Ensuring Database Resilience and Recovery Strategies for Python Applications
Last Updated :
10 Apr, 2024
In today’s data-driven world, the reliability and availability of your Python applications heavily rely on the resilience of their underlying databases. Database resilience refers to a set of practices designed to guard your data against ability screw-ups, enabling speedy recovery in case of disruptions. By implementing robust recovery strategies, downtime and data loss in case of disruptions and data loss can be minimized, ensuring uninterrupted functionality of applications.
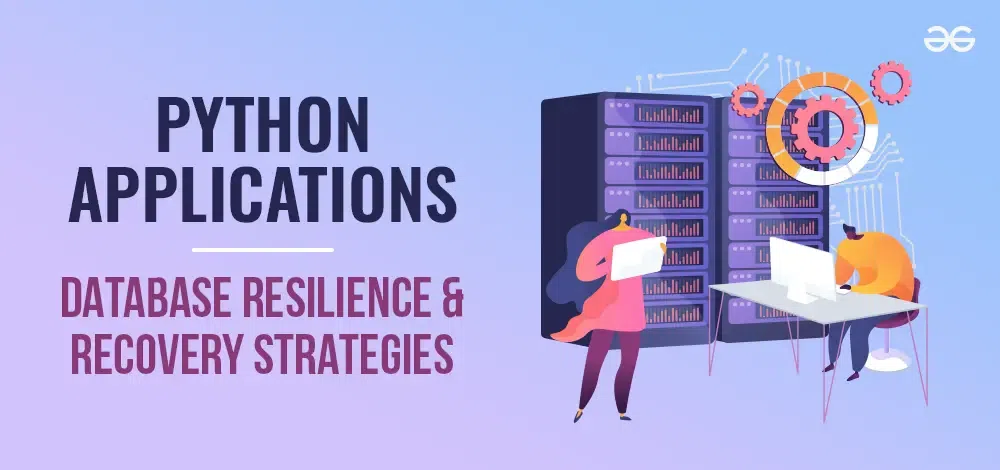
Database Resilience and Recovery Strategies
Key Concepts
- Recovery Time Objective (RTO): The most tolerable time in your application to be unavailable following a catastrophe. Lower RTOs symbolize quicker restoration.
- Recovery Point Objective (RPO): The appropriate quantity of data loss that can be tolerated among the final hit backup and the incident. Lower RPOs reduce facts loss.
- Backups: Regularly scheduled introduction of copies of your database, saved in a separate vicinity for catastrophe restoration. Popular alternatives include full, incremental, and differential backups.
- Data Replication: Maintaining synchronized copies of your database in a geographically wonderful region. This ensures endured operation if the primary database turns into unavailable. Techniques include synchronous and asynchronous replication.
- High Availability (HA): Architectures that limit downtime via employing redundant components. Examples consist of failover clusters, where a standby server seamlessly takes over if the number one server fails.
- Disaster Recovery (DR): A complete plan outlining steps to repair your database and application after a good sized outage. It encompasses procedures for restoring statistics from backups, citing secondary systems, and trying out restoration tactics.
- Database Error Handling: Gracefully coping with exceptions that could get up during database
- Interactions: This entails implementing retry good judgment, logging errors for troubleshooting, and providing informative messages to customers.
- Testing: Regularly testing restoration tactics to verify their effectiveness and become aware of potential issues. This ensures a smooth and efficient healing technique in the event of an actual catastrophe.
Steps to Ensure Robust Database Resilience
- Identify Risks and Requirements: Analyze ability threats for your database, such as hardware screw ups, software bugs, human errors, or cyberattacks. Determine suited RTO and RPO values based to your software’s criticality.
- Implement Backup Automation: Schedule automated backups to run regularly (e.G., each day, hourly) and store them securely in a separate area, ideally bodily or logically awesome (cloud garage may be an awesome option).
- Enable Data Replication (Optional): If your RPO could be very low, set up information replication to hold synchronized copies of your database in a far off vicinity. This reduces the impact of number one database outages.
