Flyweight Pattern | C++ Design Patterns
Last Updated :
31 Oct, 2023
A flyweight pattern is a structural design pattern used to optimize memory usage and performance when dealing with a large number of objects that share some common characteristics. It achieves this by separating an object’s intrinsic state (shared among multiple objects) from its extrinsic state (unique to each object) and storing the intrinsic state externally, typically within a Flyweight factory. This pattern is particularly useful when you need to create a significant number of similar objects and want to minimize the memory footprint.
Important Topics for the Flyweight Pattern in C++ Design Patterns
Problem Statement
In many applications, you may need to create a large number of objects that have some common properties or characteristics. Without optimization, this can lead to excessive memory consumption because each object contains duplicated data for the common properties. Additionally, it may impact performance due to the overhead of creating and managing numerous objects.
Solution
The Flyweight pattern suggests separating the intrinsic state (shared among multiple objects) from the extrinsic state (varies between objects). The intrinsic state is stored externally, typically within a Flyweight factory, and the extrinsic state is provided by the client code when needed.
Key Components
- Flyweight Interface or Base Class: This defines the methods for accessing and manipulating the intrinsic state.
- Concrete Flyweight: Implementations of the Flyweight interface that store and manage the intrinsic state. They are typically lightweight and capable of being shared.
- Flyweight Factory: A factory class responsible for creating and managing flyweight objects. It ensures that flyweights are shared and reused when possible.
Use Cases
- Text processing, where individual characters can be represented as flyweights, and the text document contains references to these characters.
- Graphic design applications, where graphical elements like fonts, icons, or colors can be represented as flyweights.
- Game development, where objects like trees, rocks, or bullets can be managed as flyweights to reduce memory consumption.
Example
Consider a text editor where each character in the document is represented as an object. If you have a large document, creating individual objects for each character can be memory-intensive. However, most characters share common properties like the font and size, which can be optimized to reduce memory usage.
Below is the implementation of a simplified example using characters in a text editor:
C++
#include <iostream>
#include <unordered_map>
class Character {
public :
Character( char intrinsicState) : m_intrinsicState(intrinsicState) {}
void draw( int extrinsicState) {
std::cout << "Drawing character '" << m_intrinsicState << "' at position " << extrinsicState << std::endl;
}
private :
char m_intrinsicState;
};
class CharacterFactory {
public :
Character* getCharacter( char key) {
if (m_characters.find(key) == m_characters.end()) {
m_characters[key] = new Character(key);
}
return m_characters[key];
}
private :
std::unordered_map< char , Character*> m_characters;
};
int main() {
CharacterFactory characterFactory;
int position = 0;
characterFactory.getCharacter( 'A' )->draw(position++);
characterFactory.getCharacter( 'B' )->draw(position++);
characterFactory.getCharacter( 'C' )->draw(position++);
return 0;
}
|
Output:
Drawing character ‘A’ at position 0
Drawing character ‘B’ at position 1
Drawing character ‘C’ at position 2
In this example, we have a Character class that represents a flyweight object.
- The Character class has an intrinsic state, which is the character itself.
- An extrinsic state, which is the position where the character is drawn.
The CharacterFactory class acts as a flyweight factory and stores references to the flyweight objects. When a client requests a character, the factory checks if it already exists in the factory’s storage. If it does, it returns the existing object; otherwise, it creates a new object and adds it to the storage.
In the main() function, we demonstrate how to use the flyweight objects. We create three characters (‘A’, ‘B’, ‘C’) at different positions and draw them on the screen.
Diagram Explaining the Flyweight Pattern
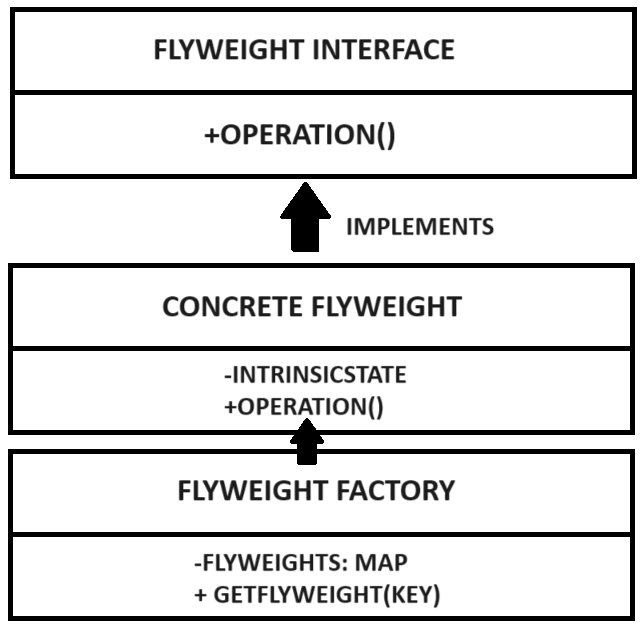
Flow diagram of flyweight pattern
In this diagram:
- Flyweight Interface defines the methods for accessing and manipulating the intrinsic state. This interface is typically implemented by concrete flyweights.
- Concrete Flyweight represents the shared intrinsic state. It contains a private member (intrinsicState) for storing this shared data. The operation method may use this intrinsic state when necessary.
- Flyweight Factory is responsible for creating and managing flyweight objects. It maintains a collection (e.g., a map) of flyweights, where each flyweight is associated with a unique key. The getFlyweight method ensures that flyweights are shared and reused based on the provided key.
