Facade Method – C++ Design Patterns
Last Updated :
31 Oct, 2023
The Facade Pattern is a design pattern in software engineering that falls under the structural pattern category. It provides a simplified and unified interface to a set of interfaces or subsystems within a larger system, making it easier to use and reducing the complexity of the system for clients. Essentially, it acts as a facade or entry point to a more complex system, shielding clients from its intricacies.
Important Topics for the Facade Method in C++ Design Patterns
Imagine you have a complex software system with numerous components, each having its own set of methods and interactions. Without the Facade Pattern, clients would need to interact with these components directly, which could lead to a tangled mess of dependencies and make the system difficult to understand and maintain.
Implementation of the Facade Pattern in C++ Design Pattern
Below is the implementation of the Facade Pattern in C++:
C++
#include <iostream>
class Engine {
public :
void Start()
{
std::cout << "Engine started" << std::endl;
}
void Stop()
{
std::cout << "Engine stopped" << std::endl;
}
};
class Lights {
public :
void TurnOn() { std::cout << "Lights on" << std::endl; }
void TurnOff()
{
std::cout << "Lights off" << std::endl;
}
};
class Car {
private :
Engine engine;
Lights lights;
public :
void StartCar()
{
engine.Start();
lights.TurnOn();
std::cout << "Car is ready to drive" << std::endl;
}
void StopCar()
{
lights.TurnOff();
engine.Stop();
std::cout << "Car has stopped" << std::endl;
}
};
int main()
{
Car car;
car.StartCar();
car.StopCar();
return 0;
}
|
Output
Engine started
Lights on
Car is ready to drive
Lights off
Engine stopped
Car has stopped
Explanation of the above code:
- We have two subsystems, Engine and Lights, each with its own methods.
- The Car class acts as the facade. It encapsulates the interactions with the subsystems and provides a simplified interface to the client.
- The Car class’s StartCar and StopCar methods orchestrate the interactions with the subsystems to start and stop the car. Clients don’t need to know the details of how the engine or lights work, they simply call the methods on the Car facade.
This way, the Facade Pattern hides the complexity of the underlying subsystems (engine and lights) and provides a cleaner and more straightforward interface for clients (the Car class). Clients can interact with the Car class without needing to understand the internal workings of the car components, promoting simplicity and maintainability in the code.
Diagram of the Facade Pattern in C++ Design Pattern:
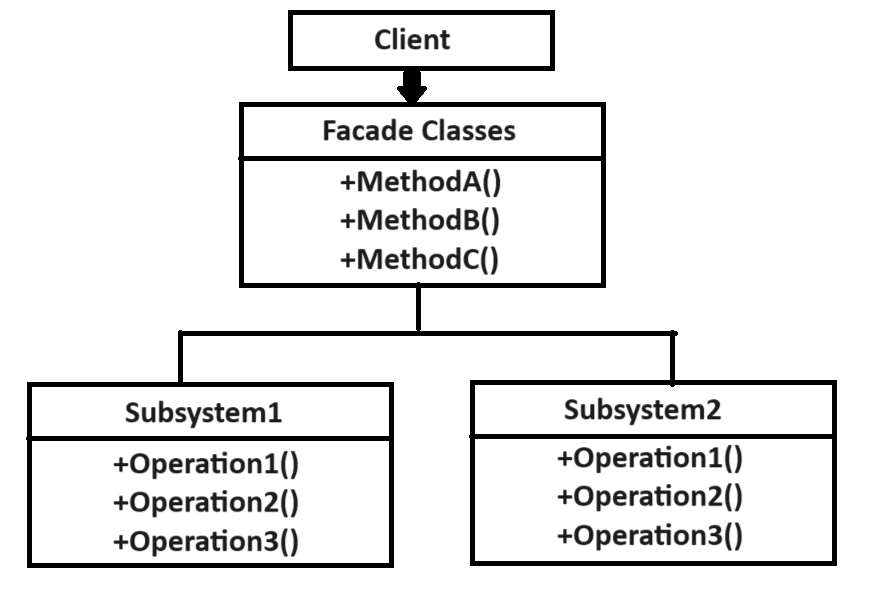
Flow diagram of facade pattern in c++
In this diagram:
- Client represents the class or component that uses the Facade Pattern.
