What is the role of next(err) in error handling middleware in Express JS?
Last Updated :
17 Dec, 2023
Express is the most popular framework for Node.js which is used to build web-based applications and APIs. In application development, error handling is one of the important concepts that provides the aspect to handle the errors that occur in the middleware. The middleware functions have access to the request object, the response object, and the next middleware function which is defined as next.
In this article, we will see the concept of next(err) for error handling in Express. We will see its Syntax and practical example with output.
Prerequisites
Role of next(err) in Error Handling Middleware:
In Express.js the next function is the callback function which is mainly used to pass the control to the next middleware for performing certain tasks. If we use the next function along with the error parameter as (err) then it handles the error which occurs during the processing of the request and passes the control to the next middleware function.
- Pass Control to the Next Middleware: When the next(err) is called, the Express knows that an error has occurred, so it skips the control to the next middleware function which handles the error.
- Error Propagation: The parameter err consists of the information about the error that has occurred. This parameter is mostly the instance of the “Error” object it can be a custom object also. We can also log the error information, which is sent as a response to the client.
- Bypass the Regular Middleware: In the application, when the error has occurred and the next(Err) function is called, the regular middleware functions for the current route are skipped and the control is passed to the next middleware to handle the error.
- Error Response: The middleware that is developed to handle these errors can give the correct response to the client. This can include the logging of errors or sending some specific HTTP status codes that give the information about the error.
Syntax:
app.use((err, req, res, next) => {
// Handle the error or log it
console.error(err);
// Pass the error to the next error-handling middleware
next(err);
});
Steps to use next(err) in error handling middleware in Express.js
Step 1: In the first step, we will create the new folder as next-error by using the below command in the VSCode terminal.
mkdir next-error
cd next-error
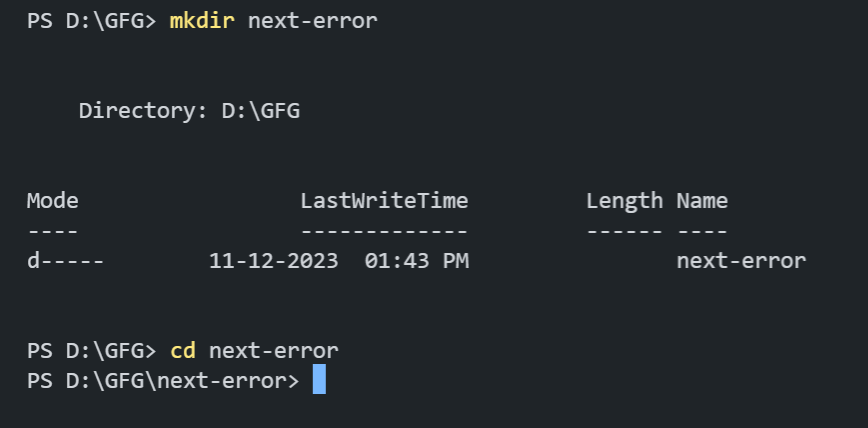
Step 2: After creating the folder, initialize the NPM using the below command. Using this the package.json file will be created.
npm init -y
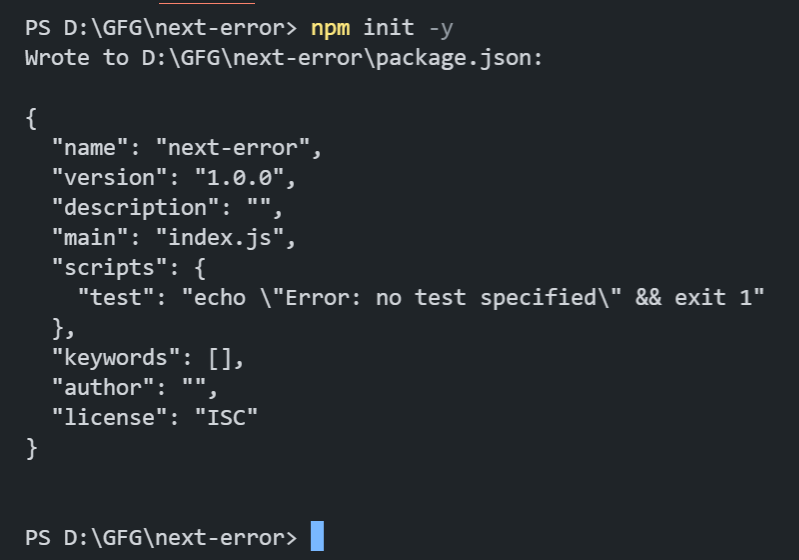
Step 3: Now, we will install the express dependency for our project using the below command.
npm i express
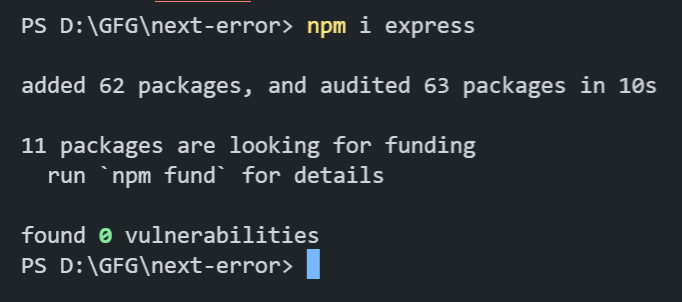
Dependencies:
"dependencies": {
"express": "^4.18.2",
}
Step 4: Now create the below Project Structure of our project which includes the file as app.js.
