Explain the role of the root injector in Angular.
Last Updated :
19 Apr, 2024
Angular’s dependency injection enables modular and reusable components across applications. At the core of dependency injection lies the root injector, a powerful mechanism responsible for managing dependencies and providing instances of services, components, directives, and more throughout the application. In this article, we’ll see more about the role and significance of the root injector in Angular, exploring its functionalities, responsibilities, etc.
Prerequisites
What is Root Injection?
In Angular, the root injector serves as the primary entry point for the dependency injection system, acting as a centralized hub that is responsible for creating and managing instances of injectable entities such as services, components, and directives throughout the application. It sits at the top of the hierarchical injection system, that serves as the primary provider of dependencies for the entire application.
Features of Root Injection
The root injector offers several key features:
- Singleton Instances: The root injector ensures that each service has only one instance shared throughout the entire application, acting as the keeper of single copies.
- Hierarchical Injectors: The root injector is part of a hierarchical structure, where each component has its own injector that inherits from its parent. This allows services to have different instances depending on their usage within the application.
- Provider Registration: The root injector acts as a registrar for services, keeping track of how services should be created and provided to different parts of the application.
- Dependency Resolution: When a component or service requires another service, the root injector resolves the dependency by identifying the required service and providing its instance.
Key Roles and Responsibilities
- Global Singleton Scope: The root injector creates instances of services with a singleton scope by default, ensuring that there’s only one instance of each service throughout the application.
- Lazy Loading Support: The root injector supports lazy loading in Angular applications. When a module is lazily loaded, Angular creates a new injector for that module, which inherits from the root injector.
- Platform Initialization: The root injector initializes the Angular platform and bootstraps the application. It creates the initial set of components and services required to start the application and manages the application lifecycle.
- Providing Global Services: Services provided at the root level are considered global services, available throughout the application. The root injector manages the instantiation and lifecycle of these global services.
- Configuration and Providers: The root injector is configured with providers, which define how dependencies are resolved and expressed.
Steps to implement root injector in Angular
Here’s an example of how to create an Angular application and use the root injector to provide a service:
Step 1: Generate a new Angular application using the Angular CLI
ng new my-app
Step 2: Generate a service using the Angular CLI:
ng generate service my-service
Folder Structure:
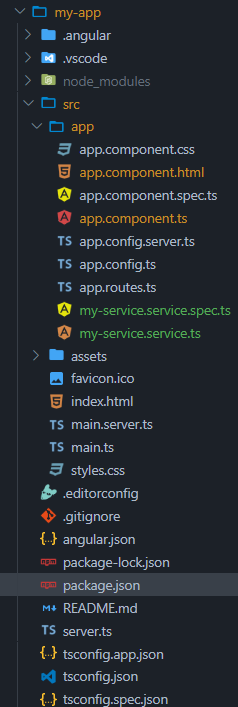
The updated dependencies in the package.json file
"dependencies": {
"@angular/animations": "^17.2.0",
"@angular/common": "^17.2.0",
"@angular/compiler": "^17.2.0",
"@angular/core": "^17.2.0",
"@angular/forms": "^17.2.0",
"@angular/platform-browser": "^17.2.0",
"@angular/platform-browser-dynamic": "^17.2.0",
"@angular/platform-server": "^17.2.0",
"@angular/router": "^17.2.0",
"@angular/ssr": "^17.2.3",
"express": "^4.18.2",
"rxjs": "~7.8.0",
"tslib": "^2.3.0",
"zone.js": "~0.14.3"
}
Example: Add the following codes in the required files.
JavaScript
//my-service.service.ts
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root'
})
export class MyServiceService {
getMessage() {
return 'Hello from MyService!';
}
}
JavaScript
//app.component.ts
import { Component } from '@angular/core';
import { MyServiceService } from './my-service.service';
@Component({
selector: 'app-root',
template: `
<div>
<h1>{{ message }}</h1>
</div>
`
})
export class AppComponent {
message: string;
constructor(private myService: MyServiceService) {
this.message = this.myService.getMessage();
}
}
Step 3: Run the application using the Angular CLI:
ng serve --open
Output: When you open the application in your browser, you should see the message “Hello from MyService!” displayed on the page. Something like this.
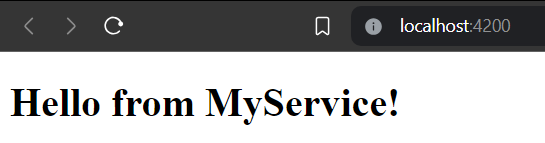
In this example, the MyServiceService is registered as a singleton service in the root injector of the Angular application by utilizing the providedIn: ‘root’ metadata. This configuration ensures that a single instance of MyServiceService is created and shared across the entire application hierarchy, promoting efficient resource utilization and consistent behavior. The root injector acts as the central authority, creating and managing this shared instance during the application’s bootstrap process, allowing any component, directive, or service to access and interact with the same instance of MyServiceService.
Share your thoughts in the comments
Please Login to comment...