Dockerizing a Python Flask Application with a Database
Last Updated :
19 Oct, 2023
Applications are packaged, distributed, and run via Docker within Docker containers throughout the process of “dockerizing”. The exact environment and dependencies must be installed in order to run that application on another local machine when it is developed under a specific environment and dependencies. As a result, it is very difficult to proceed on another local machine. This is where docker comes for containerizing applications. Flask web applications will be deployed inside of Docker containers since they don’t require a specific system environment to run. it consists of two containers one is for flask and the other is for database. these containers are connected using “docker-compose.yml” file. here we are going to use database mongoDB.
Key Terminologies
The Dockerized Flask application consists of :
- Flask: it is micro web framework based on python programming language used for eveloping backend REST API microservices.
- MongoDB: it is a Document type key-value pair NOSQL database, stores data in json format.
- Docker: it is used to build, test, and deploy applications
- Containerization: it is technique used to packing the application’s dependencies into one or more containers.
- App.py : where the main python(Flask server) code is written
- Templates: where html and css (front-end) files are stored
- Reqirements.txt : the requirements to run the appliction are written
- Dockerfile: used to build docker image
- Docker-compose.yml : used to configure services, networks, connection, volumes
Step-By-Step Process
1. Create A Directory
Start by creating a directory for your project. In this example, we’ll name it
mkdir <Name Of the Directory> (flask_to_do_list)
open directory in any IDE , i am using VS Code
2. Create The Flask Application
Here, I am going to create a simple to do list flask application where i can add and remove daily tasks
app.py:
Python3
from flask import Flask, render_template, request, redirect, url_for
from pymongo import MongoClient
from bson import ObjectId
app = Flask(__name__)
client = MongoClient(host = 'test_mongodb' ,port = 27017 , username = 'root' , password = 'pass' ,authSource = "admin" )
db = client.mytododb
tasks_collection = db.tasks
@app .route( '/' )
def index():
tasks = tasks_collection.find()
return render_template( 'index.html' , tasks = tasks)
@app .route( '/add_task' , methods = [ 'POST' ])
def add_task():
task_name = request.form.get( 'task_name' )
if task_name:
tasks_collection.insert_one({ 'name' : task_name})
return redirect(url_for( 'index' ))
@app .route( '/delete_task/<task_id>' , methods = [ 'GET' ])
def delete_task(task_id):
tasks_collection.delete_one({ '_id' : ObjectId(task_id)})
return redirect(url_for( 'index' ))
if __name__ = = '__main__' :
app.run(host = '0.0.0.0' ,debug = True )
|
3. Set Up Front-End
Create another directory named templates, it consists of front-end (html) codes.inside templates directory index.html:
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content = "width=device-width, initial-scale=1.0" >
< title >Todo List</ title >
</ head >
< body >
< h1 >Todo List</ h1 >
< form action = "/add_task" method = "POST" >
< input type = "text" name = "task_name" placeholder = "Task name" >
< button type = "submit" >Add Task</ button >
</ form >
< ul >
{% for task in tasks %}
< li >{{ task.name }} < a href = "/delete_task/{{ task._id }}" >Delete</ a ></ li >
{% endfor %}
</ ul >
</ body >
</ html >
|
now we can run our flask application if the dependencies are installed on particular system.
4. Create a Dockerfile
Dockerfile is used to specify base image, copying the application code to container, set up environment and dependencies.create file named “Dockerfile” without any dot extension at the end insert the following code into it.
