File Execution in Pytest
Last Updated :
04 Nov, 2023
In this article, we will explore how to execute test files in Pytest, along with various options and techniques to customize test runs.
What is Pytest?
Pytest is a Python-based testing framework designed for creating and running test codes. While it excels in API testing in the current landscape of REST services, Pytest is versatile enough to handle a wide range of tests, from basic to complex. This includes testing APIs, databases, user interfaces, and more.
Pytest Features
- Simple & Easy: Writing tests with Pytest is straightforward. It uses plain Python code, making it easy for developers to get started.
- Automatic Test Discovery: Pytest can automatically discover and run all the test files in your project. It looks for files named test_*.py or *_test.py and identifies functions named test_* as test cases.
- Availability of Plugins: Pytest has a huge set of plugins that can extend its functionality.
- Parameterized Testing: We can run the same test with different sets of inputs using parameterized testing, which helps in testing various scenarios with minimal code repetition. Also, it will be run on different system environments.
- Report Generating: It can generate coverage reports, showing which parts of your code have been tested and which haven’t.
Install Pytest in Python
We can install Pytest by writing the following command in the command prompt:
pip install pytest
Creating Test Files
First, create a test file which will be tested named ‘my_functions.py’.
- add_numbers(x, y): This function takes two arguments, x and y, and returns their sum.
- subtract_numbers(x, y): This function takes two arguments, x and y, and returns the result of subtracting y from x.
- multiply_numbers(x, y): This function takes two arguments, x and y, and returns their product.
- divide_numbers(x, y): This function takes two arguments, x and y, and performs division. It includes a check to raise a ValueError if y is zero (since division by zero is undefined in mathematics).
Python3
def add_numbers(x, y):
return x + y
def subtract_numbers(x, y):
return x - y
def multiply_numbers(x, y):
return x * y
def divide_numbers(x, y):
if y = = 0 :
raise ValueError( "Cannot divide by zero" )
return x / y
|
Writing a Test Function
Create a another new file named ‘test_my_functions.py’. Pytest automatically identifies files with this naming convention as containing tests.
In test_my_functions.py write a test function using the pytest syntax.
- test_addition(): This test function checks if the add_numbers function in my_functions.py is working correctly. It calls add_numbers(2, 3) and asserts that the result is 5, as expected.
- test_subtraction(): This test function checks if the subtract_numbers function in my_functions.py is working correctly. It calls subtract_numbers(5, 2) and asserts that the result is 3, as expected.
- test_multiplication(): This test function checks if the multiply_numbers function in my_functions.py is working correctly. It calls multiply_numbers(3, 4) and asserts that the result is 12, as expected.
- test_division(): This test function checks if the divide_numbers function in my_functions.py is working correctly. It calls divide_numbers(10, 2) and asserts that the result is 5, as expected. Additionally, it includes an additional test to handle division by zero by attempting to divide by zero and expecting a ValueError to be raised.
- test_addition_negative(): This test function checks if the add_numbers function handles negative numbers correctly. It calls add_numbers(-2, 3) and asserts that the result is 1, as expected.
Python3
import my_functions
def test_addition():
result = my_functions.add_numbers( 2 , 3 )
assert result = = 5 , f "Expected 5, but got {result}"
def test_subtraction():
result = my_functions.subtract_numbers( 5 , 2 )
assert result = = 3 , f "Expected 3, but got {result}"
def test_multiplication():
result = my_functions.multiply_numbers( 3 , 4 )
assert result = = 12 , f "Expected 12, but got {result}"
def test_division():
result = my_functions.divide_numbers( 10 , 2 )
assert result = = 5 , f "Expected 5, but got {result}"
try :
my_functions.divide_numbers( 10 , 0 )
except ValueError as e:
assert str (e) = = "Cannot divide by zero" , f "Expected 'Cannot divide by zero', but got '{e}'"
def test_addition_negative():
result = my_functions.add_numbers( - 2 , 3 )
assert result = = 1 , f "Expected 1, but got {result}"
|
Output
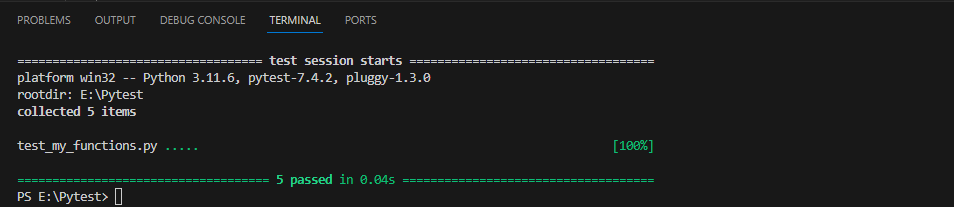
test_my_functions Output
Handling Test Failure Example
When a test fails in Pytest, it means that the expected outcome did not match the actual outcome. Pytest provides clear feedback to identify the cause of the failure. Understanding these techniques will help you efficiently manage and execute your test suites, ensuring the reliability and quality of your Python applications.
Let’s understand, What If a test fails:
- Pytest will display an error message indicating which test failed, along with any relevant information about the failure.
- It will show the exact location in your code where the assertion failed, helping you pinpoint the issue.
- Pytest will also display the values that were expected and the actual values that were obtained.
Example
Create a file named my_functions.py with the following content:
Python3
def add_numbers(x, y):
return x + y
|
Create a file named test_my_functions.py with the following content:
Python3
from my_functions import add_numbers
def test_addition():
result = add_numbers( 2 , 3 )
assert result = = 6
|
Output:
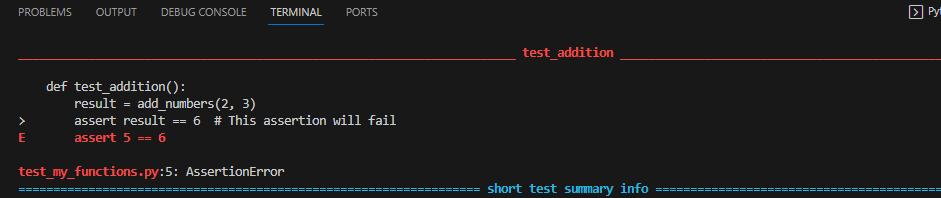
Assertion Error Output
In this example, pytest clearly indicates that the test_addition function failed, and it shows that the assertion assert result == 6 was not met because 5 != 6. This feedback helps you quickly identify what went wrong and where.
Share your thoughts in the comments
Please Login to comment...