()))(()()())))" ;
System.out.println(findIndex(str));
}
}
|
Python3
def findIndex( str ):
cnt_close = 0
l = len ( str )
for i in range ( 0 , l):
if ( str [i] = = ')' ):
cnt_close = cnt_close + 1
for i in range ( 0 , l):
if (cnt_close = = i):
return i
return l
str = "(()))(()()())))"
print (findIndex( str ))
|
C#
using System;
public class GFG
{
public static int findIndex(String str)
{
var len = str.Length;
var cnt_close = 0;
for ( int i = 0; i < len; i++) {
if (str[i] == ')' ) {
cnt_close++;
}
}
for ( int i = 0; i < len; i++) {
if (cnt_close == i) {
return i;
}
}
return len;
}
public static void Main(String[] args)
{
var str = "(()))(()()())))" ;
Console.WriteLine(findIndex(str));
}
}
|
Javascript
<script>
function findIndex(str)
{
let len = str.length;
int cnt_close = 0;
for (let i = 0; i < len; i++)
if (str[i] == ')' )
cnt_close++;
for (let i = 0; i < len; i++)
if (cnt_close == i)
return i;
return len;
let str = "(()))(()()())))" ;
document.write(findIndex(str));
</script>
|
PHP
<?php
function findIndex( $str )
{
$len = strlen ( $str );
$cnt_close = 0;
for ( $i = 1; $i < $len ; $i ++)
if ( $str [ $i ] == ')' )
$cnt_close ++;
for ( $i = 1; $i < $len ; $i ++)
if ( $cnt_close == $i )
return $i ;
return $len ;
}
$str = "(()))(()()())))" ;
echo (findIndex( $str ));
?>
|
Time Complexity: O(N), Where N is the size of given string
Auxiliary Space: O(1)
Approach 3: (Solution in 1 iteration)
- Count the total number of closed brackets in string and store in variable, let’s say cnt_close.
- So count of open brackets is length of (string – count) of closed brackets.
- Traverse string again but now keep count of open brackets in string, let’s say cnt_open.
- Now while traversing, let index be i, so count of closed brackets till that index will be (i+1 – cnt_open).
- Hence, we can check for what index, the count of open brackets in first part equals that of count of closed brackets in second part.
- Equation becomes cnt_close – (i+1 – cnt_open) = cnt_open, we have to find i.
- After evaluating above equation we can see cnt_open gets cancelled on both sides so no need for the extra loop and simply return cnt_close.
C++
#include<bits/stdc++.h>
using namespace std;
int findIndex(string str){
int cnt_close = 0;
int l = str.length();
for ( int i = 0; i<l; i++){
if (str[i] == ')' )
cnt_close++;
}
return cnt_close;
}
int main(){
string str = "(()))(()()())))" ;
cout<<findIndex(str);
return 0;
}
|
Java
import java.util.*;
public class GFG {
static int findIndex(String str)
{
int cnt_close = 0 ;
int l = str.length();
for ( int i = 0 ; i < l; i++) {
if (str.charAt(i) == ')' )
cnt_close++;
}
return cnt_close;
}
public static void main(String[] args)
{
String str = "(()))(()()())))" ;
System.out.println( "OP: " + findIndex(str));
}
}
|
Python3
def findIndex( str ):
cnt_close = 0
l = len ( str )
for i in range ( 0 , l):
if ( str [i] = = ')' ):
cnt_close = cnt_close + 1
return cnt_close
str = "(()))(()()())))"
print (f "OP: {findIndex(str)}" )
|
C#
using System;
using System.Collections.Generic;
class GFG {
static int findIndex( string str){
int cnt_close = 0;
int l = str.Length;
for ( int i = 0; i < l; i++){
if (str[i] == ')' )
cnt_close++;
}
return cnt_close;
}
public static void Main()
{
string str = "(()))(()()())))" ;
Console.Write( "OP: " +findIndex(str));
}
}
|
Javascript
function findIndex(str){
let cnt_close = 0;
let l = str.length;
for (let i = 0; i<l; i++){
if (str[i] == ')' )
cnt_close++;
}
return cnt_close;
}
let str = "(()))(()()())))" ;
document.write(findIndex(str));
|
Time Complexity: O(N), Where N is the size of the given string
Auxiliary Space: O(1)
Share your thoughts in the comments
Please Login to comment...