- Facade Class is the class that implements the Facade Pattern. It provides a simplified interface to the client and delegates calls to the underlying subsystems.
- Subsystem 1 and Subsystem 2 are the components or classes that perform specific operations. The client interacts with these subsystems through the facade.
The Facade Class exposes methods (MethodA, MethodB, MethodC) that orchestrate interactions with the subsystems. Clients interact with the Facade Class and are shielded from the complexities of the subsystems.This diagram visually represents how the Facade Pattern simplifies the interaction between the client and a set of subsystems, making it easier for clients to use the system.
Key Benefits of using the Facade Design Pattern in C++ Design Pattern
- Simplified Interface: Clients only need to know about the facade’s methods and don’t need to understand the complexities of the underlying subsystems.
- Reduced Complexity: The pattern hides the intricacies of the subsystems, making the system easier to comprehend and manage.
- Loose Coupling: Clients are decoupled from the subsystems, which allows for more flexible and modular software design.
- Easy Maintenance: Changes to the subsystems can be isolated within the facade, minimizing the impact on client code.
- Improved Testing: Testing becomes easier since clients interact with a single interface rather than multiple subsystems.
Advantages of the Facade Pattern in C++ Design Pattern
Here are the advantages of using the Facade Pattern in C++ ,
- Simplified Interface: The Facade Pattern provides a simple and easy-to-understand interface to a complex system. It hides the intricate details of the subsystems and presents a unified set of methods, making it easier for clients to interact with the system.
- Abstraction of Complexity: It abstracts away the complexity of the underlying subsystems. Clients don’t need to know how various components or classes work together; they can achieve their goals by calling methods on the facade object.
- Reduced Coupling: By introducing a facade, the dependencies between the client code and the subsystems are minimized. This loose coupling enhances maintainability and allows for changes to the subsystems without affecting the client code.
- Enhanced Code Readability: The Facade Pattern improves code readability by providing a clear and concise API. It simplifies client code, making it more understandable and less error-prone.
- Promotes Modular Design: It encourages a modular software design approach. Each subsystem can be developed, tested, and maintained independently. This modularity simplifies development and maintenance efforts.
- Easier Testing: Testing becomes more straightforward because clients can be tested using the facade, which abstracts away the complexities of the subsystems. This isolation simplifies unit testing and reduces the need for complex mocking.
- Encapsulation of Changes: If changes are required in the subsystems (e.g., upgrading a library or refactoring internal code), the facade acts as a buffer. You can make changes within the subsystems while keeping the facade interface stable, minimizing the impact on clients.
- Improved Reusability: Facades can be reused in different parts of the codebase or in different projects, providing a consistent and standardized way to interact with the underlying system.
- Enhanced Debugging: Debugging is more manageable because issues related to the subsystems can be localized within the facade. This isolation simplifies the debugging process and reduces the complexity of finding and fixing bugs.
- Promotes Best Practices: It encourages the use of design principles like separation of concerns and the single responsibility principle. Each subsystem focuses on a specific task, leading to a more organized and maintainable codebase.
Disadvantages of the Facade Pattern in C++ Design Pattern
Here are some of the potential disadvantages or limitations of using the Facade Pattern in C++,
- Limited Flexibility: The Facade Pattern can oversimplify the interface to subsystems, which may limit the flexibility of advanced users who need fine-grained control over the subsystems. Advanced users might find the facade’s interface too restrictive.
- Increased Complexity: In some cases, the facade itself can become complex if it needs to support a wide range of functionalities. This complexity within the facade can be challenging to manage and maintain.
