Proxy Pattern | C++ Design Patterns
Last Updated :
31 Oct, 2023
Design Patterns are an essential part of software engineering, offering proven solutions to common problems encountered during software development. One such pattern is the Proxy Pattern. The Proxy Pattern is a structural design pattern that provides a surrogate or placeholder for another object, allowing you to control access to it. This pattern can be particularly useful in situations where you need to add an extra layer of control, lazy loading, or remote access to objects.
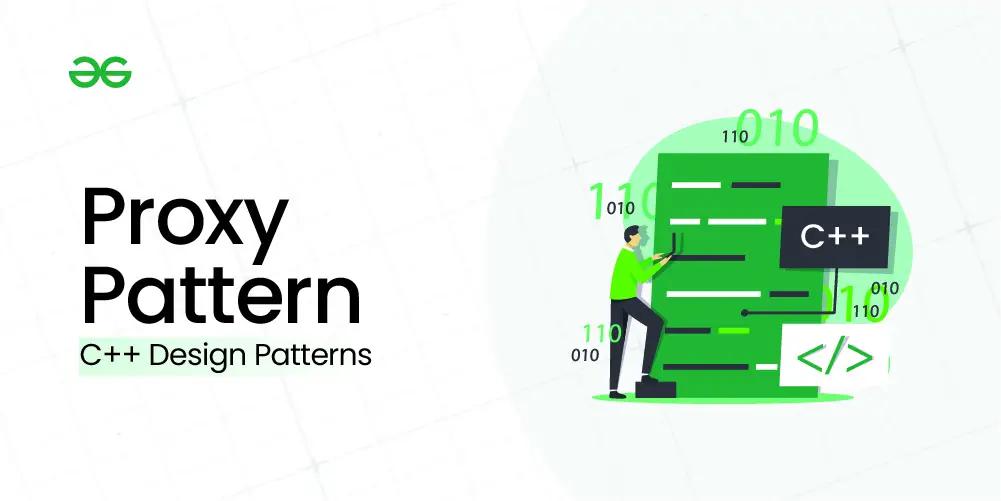
Important Topics for the Proxy Pattern in C++ Design Patterns
What is a Proxy Pattern?
The Proxy Pattern, also known as the Surrogate Pattern that falls under the Gang of Four Design Patterns, is a structural design pattern, meaning it deals with how classes and objects are composed to form larger structures. The pattern involves creating a new class (the proxy) that acts as an intermediary or placeholder for an object (the subject) to control access to it. The proxy can be a local representative of the subject, which helps in various tasks such as lazy initialization, access control, logging, monitoring, or remote communication.
Components of the Proxy Pattern
Imagine you have a friend who’s an expert at solving puzzles, but you don’t want to bother them all the time. You’d like someone to help you access their puzzle-solving skills when needed.
- Subject: Your friend’s ability to solve puzzles is like the Subject. This is the core thing you want to use, which is solving puzzles.
- Real Object: Your friend is the Real Object in this case. They can solve puzzles really well, but it might take some time and effort to engage them every time you need help.
- Proxy: Now let’s introduce a Proxy. In this scenario, the proxy could be your other friend, who acts as an intermediary. When you have a puzzle to solve, you talk to this proxy friend. The proxy friend decides if the puzzle is simple enough to handle themselves. If it’s a tough puzzle, they’ll ask your puzzle-solving expert friend for help.
In simple terms, the Proxy Pattern is like having a middle person (proxy) who decides when to get help from the real expert (real object) and when to handle things themselves. It’s a way to manage and control access to someone’s skills without bothering them unnecessarily.
Implementation of the Proxy Pattern in C++
We’ll create a simplified example where an Image class is used to represent images, and we’ll implement a proxy to control access and display these images.