Dockerfile :
FROM python:3.6
ADD . /app
WORKDIR /app
RUN pip install -r requirements.txt
6. Configure docker-compose.yml
The docker-compose.yml file is used to define services , networks ,ports, connection between containers and volumes. The ports are given to Flask app is 5000 and for database is 27017
docker-compose.yml :
web:
build: .
command: python -u app.py
ports:
- "5000:5000"
volumes:
- .:/app
links:
- db
db:
image: mongo:latest
hostname: test_mongodb
environment:
- MONGO_INITDB_DATABASE=animal_db
- MONGO_INITDB_ROOT_USERNAME=root
- MONGO_INITDB_ROOT_PASSWORD=pass
ports:
- 27017:27017
7. Define Dependencies
Requirements.txt is used to install the dependencies for application in the image to run in the container.
requirements.txt:
flask
pymongo
Now all the files are created and their code is also written. The directory structure in the VS Code:
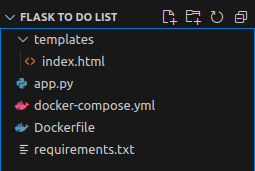
8. Build Docker Images
In your terminal, navigate to the project directory and build the Docker image of the Flask application with MongoDB by running:
sudo docker-compose up
All Set, building of images is done, now we have to start pulling the images and start containers.
9. Running Docker Container
Make new terminal and run the following command to build the docker image of flask application with database MongoDB:
sudo docker-compose up
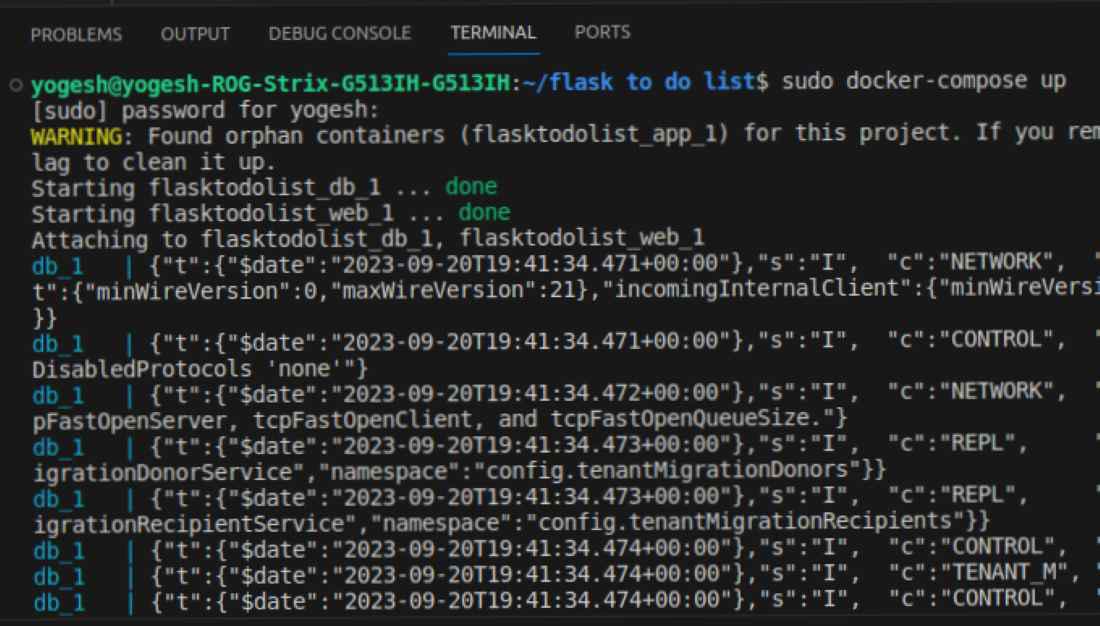
you can also refer to screenshots below for more clarity
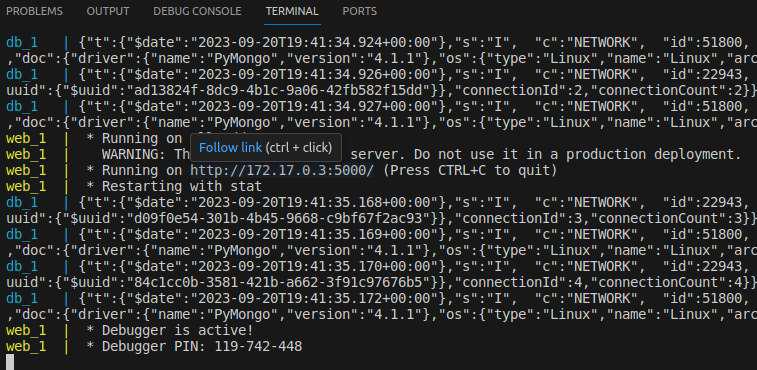
9. Test the Application