In practice, the Flyweight Factory is responsible for managing the sharing of flyweight objects, ensuring that multiple objects with the same intrinsic state are represented by a single shared instance. This helps minimize memory usage and improve performance, especially when dealing with a large number of similar objects.
Advantages of Flyweight Pattern in C++ Design Patterns
Here are the advantages of the Flyweight pattern in C++:
- Memory Efficiency: The primary advantage of the Flyweight pattern is its ability to significantly reduce memory consumption. By sharing common intrinsic state among multiple objects, it avoids storing redundant data, which is especially valuable when dealing with a large number of similar objects.
- Performance Improvement: Reduced memory usage often leads to improved performance. Fewer memory allocations and deallocations can result in faster object creation and less overhead, making the application more efficient.
- Reuse of Objects: Flyweight objects are designed to be reused. Instead of creating a new object for every instance, the pattern encourages the reuse of existing objects with the same intrinsic state. This promotes efficient memory and resource management.
- Scalability: The Flyweight pattern is well-suited for applications that need to scale efficiently, especially in situations where a large number of objects are required. It helps keep memory usage predictable and manageable as the application grows.
- Simplified Design: By separating intrinsic state from extrinsic state and using shared flyweights, the design of the application becomes simpler and more modular. It promotes clean separation of concerns and maintains a clear distinction between shared and unique data.
- Reduced Object Overhead: Creating and managing a large number of objects can introduce overhead in terms of memory and CPU usage. By sharing common state, the pattern reduces this overhead, leading to a more streamlined and responsive application.
- Improved Maintenance: Flyweight objects are typically lightweight and self-contained. This makes the codebase easier to maintain and understand, as you have fewer classes and objects to manage, especially when dealing with a multitude of similar entities.
- Consistency: Since shared intrinsic state is centralized within the flyweight objects, it ensures consistency in the application. Changes to the intrinsic state are reflected in all objects that share that state, promoting data integrity.
- Resource Management: In resource-intensive applications (e.g., graphics rendering or gaming), the Flyweight pattern can help manage resources efficiently, such as textures, fonts, or meshes, by sharing them among multiple instances.
- Customization: While intrinsic state is shared, extrinsic state can still be customized for each object. This allows you to have individuality and uniqueness where needed while optimizing memory usage for shared properties.
Disadvantages of Flyweight Pattern in C++ Design Patterns
Here are some potential disadvantages of using the Flyweight pattern in C++,
- Complexity: Implementing the Flyweight pattern can introduce additional complexity to your codebase, especially when managing the separation of intrinsic and extrinsic states. This complexity may make the code harder to understand for developers who are not familiar with the pattern.
- Increased Codebase: The pattern may require the creation of multiple classes and interfaces, including the Flyweight interface, Concrete Flyweight, and Flyweight Factory. This can lead to a larger codebase, which might be overkill for simpler applications.
- Performance Overhead: In some cases, the Flyweight Factory’s lookup and creation logic can introduce a small performance overhead. While this is typically negligible, it can be a concern in performance-critical applications.
- Maintaining Extrinsic State: Managing the extrinsic state (unique state) of flyweight objects can be tricky. Developers need to ensure that the extrinsic state is properly assigned to the flyweight instances, which can be error-prone.
- Limited Applicability: The Flyweight pattern is most beneficial when there is a significant amount of shared intrinsic state. In situations where the objects have little to no shared state, implementing the pattern may not provide noticeable benefits and could add unnecessary complexity.
- Thread Safety: Ensuring thread safety when working with shared flyweights can be challenging, especially in multi-threaded applications. Developers need to carefully consider synchronization mechanisms to prevent race conditions.
- Inflexibility: The Flyweight pattern may lead to inflexibility in certain cases. For example, if you need to modify the intrinsic state of a specific instance without affecting others, the pattern might not be suitable.
- Complex Initialization: Depending on the complexity of the intrinsic state and how it’s shared, initializing flyweight objects and maintaining their state can be intricate, potentially leading to initialization-related issues.
- Debugging: Debugging applications that use the Flyweight pattern can be more challenging, as multiple objects might share the same intrinsic state. Identifying which instance is causing a particular issue may require additional effort.
- Overhead for Small Collections: In scenarios where you have a small collection of objects or where most objects have unique intrinsic states, the overhead introduced by implementing the Flyweight pattern may not be justified.
Uses of Flyweight Pattern
Here are some common uses of the Flyweight pattern in C++,
- Text Editors: In a text editor application, individual characters can be represented as flyweights. Each character has intrinsic properties like font, size, and style, which can be shared among multiple occurrences.
- Graphics Rendering: In graphics rendering engines, objects such as fonts, textures, and geometric shapes often have shared properties. The Flyweight pattern can be used to efficiently manage and render these objects, reducing memory usage.
- Game Development: In video games, elements like game sprites, textures, and particle effects can be represented as flyweights. This helps optimize memory and rendering performance, especially in scenes with many similar game objects.
- Geographical Information Systems (GIS): GIS applications often deal with large datasets of geographical features. The Flyweight pattern can be applied to represent common attributes shared among map features like rivers, roads, or landmarks.
- Virtual Worlds: In virtual worlds or simulations, objects like trees, rocks, or buildings can be flyweights. These objects often share common characteristics like textures, geometry, and behavior.
- Financial Software: In financial software, financial instruments like stocks, bonds, or currencies can be implemented as flyweights to optimize memory usage while tracking financial data.
- Weather Data Visualization: Visualization applications for weather data can use the Flyweight pattern to efficiently manage shared attributes like temperature, humidity, and wind speed across different locations.
Share your thoughts in the comments
Please Login to comment...