Exception handling in Objective-C
Last Updated :
12 Dec, 2023
Exception handling is an essential aspect of Objective-C programming, enabling developers to manage unforeseen errors effectively. Objective-C provides a robust set of tools and methodologies to handle exceptions, ensuring the stability and reliability of applications. Let’s delve deeper into the nuances of exception handling in Objective-C, exploring its various types, syntax, keywords, and practical examples.
Types and Subtypes of Exceptions
Objective-C categorizes exceptions into several types and subtypes, each addressing specific scenarios:
1. NSException
This serves as the base class for exceptions and includes various subtypes like NSRangeException, NSInvalidArgumentException, etc.
2. Custom Exceptions
Developers can create their own exception types by subclassing NSException to handle application-specific errors.
Objective-C employs several essential keywords for managing exceptions:
- @try: Begins a block of code where exceptions might occur.
- @catch(): Defines blocks that handle exceptions thrown within the @try block. It specifies the exception type to catch and handle.
- @finally: Contains code that executes regardless of whether an exception occurs.
- @throw: Raises exceptions programmatically.
Syntax For @try, @catch, and @finally:
@try {
// Code that might throw exceptions
// …
}
@catch (NSException *exception) {
// Handle specific exceptions
// …
}
@finally {
// Cleanup code executed irrespective of exceptions
// …
}
Syntax For Creating and Handling Custom Exceptions:
// Custom exception subclass
@interface CustomException : NSException
@end
@implementation CustomException
@end
// Usage
@try {
// Code that might throw custom exceptions
// …
if (/* Condition */) {
@throw [CustomException exceptionWithName:@”CustomException” reason:@”Custom exception occurred” userInfo:nil];
}
}
@catch (CustomException *exception) {
// Handle custom exceptions
// …
}
@finally {
// Cleanup code executed irrespective of exceptions
// …
}
Example 1:
Below code demonstrates exception handling for division by zero:
ObjectiveC
#import <Foundation/Foundation.h>
int main( int argc, const char * argv[])
{
@autoreleasepool
{
@try
{
int numerator = 10;
int denominator = 0;
if (denominator == 0)
{
@throw [ NSException exceptionWithName: @"DivideByZeroException"
reason: @"Denominator cannot be zero"
userInfo: nil ];
}
int result = numerator / denominator;
NSLog ( @"Result of division: %d" , result);
}
@catch ( NSException *exception)
{
NSLog ( @"Exception caught: %@" , exception.reason);
}
@finally
{
NSLog ( @"Finally block executed" );
}
}
return 0;
}
|
Output:
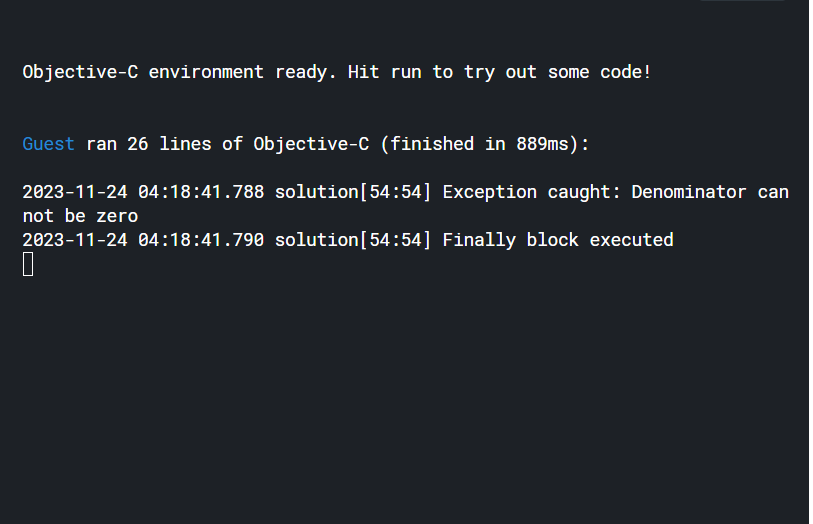
Explanation:
- The program starts with the main function, the entry point of execution, encapsulated within an @autoreleasepool block, managing memory allocation.
- Within this function, there’s a structured exception handling using @try, @catch, and @finally blocks.
- The @try block contains the code where an exception might occur. It initializes two integers, numerator and denominator, and attempts to perform a division operation. Before the division, it checks if the denominator is zero. If it is, an exception is deliberately raised using @throw. This exception is an instance of NSException, customized with a message stating that the denominator cannot be zero.
- If the denominator is not zero, the code proceeds with the division operation, calculating numerator divided by denominator. The result is then printed to the console using NSLog.
- The @catch block immediately follows the @try block and catches any exception of type NSException. It handles the caught exception by printing information about the exception using NSLog.
- Lastly, the @finally block follows the @catch block and contains code that will execute regardless of whether an exception was thrown or not. In this block, a message indicating the execution of the @finally block is logged to the console using NSLog.
