Facade Method Design Pattern in Java
Last Updated :
20 Feb, 2024
Facade Method Design Pattern is a structural design pattern that provides a simplified interface to a complex subsystem. It acts as a “front door,” concealing the internal complexity of the subsystem and making it easier for clients to interact with it. In this article, we will get to know about what is Facade Method Design Pattern in Java, and why we need Facade Method Design Pattern in Java, with the help of a problem statement and solution
Important Topics for the Facade Method Design Pattern in Java
1. What is the Facade Method Design Pattern in Java?
Facade Design Pattern is a structural design pattern that provides a simplified interface to a set of interfaces in a subsystem, making it easier to use.
.webp)
The functionality of Facade Method Design Patterns in Java are :
- It involves a single class, known as the “facade,” which provides a simplified, higher-level interface to a set of interfaces in a subsystem.
- This simplification helps clients use the subsystem more easily without needing to understand its complexities.
2. Why do we need Facade Method Design Pattern in Java
Example:
A BankingFacade could provide simple methods for account creation, transactions, and balance inquiries, hiding the internal workings of different account types, transaction processing, and security measures.
- Facade Design Pattern is a valuable tool for managing complexity, promoting loose coupling, and enhancing code readability in Java applications.
- It’s particularly useful when dealing with large, interconnected systems or when you want to create a simplified interface for external users.
- Facades can control access to certain parts of a subsystem, enhancing security or enforcing usage patterns.
- It provides a simple, unified interface to a set of interfaces in a subsystem, making it easier to use and understand.
3. Key Component of Facade Method Design Pattern in Java
The key components of the Facade Method Design Pattern in Java:
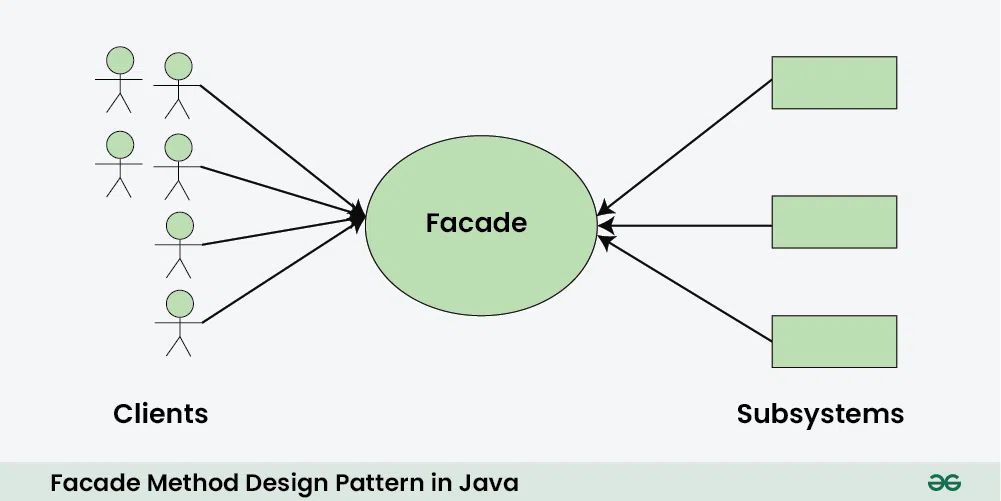
- Subsystem:
- Represents the complex part of the system that the Facade aims to simplify.
- Comprises multiple classes and interfaces that collaborate to provide a set of functionalities.
- Clients typically should not interact with the Subsystem directly, but only through the Facade.
- Facade:
- The heart of the pattern.
- Acts as a simplified, unified interface to the Subsystem.
- Clients interact exclusively with the Facade, not with the underlying Subsystem classes.
- Typically has methods that delegate calls to appropriate classes within the Subsystem, hiding their complexity.
- Client:
- Represents any code that needs to utilize the Subsystem’s functionality.
- Interacts solely with the Facade, unaware of the Subsystem’s internal structure.
- Benefits from the simplified interface provided by the Facade, making code more concise and maintainable.
4. Implementation of Facade Method Design Pattern in Java
Problem Statement:
You are working on a multimedia application that handles various types of media, including audio files, video files, and image files. The application needs to provide a simple and unified interface for playing audio, video, and loading images.