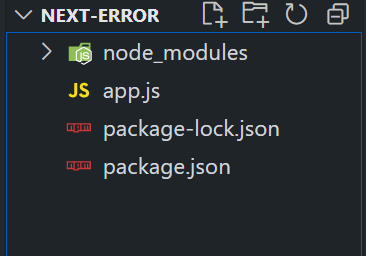
Step 5: Use the below app.js code to use the next(err) in error handling middleware in Express.js.
Javascript
const express = require( "express" );
const app = express();
app.use((req, res, next) => {
const err = new Error( "Failed to fetch data from GeeksforGeeks API" );
err.status = 500;
err.geeksforGeeksData = {
endpoint: "/api/geeks/data" ,
message: `Check if GeeksforGeeks API is
accessible and the data format is correct.`,
};
next(err);
});
app.use((err, req, res, next) => {
console.error(err);
res.status(err.status || 500).json({
error: {
message: err.message || "Something went wrong!" ,
geeksforGeeksData: err.geeksforGeeksData || null ,
},
});
});
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server is running on http:
});
|
To run the application, we need to start the server by using the below command.
node app.js
Output:
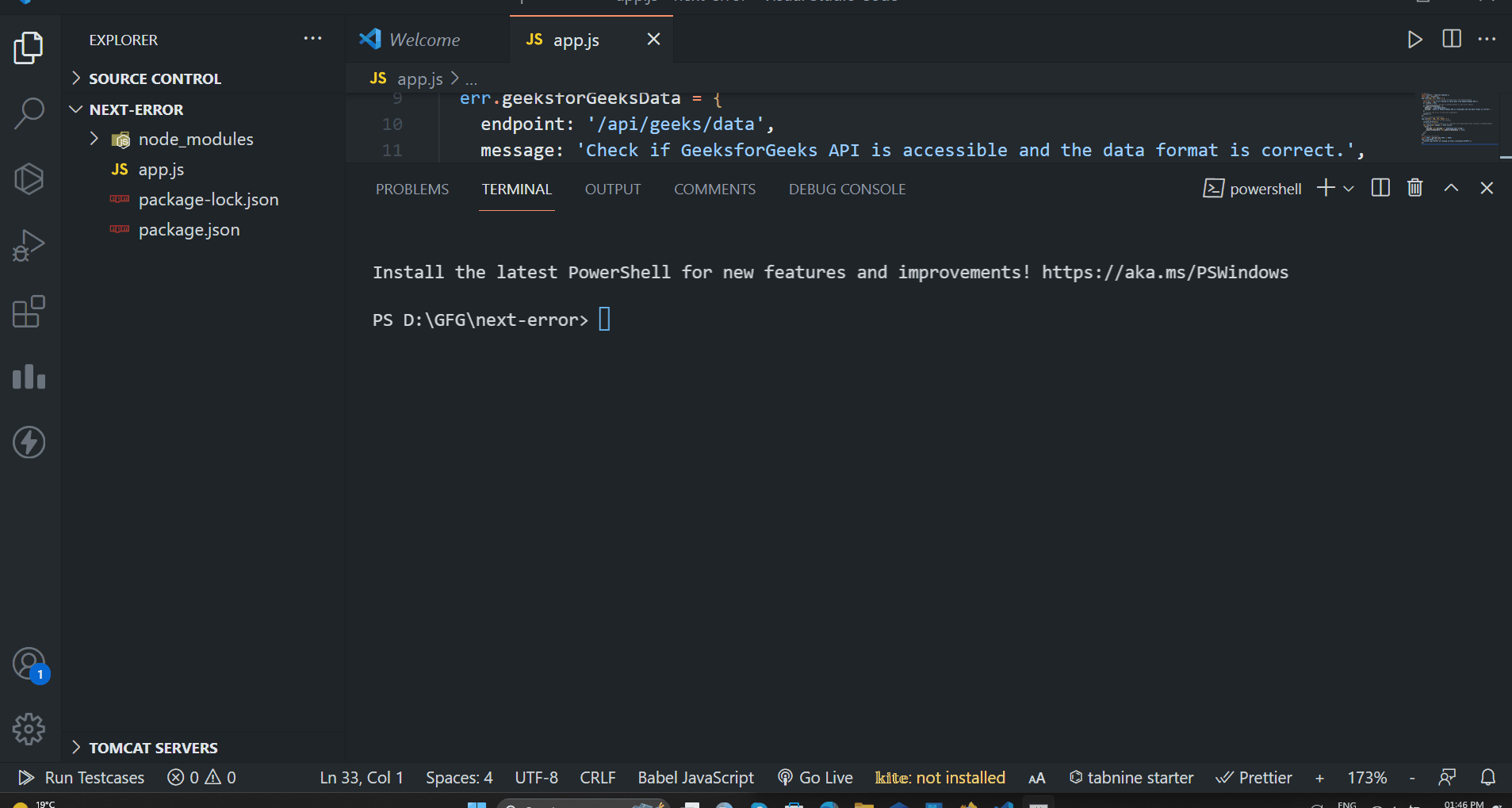
Explanation:
- In the above example, we are showing the error message that there is a failure in fetching data from the GeeksforGeeks API.
- The error is been passed to an error-handling middleware, where we are displaying the error in JSON response.
- Along with this, there is more data provided like endpoint and helpful message that includes more proper error response.
Share your thoughts in the comments
Please Login to comment...