How to Implement Press Back Again to Exit in Android?
Last Updated :
01 Sep, 2020
The ‘Back‘ button has many different uses in many different android apps. While some app developers use it to close their apps, some use it to traverse back to the app’s previous activity. Many apps require the user to press the ‘Back’ button two times within an interval to successfully close the application, which is considered the best practice.
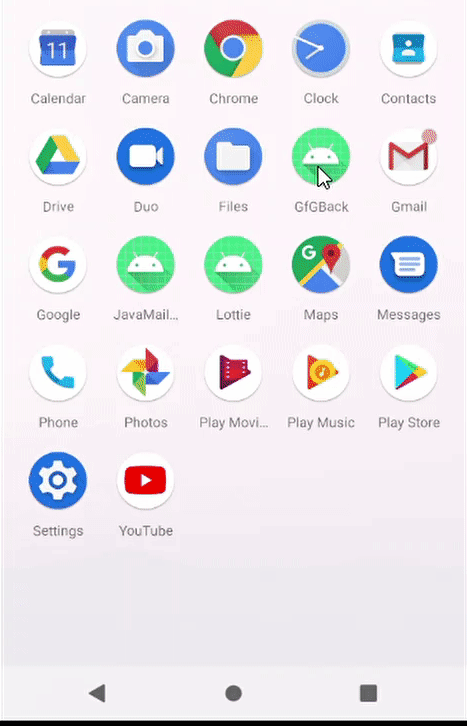
Why implement this in the app?
- It adds a better UX to the app providing the user with a satisfying experience.
- It acts as a confirmation in case the user presses the ‘Back’ button by mistake.
Approach
Step 1: Create a new Android Studio project
Please refer to this article How to create a new project in Android Studio to see in detail how to create a new Android Studio project. Note that choose Java as the programming language.
Step 2: Working with activity_main.xml file
In this example, we will have only a single layout, activity_main.xml which will contain an ImageView and a TextView. This is how our activity_main.xml looks like:
activity_main.xml
<? xml version = "1.0" encoding = "utf-8" ?>
< LinearLayout
android:layout_width = "match_parent"
android:layout_height = "match_parent"
tools:context = ".MainActivity"
android:background = "#388e3c"
android:orientation = "vertical" >
< ImageView
android:layout_width = "wrap_content"
android:layout_height = "wrap_content"
android:src = "@drawable/gfglog" />
< TextView
android:padding = "15dp"
android:layout_width = "wrap_content"
android:layout_height = "wrap_content"
android:text = "This was written at GeeksforGeeks"
android:textColor = "#f5f5f5"
android:textSize = "30sp"
android:gravity = "center_horizontal" />
</ LinearLayout >
|
The above layout looks like this:
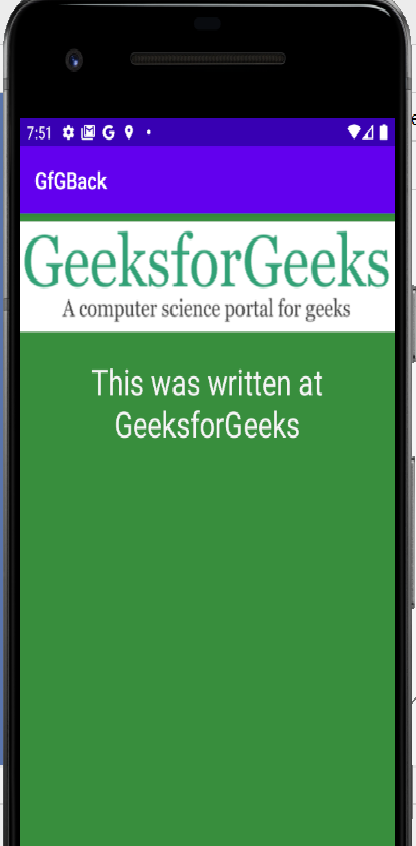
Layout Output
Step 3: Working with MainActivity.java file
Now comes the main part of the app. In order to check when the ‘BACK’ button is pressed, use onBackPressed() method from the Android library. Next, perform a check to see if the ‘BACK’ button is pressed again within 2 seconds and will close the app if it is so. Otherwise, don’t exit. Here’s how the MainActivity.java looks like:
MainActivity.java
package org.geeksforgeeks.pressbackexit;
import android.os.Bundle;
import android.widget.Toast;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
private long pressedTime;
@Override
protected void onCreate(Bundle savedInstanceState) {
super .onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
@Override
public void onBackPressed() {
if (pressedTime + 2000 > System.currentTimeMillis()) {
super .onBackPressed();
finish();
} else {
Toast.makeText(getBaseContext(), "Press back again to exit" , Toast.LENGTH_SHORT).show();
}
pressedTime = System.currentTimeMillis();
}
}
|
In the above code, when the user presses the ‘BACK’ button once, they are greeted with a toast asking them to press it again to exit. If the user then presses ‘BACK’ again within 2 seconds(2000ms), then the app is closed, otherwise, we remain there.
Output: Run on Emulator
Share your thoughts in the comments
Please Login to comment...