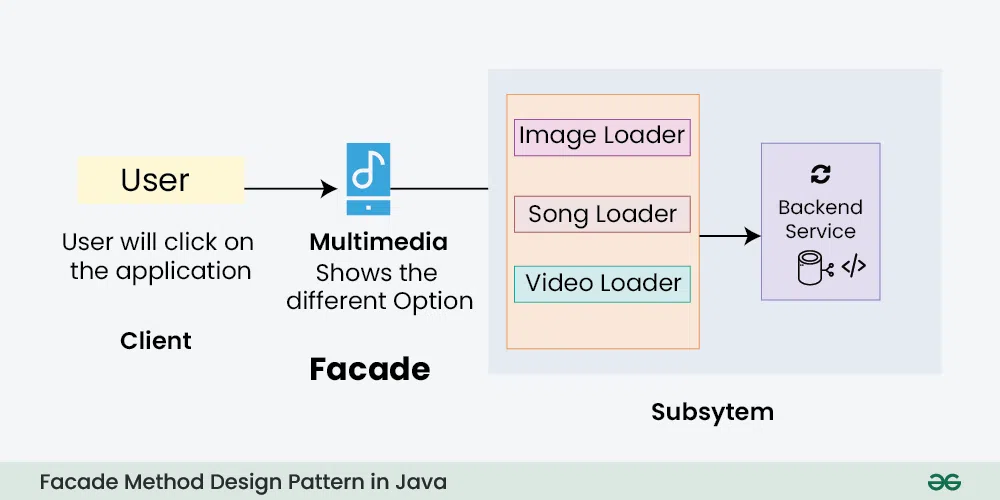
MultimediaFacade
class that acts as a single entry point for interacting with the multimedia subsystem.
- AudioPlayer, VideoPlayer, and ImageLoader, providing a simple
playMedia
method that takes a filename and media type as parameters.
- Client code can then use the facade to play different types of media without worrying about the details of each subsystem component.
Step wise Step implementation of Facade Method Design Pattern
We will see the implementation of Facade Method Design Pattern
4.1 Subsystem Class:
The subsystem Code works as an functionality any class, from the above example we will make the AudioPlayer, VideoPlayer , and ImageLoader as an class. But the user is not able to see what is happening behind the User Interface.
AudioPlayer
class AudioPlayer {
void playAudio(String filename) {
System.out.println("Playing audio file: " + filename);
}
}
|
VideoPlayer
class VideoPlayer {
void playVideo(String filename) {
System.out.println("Playing video file: " + filename);
}
}
|
ImageLoader
class ImageLoader {
void loadImage(String filename) {
System.out.println("Loading image file: " + filename);
}
}
|
4.2 Facade Class:
Here Facade class is Multimedia class. We can take the example of UserInterface here UI is Facade and the code, api server side programming all the thing comes in Sybsystem class.
Java
class MultimediaFacade {
private AudioPlayer audioPlayer;
private VideoPlayer videoPlayer;
private ImageLoader imageLoader;
public MultimediaFacade() {
this .audioPlayer = new AudioPlayer();
this .videoPlayer = new VideoPlayer();
this .imageLoader = new ImageLoader();
}
void playMedia(String filename, String mediaType) {
if (mediaType.equals("audio")) {
audioPlayer.playAudio(filename);
} else if (mediaType.equals("video")) {
videoPlayer.playVideo(filename);
} else if (mediaType.equals("image")) {
imageLoader.loadImage(filename);
} else {
System.out.println("Unsupported media type: " + mediaType);
}
}
}
|
4.3 Client Class:
Java
public class Client {
public static void main(String[] args) {
MultimediaFacade facade = new MultimediaFacade();
facade.playMedia("song.mp3", "audio");
facade.playMedia("movie.mp4", "video");
facade.playMedia("picture.jpg", "image");
facade.playMedia("unknown.file", "unknown");
}
}
|
4.4 Overall Code for the above Implementation:
Java
import java.util.*;
class AudioPlayer {
void playAudio(String filename) {
System.out.println("Playing audio file: " + filename);
}
}
class VideoPlayer {
void playVideo(String filename) {
System.out.println("Playing video file: " + filename);
}
}
class ImageLoader {
void loadImage(String filename) {
System.out.println("Loading image file: " + filename);
}
}
class MultimediaFacade {
private AudioPlayer audioPlayer;
private VideoPlayer videoPlayer;
private ImageLoader imageLoader;
public MultimediaFacade() {
this .audioPlayer = new AudioPlayer();
this .videoPlayer = new VideoPlayer();
this .imageLoader = new ImageLoader();
}
void playMedia(String filename, String mediaType) {
if (mediaType.equals("audio")) {
audioPlayer.playAudio(filename);
} else if (mediaType.equals("video")) {
videoPlayer.playVideo(filename);
} else if (mediaType.equals("image")) {
imageLoader.loadImage(filename);
} else {
System.out.println("Unsupported media type: " + mediaType);
}
}
}
public class Main {
public static void main(String[] args) {
MultimediaFacade facade = new MultimediaFacade();
facade.playMedia("song.mp3", "audio");
facade.playMedia("movie.mp4", "video");
facade.playMedia("picture.jpg", "image");
facade.playMedia("unknown.file", "unknown");
}
}
|
Output
Playing audio file: song.mp3
Playing video file: movie.mp4
Loading image file: picture.jpg
Unsupported media type: unknown
5. Use Cases of Facade Method Design Pattern in Java
- Simplifying Complex Libraries: When working with complex libraries or frameworks, a facade can provide a simplified interface to a subset of functionalities, making it easier for developers to interact with the library without dealing with its intricacies.