Step 1: Define the Subject Interface
The Subject is the common interface that both the RealObject and Proxy will implement. In our example, it will be the Image interface:
C++
class Image {
public :
virtual void display() = 0;
};
|
Step 2: Implement the Real Object
The Real Object is the actual class that performs the real work. In our example, we create an RealImage class:
C++
class RealImage : public Image {
private :
std::string filename;
public :
RealImage( const std::string& filename) : filename(filename) {
std::cout << "Loading image: " << filename << std::endl;
}
void display() override {
std::cout << "Displaying image: " << filename << std::endl;
}
};
|
Step 3: Create the Proxy
The Proxy class implements the same interface as the Real Object, and it maintains a reference to the Real Object. The proxy controls access to the Real Object. We’ll call this ImageProxy:
C++
class ImageProxy : public Image {
private :
RealImage* realImage;
std::string filename;
public :
ImageProxy( const std::string& filename) : filename(filename), realImage(nullptr) {}
void display() override {
if (realImage == nullptr) {
realImage = new RealImage(filename);
}
realImage->display();
}
};
|
Step 4: Use the Proxy Pattern
Now, let’s use the Proxy Pattern:
C++
int main() {
Image* image = new ImageProxy("example.jpg");
image->display();
image->display();
delete image;
return 0;
}
|
This code sets up an image proxy, and when you request to display the image, the proxy checks whether the real image needs to be loaded. If it’s already loaded, the proxy just displays it. This demonstrates the lazy-loading aspect of the Proxy Pattern.
The Proxy Pattern allows you to control access to a resource (in this case, an image) and manage when and how the real object is created and accessed without changing the client’s code.
Below is the complete working code of the above example:
C++
#include <bits/stdc++.h>
using namespace std;
class Image {
public :
virtual void display() = 0;
};
class RealImage : public Image {
private :
std::string filename;
public :
RealImage( const std::string& filename) : filename(filename) {
std::cout << "Loading image: " << filename << std::endl;
}
void display() override {
std::cout << "Displaying image: " << filename << std::endl;
}
};
class ImageProxy : public Image {
private :
RealImage* realImage;
std::string filename;
public :
ImageProxy( const std::string& filename) : filename(filename), realImage(nullptr) {}
void display() override {
if (realImage == nullptr) {
realImage = new RealImage(filename);
}
realImage->display();
}
};
int main() {
Image* image = new ImageProxy("example.jpg");
image->display();
image->display();
delete image;
return 0;
}
|
Output
Loading image: example.jpg
Displaying image: example.jpg
Displaying image: example.jpg
Use Cases of the Proxy Pattern
The Proxy Pattern is particularly useful in various scenarios:
- Lazy Loading: When you have a resource-intensive object that should be loaded only when it is needed, a proxy can handle its creation. For instance, loading large images or database connections on-demand.
- Access Control: Proxies can control access to the Real Subject by adding authentication and authorization checks. This is especially useful in securing sensitive resources.
- Caching: Proxies can cache the results of expensive operations, reducing the need to invoke the Real Subject repeatedly.
- Logging and Monitoring: Proxies can be used to log or monitor the actions performed on the Real Subject without modifying its code.
Advantages of the Proxy Pattern
- Control: The Proxy Pattern allows you to control access to the real subject, enabling you to add additional features or security checks without modifying the subject itself.
- Lazy Loading: With virtual proxies, you can delay the creation of resource-intensive objects until they are actually needed, improving performance.
- Remote Access: In distributed systems, the Proxy Pattern allows you to access objects in different address spaces transparently.
- Security: Protection proxies can enforce access control and security checks, preventing unauthorized access to the real subject.
Disadvantages of the Proxy Pattern
- Complexity: Introducing proxy classes can add complexity to the codebase, making it harder to maintain and understand.
- Overhead: In some cases, the use of proxies can introduce additional method calls and indirection, potentially impacting performance.
- Maintenance: Keeping proxies in sync with changes in the real subject can be challenging.
- Misuse: If not used judiciously, the Proxy Pattern can lead to excessive code and an overly complex system.
Conclusion
The Proxy Pattern in C++ is a valuable tool for enhancing the control and efficiency of your applications. It allows you to add an additional layer of functionality to your classes without altering their structure. By using proxies, you can achieve lazy initialization, access control, logging and caching, improving the performance and maintainability of your code. When applied appropriately, the Proxy Pattern can be a powerful asses in your design patterns toolbox.
Share your thoughts in the comments
Please Login to comment...