- Maintenance Overhead: While the facade pattern can shield clients from changes in the subsystems, it can also introduce maintenance overhead. If the subsystems change frequently or if there are many subsystems, keeping the facade up to date with those changes can be time-consuming.
- Performance Overhead: The use of a facade might introduce a slight performance overhead due to the additional method calls and abstractions. In performance-critical applications, this overhead could be a concern.
- Possible Violation of Open-Closed Principle: The Facade Pattern may lead to a violation of the Open-Closed Principle if adding new features or subsystems requires modifying the facade class. This can happen if the facade needs to be extended to accommodate changes in the underlying system.
- Duplication of Functionality: If multiple facades are created for different clients or different use cases, there might be duplication of functionality across these facades. This can lead to code redundancy and maintenance challenges.
- Complex Initialization: If the subsystems require complex initialization or configuration, the facade might need to expose this complexity to the client, making the initialization process less straightforward.
- Size and Complexity of the Facade: If the subsystems are very numerous and complex, the facade itself can become large and complex. This can make the facade harder to understand and maintain.
- Tight Coupling with Subsystems: In some cases, the facade may become tightly coupled with the subsystems it encapsulates. This can happen if the facade needs to access internal details of the subsystems to perform its tasks, which defeats the purpose of loose coupling.
- Not Always Appropriate: The Facade Pattern is not suitable for all scenarios. It is most beneficial when dealing with complex systems where simplification of the interface is needed. For simpler systems, adding a facade may introduce unnecessary complexity.
Use Cases of the Facade Pattern in C++ Design Pattern
Here are some common uses of the Facade Pattern in C++:
- Simplified Library/API: When working with a complex external library or API, you can create a facade that abstracts away the intricacies, making it easier for your application to use.
- Subsystem Integration: If your application interacts with multiple subsystems or modules, a facade can provide a unified interface, reducing the complexity of coordinating interactions with these subsystems.
- Legacy Code Integration: When integrating legacy code into a modern system, a facade can act as an adapter, allowing the legacy code to work seamlessly with the new codebase without significant modifications.
- Graphics and GUI Systems: In graphical applications, a facade can simplify interactions with graphic rendering engines, user interface libraries, or input handling systems, providing a straightforward way to create graphics or user interfaces.
- Database Access: When dealing with database operations, a facade can encapsulate the database connection, query execution, and result processing, offering a clean interface to the application.
- Networking: Facades can be used to abstract network communication, handling low-level details such as socket creation, data serialization, and error handling.
- Complex Configuration Management: If your application has a complex configuration system with multiple sources (e.g., files, environment variables, databases), a facade can provide a unified way to access and manage configurations.
- Logging and Debugging: In debugging and logging scenarios, a facade can encapsulate logging operations, making it easier to log messages, errors, and debugging information consistently.
- Security: For security-related tasks such as authentication and authorization, a facade can provide a centralized interface for handling user credentials and permissions, ensuring security practices are consistently applied.
- Resource Management: In systems dealing with resource management (e.g., memory, file handles), a facade can abstract the allocation and deallocation of resources, reducing the risk of resource leaks.
- Multi-platform Development: When developing cross-platform applications, a facade can abstract platform-specific code, allowing the same codebase to work on different platforms with minimal conditional compilation.
- Unit Testing: Facades can aid in unit testing by providing mock implementations of complex subsystems, allowing you to isolate and test specific parts of your code.
- State Management: In applications with complex state management, a facade can simplify state transitions and provide a consistent way to manage and access application states.
- Middleware and Middleware Integration: When integrating middleware systems like message brokers, middleware-specific configurations and interactions can be encapsulated within a facade.
- Application Frameworks: In the development of application frameworks or libraries, a facade can offer a well-defined entry point for users of the framework, shielding them from the framework’s internal complexities.
Share your thoughts in the comments
Please Login to comment...