for loop in Objective-C
Last Updated :
06 Mar, 2023
Like most programming languages, Objective-C also has a repeating statement called for loop. It is a repetition control statement that allows you to repeatedly execute a single instruction or a block of instructions into a specified number of times. unlike other loops like while-loop or do-while loop, for loop has the capability to initialize its all variables, checking the termination condition as well as the updation of the expressions of its variables in one line. The below example shows how a for loop works:
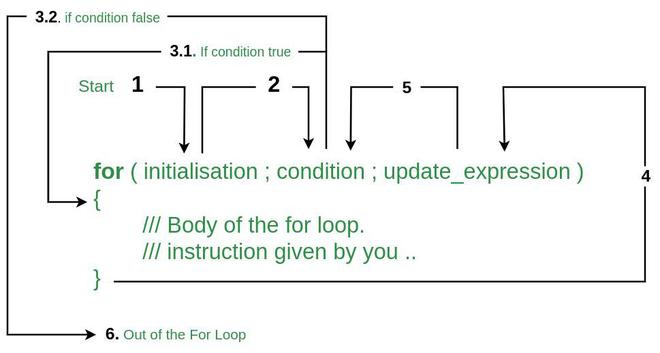
A detailed photo of different sections of for loop.
Syntax:
for (initialisation_of_expression; condition_of_expression; update_expression)
{
/// body of the loop.
/// instruction given by you.
}
Different parts of the For Loop:
- initialisation_of_expression: Here, you can define and initialize the counters or iterators. example : int counter = 1;
- condition_of_expression: Here you have to give the condition, according to which for loop will determine the number of repetitions. For example: counter <= 10 ;
- update_expression: Here, you set an increment or decrement value to update the counter. For example: counter++;
How does For Loop execute
- First, the control flow comes to the initialization part of the for loop and initializes all the declarations.
- Then, the control flow jumps to the conditions section to check the given condition
- if the given condition evaluates true, the flow will jump to the loop body and execute the instructions
- if the condition evaluates false, the control flow will jump out from the loop and execute the lines given after the loop.
- Now, the flow jumps to the updation part and evaluates the updation part.
- Then again, the control flow jumps to step 2 to check the condition with updated values.
- After the condition becomes false, the control flow jumps out from the loop. and execute the next lines …
Control Flow Chart
Here is the control flow chart of for loop:
Example:
ObjectiveC
#import <Foundation/Foundation.h>
int main ()
{
NSAutoreleasePool *myPool = [[ NSAutoreleasePool alloc] init];
int counter;
for (counter = 1; counter <= 10; counter++)
{
NSLog ( @"%d. GeeksForGeeks\n" , counter);
}
return 0;
[myPool drain];
}
|
Output:
2022-11-17 03:43:03.286 main[15947] 1. GeeksForGeeks
2022-11-17 03:43:03.287 main[15947] 2. GeeksForGeeks
2022-11-17 03:43:03.287 main[15947] 3. GeeksForGeeks
2022-11-17 03:43:03.287 main[15947] 4. GeeksForGeeks
2022-11-17 03:43:03.287 main[15947] 5. GeeksForGeeks
2022-11-17 03:43:03.287 main[15947] 6. GeeksForGeeks
2022-11-17 03:43:03.287 main[15947] 7. GeeksForGeeks
2022-11-17 03:43:03.287 main[15947] 8. GeeksForGeeks
2022-11-17 03:43:03.287 main[15947] 9. GeeksForGeeks
2022-11-17 03:43:03.287 main[15947] 10. GeeksForGeeks
Breaking Out from for loop in Objective-C
As you know about the for loop in Objective-C, now can you think of a way to break out of the loop before the condition (written on the first line of for loop) become false. In this situation, the keyword “break” can help you. it allow you to break from the loop, you just have to mention a secondary condition to execute the break statement, which will break out the iteration of the for loop and come out of the loop certainly.
Example:
ObjectiveC
#import <Foundation/Foundation.h>
int main ()
{
NSAutoreleasePool *myPool = [[ NSAutoreleasePool alloc] init];
int counter;
for (counter = 1; counter <= 10; counter++)
{
NSLog ( @"%d. GeeksForGeeks\n" , counter);
if (counter == 7)
{
NSLog ( @"\nBreaking out from the loop...\n" );
break ;
}
}
return 0;
[myPool drain];
}
|
In the place of condition, we define the counter should be less than equal to 10, but inside the loop, we also define if the counter is equal to 7 then break out from the loop, and before breaking print the massage “Breaking out from the loop …”.
Output:
2022-11-17 03:47:04.091 main[22052] 1. GeeksForGeeks
2022-11-17 03:47:04.091 main[22052] 2. GeeksForGeeks
2022-11-17 03:47:04.092 main[22052] 3. GeeksForGeeks
2022-11-17 03:47:04.092 main[22052] 4. GeeksForGeeks
2022-11-17 03:47:04.092 main[22052] 5. GeeksForGeeks
2022-11-17 03:47:04.092 main[22052] 6. GeeksForGeeks
2022-11-17 03:47:04.092 main[22052] 7. GeeksForGeeks
Breaking out from the loop...
By observing the output of the above program, You can see that the message “GeeksForGeeks” is printed only 7 times, then the control goes inside the if section, as the value of the variable counter is equal to 7, controls print the massage “Breaking out from the loop” and exit from the loop.
Nested For Loop
Nested loop means A loop inside another loop. You can create a nested loop by placing one loop inside another. The initialization, condition, and updation part will be written separately for each loop.