- Develop a Disaster Recovery Plan: Document a complete plan that outlines recovery methods for diverse situations. Include information on activating backups, restoring facts, citing secondary structures, and trying out recovery processes.
- Implement Database Error Handling: Incorporate errors coping with mechanisms on your Python code to gracefully manage database interplay exceptions. This entails retry good judgment, logging errors, and imparting informative feedback to users.
- Test and Refine: Regularly take a look at your restoration plan and tactics to guarantee their effectiveness. Conduct simulations to pick out and deal with capability problems. Refine your plan based on test effects and ongoing risk assessments.
Recovery Strategies for Python Applications
- Choose a Backup Strategy: Select a backup technique that aligns along with your RPO and storage constraints. Consider complete backups for complete database copies, incremental backups for capturing adjustments since the last full backup, and differential backups for modifications because the closing incremental backup.
- Fault Tolerance: Design applications with fault tolerance in mind to gracefully handle errors and failures without crashing. Utilize Python libraries like ‘try-except’ blocks, ‘finally’ clauses and custom exception handling to manage errors effectively.
- High Availability Architectures: Deploy applications in high availability to minimize downtime and ensure mange errors. For important packages, take into account employing a high-availability (HA) architecture, such as failover clusters, to make certain endured operation if the primary server reports a malfunction.
- Automated Monitoring and Alerting: Implement automated monitoring solution to detect performance issues, anamolies and potential failures in real-time.
- Disaster Recovery Planning: Develop a disaster recovery plans outlines steps to restore operations in the event of catastrophic failures or disasters.
Example of basic error handling and Data Restoration
Here’s a simplified example using Python’s sqlite3 module to illustrate basic blunders dealing with and data restoration from a backup:
Explanation : Each step is wrapped in a try-except block to catch and handle any potential errors. Comments are added to explain each section of the code. Simulated errors are introduced to demonstrate how error handling works. A placeholder message is added for database backup creation. The database connection is closed at the end to ensure proper cleanup.
Python3
import sqlite3
# Connect to the SQLite database
try:
conn = sqlite3.connect('example.db')
print("Connected to the database")
except sqlite3.Error as e:
print("Error connecting to the database:", e)
# Create a table (if it doesn't exist)
try:
cursor = conn.cursor()
cursor.execute('''CREATE TABLE IF NOT EXISTS users
(id INTEGER PRIMARY KEY, name TEXT, age INTEGER)''')
print("Table 'users' created successfully")
except sqlite3.Error as e:
print("Error creating table:", e)
# Insert some sample data
try:
cursor.execute("INSERT INTO users (name, age) VALUES (?, ?)", ('John Doe', 30))
cursor.execute("INSERT INTO users (name, age) VALUES (?, ?)", ('Jane Smith', 25))
conn.commit()
print("Sample data inserted successfully")
except sqlite3.Error as e:
print("Error inserting data:", e)
# Simulate a database error (e.g., table does not exist)
try:
cursor.execute("SELECT * FROM non_existent_table")
rows = cursor.fetchall()
for row in rows:
print(row)
except sqlite3.Error as e:
print("Error executing query:", e)
# Backup the database
try:
# You would typically back up the database file to another location
# For simplicity, we'll just print a message here
print("Database backup created successfully")
except Exception as e:
print("Error creating database backup:", e)
# Close the database connection
try:
conn.close()
print("Database connection closed")
except sqlite3.Error as e:
print("Error closing database connection:", e)
Output:
Connected to the database
Table 'users' created successfully
Sample data inserted successfully
Error executing query: no such table: non_existent_table
Database backup created successfully
Database connection closed
Conclusion
In conclusion, ensuring the resilience of databases is essential for the reliability and availability of Python applications. By implementing robust practices such as defining Recovery Time Objectives (RTOs) and Recovery Point Objectives (RPOs), establishing backup strategies, enabling data replication, designing high availability architectures, and developing comprehensive disaster recovery plans, developers can mitigate the impact of potential failures and disruptions.
Share your thoughts in the comments
Please Login to comment...