Exit From App on Double Click of Back Button in Android using Kotlin
Last Updated :
06 Dec, 2021
The ‘Back‘ button has many different uses in many different android apps. While some app developers use it to close their apps, some use it to traverse back to the app’s previous activity. Many apps require the user to press the ‘Back’ button two times within an interval to successfully close the application, which is considered the best practice. So it is a good practice to exit from an app with a double click of the back button. As some time user can press the back button by mistake. So, to ensure that, whether the user really wants to exit from the app we implement this feature.
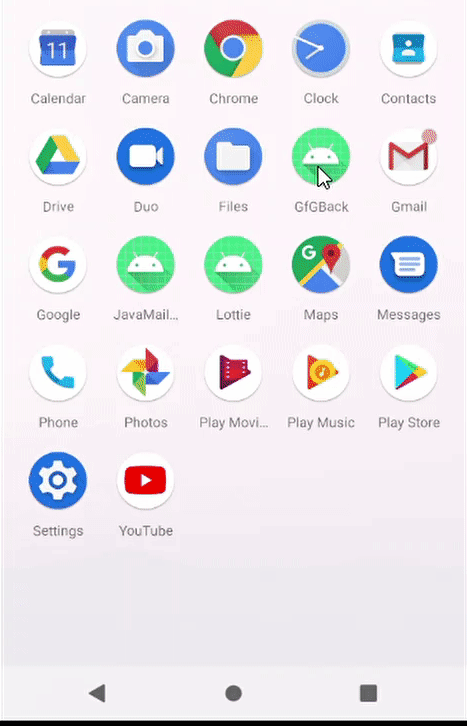
So in this article, we will learn How to implement an exit feature with a double click of the back button in our app. We will implement this feature in our app using kotlin.
Note: To implement in Java refer to this article: How to Implement Press Back Again to Exit in Android?
Step by step Implementation
Step 1: Create a new android project in kotlin.
Step 2: XML layout for activity, In this project we only have single activity. And the XML layout design will also be very simple.
XML
<? xml version = "1.0" encoding = "utf-8" ?>
< androidx.constraintlayout.widget.ConstraintLayout
android:layout_width = "match_parent"
android:layout_height = "match_parent"
tools:context = ".MainActivity" >
< ImageView
android:layout_width = "80dp"
android:layout_height = "80dp"
android:src = "@drawable/ic_geeksforgeeks"
app:layout_constraintBottom_toTopOf = "@+id/textView"
app:layout_constraintEnd_toEndOf = "parent"
app:layout_constraintStart_toStartOf = "parent"
app:layout_constraintTop_toTopOf = "parent" />
< TextView
android:id = "@+id/textView"
android:layout_width = "wrap_content"
android:layout_height = "wrap_content"
android:text = "Welcome to GeeksForGeeks"
android:textColor = "#7CB342"
android:textSize = "20dp"
android:textStyle = "bold"
app:layout_constraintBottom_toBottomOf = "parent"
app:layout_constraintLeft_toLeftOf = "parent"
app:layout_constraintRight_toRightOf = "parent"
app:layout_constraintTop_toTopOf = "parent"
app:layout_constraintVertical_bias = "0.303" />
</ androidx.constraintlayout.widget.ConstraintLayout >
|
You can customize the layout.
Step 3: Now in mainActivity, We will override onBackPressed() method. With the first press of the back button, we will store the current system time, and display a toast. If the user presses the back button again within 3 seconds we will call the finish() method.
Kotlin
package com.ayush.gfg_exit
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.widget.Toast
import kotlin.properties.Delegates
class MainActivity : AppCompatActivity() {
var backPressedTime: Long = 0
override fun onCreate(savedInstanceState: Bundle?) {
super .onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
}
override fun onBackPressed() {
if (backPressedTime + 3000 > System.currentTimeMillis()) {
super .onBackPressed()
finish()
} else {
Toast.makeText( this , "Press back again to leave the app." , Toast.LENGTH_LONG).show()
}
backPressedTime = System.currentTimeMillis()
}
}
|
So our app is ready.
Output:
Share your thoughts in the comments
Please Login to comment...