Syntax
// Outer for loop …
for (initialisation_of_expression_1; condition_of_expression_1; update_expression_1)
{
// Inner for loop …
for (initialisation_of_expression_2; condition_of_expression_2; update_expression_2)
{
// body of the loop.
// instruction given by you.
}
}
Execution of nested for loops in Objective-C
- Step 1: First, the flow of control will come to the initialization part of the outer for loop.
- Step 2: Jump to condition checking part of the outer for loop.
- Step 2(a): If the evaluated conditions are true, jump to the body of the outer loop.
- Step 2(a-1): will initialize the inner for loop, and after initialization.
- Step 2(a-2): jump to the condition part of the inner for loop.
- Step 2(a-2-1): if the condition is true, jump to the body of the inner loop.
- Step 2(a-2-1-a): executes the instructions.
- Step 2(a-2-1-b): and then jump to the increment/decrement part.
- Step 2(a-2-1-c): jumps to step Step 2(a-2) for condition checking
- Step 2(a-2-2): if the condition is false. The flow will exit from the inner for loop.
- Step 2(a-3): control flow will jump to step 2, for the next iteration.
- Step 2(b): If evaluated conditions are false, the flow will come out of the body of the outer for loop
- Step 3: Flow will jump to the next line present after the outer for loop.
Example:
ObjectiveC
#import <Foundation/Foundation.h>
int main ()
{
NSAutoreleasePool *myPool = [[ NSAutoreleasePool alloc] init];
int i, j;
for (i = 1; i <= 3; i++)
{
NSLog ( @"%d. GeeksForGeeks -----> from outer loop\n" , i);
for (j = 1; j <= 5; j++)
{
NSLog ( @"%d. GeeksForGeeks => from inner loop.\n" , j);
}
}
return 0;
[myPool drain];
}
|
Output :
2022-11-17 03:55:40.337 main[34756] 1. GeeksForGeeks -----> from outer loop
2022-11-17 03:55:40.337 main[34756] 1. GeeksForGeeks => from inner loop.
2022-11-17 03:55:40.337 main[34756] 2. GeeksForGeeks => from inner loop.
2022-11-17 03:55:40.337 main[34756] 3. GeeksForGeeks => from inner loop.
2022-11-17 03:55:40.337 main[34756] 4. GeeksForGeeks => from inner loop.
2022-11-17 03:55:40.337 main[34756] 5. GeeksForGeeks => from inner loop.
2022-11-17 03:55:40.337 main[34756] 2. GeeksForGeeks -----> from outer loop
2022-11-17 03:55:40.337 main[34756] 1. GeeksForGeeks => from inner loop.
2022-11-17 03:55:40.337 main[34756] 2. GeeksForGeeks => from inner loop.
2022-11-17 03:55:40.337 main[34756] 3. GeeksForGeeks => from inner loop.
2022-11-17 03:55:40.337 main[34756] 4. GeeksForGeeks => from inner loop.
2022-11-17 03:55:40.337 main[34756] 5. GeeksForGeeks => from inner loop.
2022-11-17 03:55:40.337 main[34756] 3. GeeksForGeeks -----> from outer loop
2022-11-17 03:55:40.337 main[34756] 1. GeeksForGeeks => from inner loop.
2022-11-17 03:55:40.337 main[34756] 2. GeeksForGeeks => from inner loop.
2022-11-17 03:55:40.337 main[34756] 3. GeeksForGeeks => from inner loop.
2022-11-17 03:55:40.338 main[34756] 4. GeeksForGeeks => from inner loop.
2022-11-17 03:55:40.338 main[34756] 5. GeeksForGeeks => from inner loop.
For Loop with multiple variables
Till now, loop statements are executed by following a single variable or counter. In a loop, you can
- initialize multiple variables.
- check for a complicated logical expression.
- updation of multiple variables can be done inside the respected section of for loop.
Example:
ObjectiveC
#import <Foundation/Foundation.h>
int main ()
{
NSAutoreleasePool *myPool = [[ NSAutoreleasePool alloc] init];
int i, j;
for (i = 1, j = 10; i <= 10 && j >= 1; i++, j--)
{
NSLog ( @"i = %d and j = %d \n" , i, j);
}
return 0;
[myPool drain];
}
|
Output:
2022-11-17 03:59:14.012 main[39122] i = 1 and j = 10
2022-11-17 03:59:14.012 main[39122] i = 2 and j = 9
2022-11-17 03:59:14.012 main[39122] i = 3 and j = 8
2022-11-17 03:59:14.012 main[39122] i = 4 and j = 7
2022-11-17 03:59:14.012 main[39122] i = 5 and j = 6
2022-11-17 03:59:14.012 main[39122] i = 6 and j = 5
2022-11-17 03:59:14.012 main[39122] i = 7 and j = 4
2022-11-17 03:59:14.013 main[39122] i = 8 and j = 3
2022-11-17 03:59:14.013 main[39122] i = 9 and j = 2
2022-11-17 03:59:14.013 main[39122] i = 10 and j = 1
As you can see, two variables ( such as i = 1, j = 0 ) are initialized at the same place and separated by a comma (separator). in the condition checking part, check if i is less than equal to 10 also j should be greater than equal to 1. if any one of the conditions becomes false the loop will terminate. and the updation part, i is incrementing by 1 whereas j is decrementing by 1.
You can use as many variables as you required, and you can nest as many loops as required as long as your requirement get satisfied. but if constraints (time limit, time complexity) are given ( such as linear time ), then you have to think of a clever way to use the loops.
Share your thoughts in the comments
Please Login to comment...