- Legacy Code Integration: When integrating new code with existing legacy systems, a facade can act as an interface to the legacy code. This shields the new code from the complexities of the legacy system and provides a cleaner interface.
- Subsystem Abstraction: In applications with multiple subsystems, each handling specific aspects of the overall functionality, a facade can be used to abstract the interactions between these subsystems. This simplifies the usage and understanding of the subsystems.
- Library Wrappers: When using external libraries or APIs, a facade can be implemented to wrap the library’s functionality, providing a simpler and more cohesive interface that aligns with the needs of the application.
- Security Layers: In systems with complex security implementations, a facade can centralize and simplify security-related operations. This ensures that security policies are consistently applied throughout the application.
6. Advantages of Facade Method Design Pattern in Java
- Encapsulation of Complexity:
- The Facade pattern encapsulates the complexities of the subsystem components. It hides the implementation details and presents a high-level interface, promoting a clear separation between the client code and the subsystem.
- Ease of Use:
- Facades make it easier for developers to use a set of related classes or subsystems. Instead of dealing with multiple classes and their interactions, clients can work with a single facade class that abstracts away the complexities.
- Improved Maintainability:
- By providing a single entry point for client code, the Facade pattern makes it easier to maintain and evolve the underlying subsystem. Changes to the subsystem’s implementation can be isolated within the facade, reducing the impact on the client code.
- Flexibility and Decoupling:
- Facades promote loose coupling between subsystem components and clients. Changes to the subsystem, such as adding or removing components, can be made without affecting client code, as long as the facade interface remains unchanged.
- Enhanced Readability:
- The use of a facade enhances the readability of the client code. By replacing a series of complex interactions with a single method call, the code becomes more concise and easier to understand.
- Promotion of Best Practices:
- Facades can enforce best practices and conventions within a subsystem. Clients are guided to use the provided facade methods, ensuring consistent and proper usage of the underlying components.
7. Disadvantages of Facade Method Design Pattern in Java
- The simplicity provided by the facade may come at the cost of flexibility. In some cases, clients may require more fine-grained control over the subsystem components. The facade’s high-level interface might not expose all the functionalities that advanced users need.
- Tight Coupling with Subsystems:
- While the facade pattern aims to promote loose coupling between clients and subsystems, there is a risk of creating tight coupling between the facade and the individual subsystem components. Changes in the subsystem might still impact the facade, especially if the facade closely mirrors the subsystem’s structure.
- Increased Complexity for Maintenance:
- In certain situations, a facade might become an additional layer that needs to be maintained. If the facade is not properly designed, it can add complexity to the codebase instead of simplifying it. Changes in the subsystem might necessitate modifications to both the facade and client code.
- Performance Overhead:
- The use of a facade can introduce a slight performance overhead, especially if the facade has to delegate multiple calls to different subsystem components. While the impact is often negligible, it’s something to consider in performance-critical applications.
8. Conclusion
The facade pattern is appropriate when you have a complex system that you want to expose to clients in a simplified way, or you want to make an external communication layer over an existing system that is incompatible with the system. Facade deals with interfaces, not implementation. Its purpose is to hide internal complexity behind a single interface that appears simple on the outside.
Share your thoughts in the comments
Please Login to comment...