Example 2:
Below code demonstrates handling Array Index Out-of-Bounds Exception in Objective-C with @try, @catch, and @finally Blocks.
ObjectiveC
#import <Foundation/Foundation.h>
int main( int argc, const char * argv[])
{
@autoreleasepool
{
NSArray *carModels = @[ @"Sedan" , @"SUV" , @"Hatchback" ];
@try
{
NSUInteger index = 10;
if (index < [carModels count])
{
NSLog ( @"Car model at index %lu: %@" , (unsigned long )index, carModels[index]);
}
else
{
@throw [ NSException exceptionWithName: @"ArrayIndexOutOfBoundsException"
reason: @"Index out of bounds"
userInfo: nil ];
}
}
@catch ( NSException *exception)
{
NSLog ( @"Caught an exception: %@" , exception.reason);
}
@finally
{
NSLog ( @"Cleaning up resources" );
}
}
return 0;
}
|
Output:
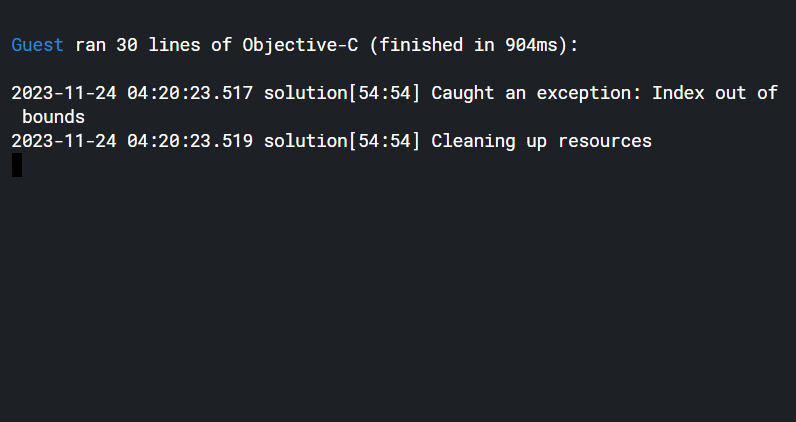
Explanation:
- The given Objective-C code initializes an array carModels containing three car model strings.
- Within a @try block, it attempts to access an element at index 10 of the array.
- Before accessing, it checks if the index is within the array bounds; if it’s within bounds, it prints the car model at that index.
- However, if the index is out of bounds, it triggers an NSException labeled “ArrayIndexOutOfBoundsException” with a reason indicating the index is out of bounds.
- The @catch block catches this exception, logging the reason for the caught exception.
- Finally, the @finally block executes regardless of whether an exception occurred or not, logging a message indicating resource cleanup.
Example 3:
Below code demonstrates exception handling.
ObjectiveC
#import <Foundation/Foundation.h>
int main( int argc, const char * argv[])
{
@autoreleasepool
{
@try
{
NSString *name = nil ;
NSUInteger nameLength = [name length];
NSLog ( @"Length of the name: %lu" , (unsigned long )nameLength);
}
@catch ( NSException *exception)
{
NSLog ( @"Exception caught: %@" , exception.reason);
}
@finally
{
NSLog ( @"Finally block executed" );
}
}
return 0;
}
|
Output:
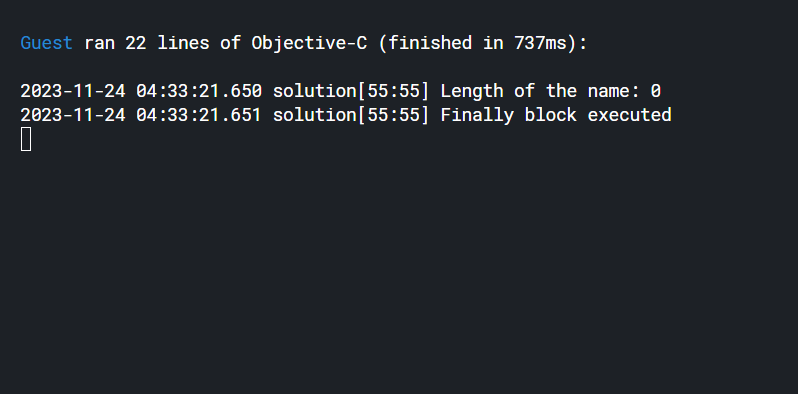
Explanation:
- Inside the @try block, it attempts to get the length of a string (name) that is nil.
- The statement [name length] tries to retrieve the length of the string, but since name is nil and messaging nil is unsafe in Objective-C, this operation would normally raise an exception (NSInvalidArgumentException).
- The @catch block catches this exception and logs the reason for the caught exception.
- Finally, the @finally block executes, indicating the completion of the operation, regardless of whether an exception occurred.
Conclusion
Exception handling in Objective-C is a critical skill for developers to ensure robust and resilient applications. By understanding the syntax, keywords, and types of exceptions, programmers can effectively manage errors and maintain the stability of their codebase.
Share your thoughts in the comments
Please Login to comment...