Error Handling in Objective-C
Last Updated :
09 Feb, 2024
In this article, we will learn about error handling in objective C. There are different methods to handle errors in Objective-C with the help of examples and code.
What is Error Handling?
Error handling is defined as the process or mechanism in programming languages for identifying, catching, and responding to exceptional situations that may occur during the execution of the program. So basically, in simple words, it is the process of identifying the errors in the program and throwing them out without affecting the program’s output.
- There can be various types of errors or they arise due to various reasons like hardware failure, programming mistakes, incorrect input, etc, so error handling is used to identify and neglect these types of errors.
- Proper error handling is very essential for creating robust and reliable software.
- In Objective-C error handling is used to write robust codes, here error handling is mostly implemented by using the Cocoa error-handling pattern or technique to identify the errors in the written code.
- This method completely relies on the use of NSError objects in the program.
- Exception handling is another method used for error handling there we use it to try and catch the block to identify the error in the program and then throw the error out of the program without affecting the code.
What is Exception Handling?
NSError is the most preferred way to handle the errors in Objective-C, but we can also use exception handling for exceptional cases. In this, we will use try and catch block for identifying the errors and throwing the errors out of the program without impacting the code. However, exceptions should be used sparingly for exceptional circumstances and not for routine error handling.
Syntax:
@try {
// Code that may raise an exception
}
@catch (NSException *exception) {
NSLog(@”An exception occurred: %@”, [exception reason]);
}
@finally {
// Code that will be executed regardless of whether an exception occurred
}
In this method these two blocks try and catch are used for identifying the errors in the program.
NSError for Error Handling
This is the most used method or technique for identifying the errors in Objective-C. NSError class is used for detecting errors in the program. It allows us to pass the errors to the calling code through an out-parameter.
Syntax:
NSError *error = nil;
NSData *data = [NSData dataWithContentsOfFile:@”example.txt” options:NSDataReadingUncached error:&error];
if (error != nil) {
NSLog(@”Error reading file: %@”, [error localizedDescription]);
} else {
// Process data
}
This approach allows developers to gracefully handle errors and communicate detailed information about the failure when interacting with APIs or performing operations that may result in unexpected outcomes.
Examples of Error Handling in Objective-C
In Objective-C the main method used to handle the errors is NSError let’s see some examples to understand how this method works.
Example 1:
Let’s see the example where the NSError method is used to find out the error in the program, if there is no error in the program then we get the exact output that we want but if there is any error in the program then the NSError method comes into play.
ObjectiveC
#import <Foundation/Foundation.h>
BOOL performTaskWithError( NSError **error) {
if (error != NULL ) {
NSDictionary *userInfo = @{
NSLocalizedDescriptionKey : @"Task failed" ,
NSLocalizedFailureReasonErrorKey : @"The task encountered an error" ,
};
*error = [ NSError errorWithDomain: @"com.example.error" code:1 userInfo:userInfo];
return NO ;
}
return YES ;
}
int main( int argc, const char * argv[]) {
@autoreleasepool {
NSError *error = nil ;
if (!performTaskWithError(&error)) {
NSLog ( @"Error: %@" , error.localizedDescription);
NSLog ( @"Failure Reason: %@" , error.localizedFailureReason);
} else {
NSLog ( @"Task completed successfully" );
}
}
return 0;
}
|
Output:

Output
Explanation:
In this program, there are some programming errors so by the help of the NSError method we get the error in the result that the Task failed due to an error. if there is no error then the desired output will be printed.
Example 2:
In this example, we are using the NSError method in the calculator program and when there is any condition like divide by zero then the NSError method throws that error.
ObjectiveC
#import <Foundation/Foundation.h>
@interface Calculator : NSObject
- ( NSInteger )divide:( NSInteger )numerator by:( NSInteger )denominator error:( NSError **)error;
@end
@implementation Calculator
- ( NSInteger )divide:( NSInteger )numerator by:( NSInteger )denominator error:( NSError **)error {
if (denominator == 0) {
if (error != NULL ) {
NSString *domain = @"CalculatorErrorDomain" ;
NSInteger code = 1;
NSDictionary *userInfo = @{ NSLocalizedDescriptionKey : @"Cannot divide by zero." };
*error = [ NSError errorWithDomain:domain code:code userInfo:userInfo];
}
return 0;
}
return numerator / denominator;
}
@end
int main( int argc, const char * argv[]) {
@autoreleasepool {
Calculator *calculator = [[Calculator alloc] init];
NSError *error = nil ;
NSInteger result = [calculator divide:10 by:0 error:&error];
if (error) {
NSLog ( @"Error: %@" , error.localizedDescription);
} else {
NSLog ( @"Result: %ld" , ( long )result);
}
}
return 0;
}
|
Output:

Output
Explanation:
So here the error in the program is like in a calculator if we are dividing any natural number by zero then we get the error. In this program, this is the error and by the help of the error handling method we can find the error and the lines where the error is by the help of this technique, it is straightforward for the user to identify the error and create a robust code.
Example 3:
In the below Objective-C program, BNSException is implemented.
ObjectiveC
#import <Foundation/Foundation.h>
void performTaskWithException( void ) {
@throw [ NSException exceptionWithName: @"TaskFailureException" reason: @"The task encountered an exception" userInfo: nil ];
}
int main( int argc, const char * argv[]) {
@autoreleasepool {
@try {
performTaskWithException();
NSLog ( @"Task completed successfully" );
} @catch ( NSException *exception) {
NSLog ( @"Exception: %@" , exception.reason);
}
}
return 0;
}
|
Output:
Exception: The task encountered an exception
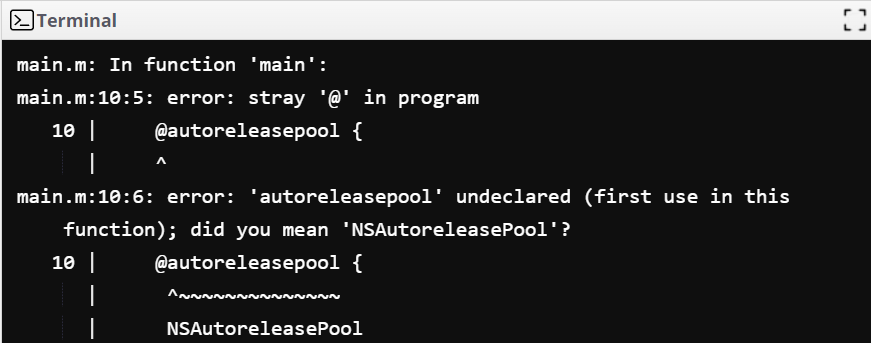
Output
With the help of this method also we can find the errors in the program of Objective-C.
Example 4:
Below Objective-C program demonstrates combining NSError and NSException.
ObjectiveC
#import <Foundation/Foundation.h>
BOOL performTaskWithErrorAndException( NSError **error) {
if (error != NULL ) {
NSDictionary *userInfo = @{
NSLocalizedDescriptionKey : @"Task failed" ,
NSLocalizedFailureReasonErrorKey : @"The task encountered an error" ,
};
*error = [ NSError errorWithDomain: @"com.example.error" code:1 userInfo:userInfo];
return NO ;
}
@throw [ NSException exceptionWithName: @"TaskFailureException" reason: @"The task encountered an exception" userInfo: nil ];
}
int main( int argc, const char * argv[]) {
@autoreleasepool {
NSError *error = nil ;
@try {
if (!performTaskWithErrorAndException(&error)) {
NSLog ( @"Error: %@" , error.localizedDescription);
NSLog ( @"Failure Reason: %@" , error.localizedFailureReason);
} else {
NSLog ( @"Task completed successfully" );
}
} @catch ( NSException *exception) {
NSLog ( @"Exception: %@" , exception.reason);
}
}
return 0;
}
|
In this example, we have used both the classes NSError and NSException in the program to find the errors in the code.
Output:

Output
Share your thoughts in the comments
Please Login to comment...