Exploring Lazy Loading Techniques for Django Templates
Last Updated :
06 Dec, 2023
In this article, we’ll explore how to implement lazy loading in Django templates using JavaScript and HTML. If you have no idea about how to create projects in Django please refer to this Getting Started with Django.
What is the Lazy Loading Technique?
Lazy loading is a performance optimization technique that can be beneficial in web development. It’s a way to improve the loading speed of web applications, which is important everywhere. By deferring the loading of certain assets until they are actually needed, you can significantly enhance the user experience and reduce the initial page load time. Lazy loading can help reduce initial page load times, especially in areas with slower internet connections. Lazy loading can be used in Django templates to enhance the rendering of big or complicated templates that contain weighty elements like photos, videos, and audio files.
Implementing Lazy Loading Techniques for Django Templates
Here we will see the steps for creating a Movie Ticket Booking Website using Django. Let’s start creating a project Getting Started we lazy load the image by using Javascript. To install Django follow these steps.
Starting the Project Folder
To start the project use this command
django-admin startproject LazyLoadDemo
cd core
To start the app use this command
python manage.py startapp myapp
In your project’s ‘settings.py’, add your app to INSTALLED_APPS:
Python3
INSTALLED_APPS = [
'myapp' ,
]
|
File structure
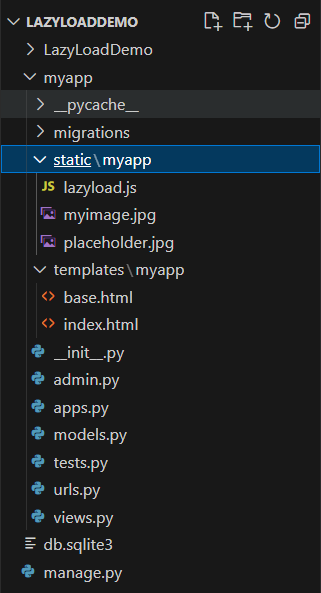
Setting up the files
views.py: The code is a Django view function named ‘index'
. When a user accesses a specific URL, it renders the ‘myapp/index.html’ template and returns it as an HTML response, displaying the content of the template. This is a common way to create web pages in a Django application.
Python3
from django.shortcuts import render
def index(request):
return render(request, 'myapp/index.html' )
|
Creating GUI
index.html: This is the index file in HTML which is used to present the homepage and load image by using the Lazy loading technique.
HTML
{% extends "myapp/base.html" %}
{% block content %}
< h1 >Welcome to My Lazy Loading Example</ h1 >
< img data-src = "/static/myapp/myimage.jpg" alt = "My Image" class = "lazy" >
{% endblock %}
|
base.html: Here we create a base HTML template that includes the JavaScript file.
HTML
{% load static %}
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< title >Lazy Load Demo</ title >
</ head >
< body >
{% block content %}{% endblock %}
< script src = "{% static 'myapp/lazyload.js' %}" ></ script >
</ body >
</ html >
|
Setting Up Static Files
Create the JavaScript file for lazy loading, lazyload.js, in the static/myapp/ directory:
Javascript
document.addEventListener( "DOMContentLoaded" , function () {
const lazyImages = document.querySelectorAll( ".lazy" );
const lazyLoad = function () {
lazyImages.forEach( function (img) {
if (img.getBoundingClientRect().top <= window.innerHeight && img.getBoundingClientRect().bottom >= 0 && getComputedStyle(img).display !== "none" ) {
img.src = img.dataset.src;
img.classList.remove( "lazy" );
}
});
};
lazyLoad();
document.addEventListener( "scroll" , lazyLoad);
window.addEventListener( "resize" , lazyLoad);
window.addEventListener( "orientationchange" , lazyLoad);
});
|
myapp/urls.py
In this URL file we are defining that which view need to be called when a particular URL is entered.
Python3
from django.urls import path
from . import views
app_name = 'myapp'
urlpatterns = [
path(' ', views.index, name=' index'),
]
|
urls.py
Here in this main URL file we are including the app urls.
Python3
from django.contrib import admin
from django.urls import include, path
urlpatterns = [
path( 'admin/' , admin.site.urls),
path( 'myapp/' , include( 'myapp.urls' )),
]
|
Deployement of the Project
Run the server with the help of following command:
python3 manage.py runserver
Output:
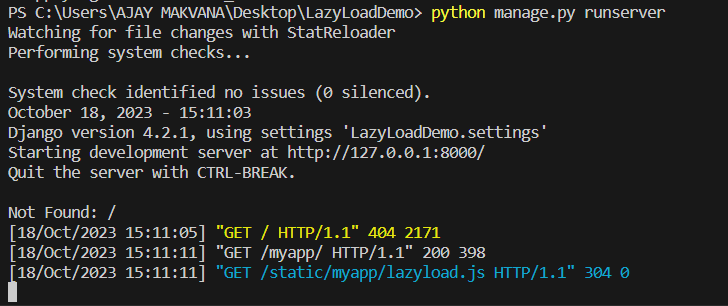
VS code terminal output
Browser Output:
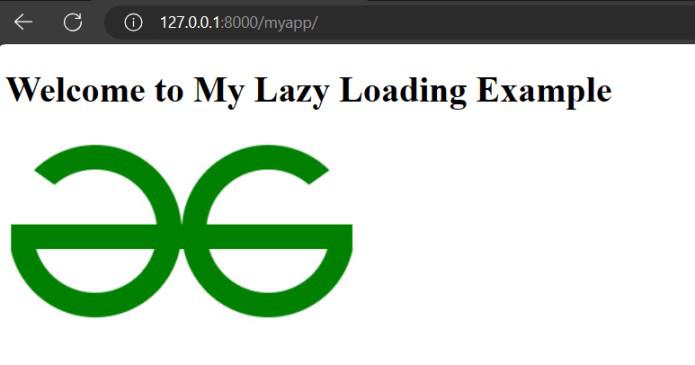
Browser Output
FAQs related to lazy loading
1. What are the performance benefits of lazy loading?
In Area, where internet speeds can vary widely, lazy loading helps to improve website loading time and performance by reducing the initial loading time, and make sites more accessible to end users with limited internet bandwidth.
2. What is lazy loading in Django templates, and why is it important?
Lazy loading in Django templates is a technique where content (like images, video or audio files) is loaded only when it’s needed, which can reduce the initial page load time.
3. Can I apply lazy loading to other types of content, not just images?
Yes, lazy loading can be applied to diff – diff types of content, including text, videos, and other assets. The main concept is to use JavaScript to load the content as needed when it comes into the user’s viewport.
Share your thoughts in the comments
Please Login to comment...