You can click on the link generated in the terminal after running of application image to test application on browser which is running inside the docker containers.Testing our application on browser with the link format is “http://<docker-machine-ip>:5000/” my docker machine ip is 172.17.0.3 then the link becomes: http://172.17.0.3:5000/
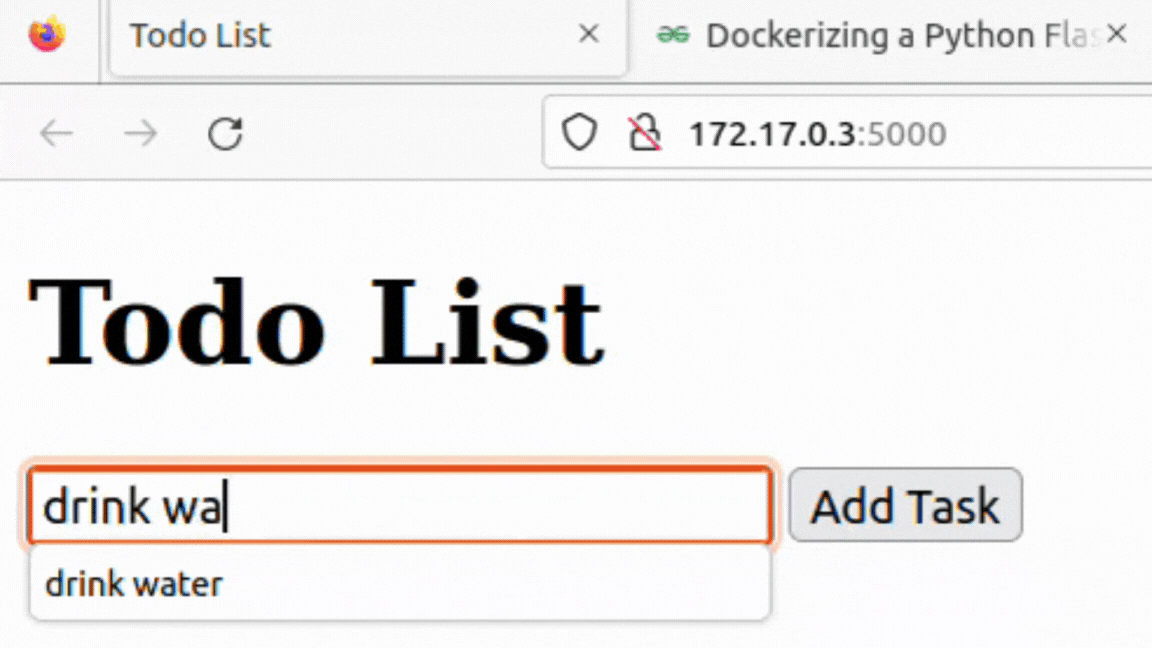
All done, the Dockerized Python Flask Application with a Database MongoDB is running in the container of docker and you can access it on the localhost port 5000 with system’s ip addresss
FAQs on Dockerizing a Python Flask Application with a Database
1. PostgreSQL can be used instead of mongoDB?
Yes, you can use postgresql instead of mongoDB or any other relational database and non relational database any type of database .
2. Does Dockerizing application speeds up deployment?
Yes, Dockerizing application speeds up deployment, as it do not requires to install dependencies and creation of environment for the new system.
3. Can i build other docker application rather than to do list?
I build to do list applications as demo application you can build any application to dockerized container application of any application for deployment purpose
4. Django can be used instead of flask?
Sure, Django is also a python’s web framework and it provides more features for building web applications than Flask. if you to work on big projects then you can definitely use Django
5. what are advantages of Dockerizing application?
Dockerizing application saves a lot more time from development, testing to deployment. as it creates consistent development environment. provides flexibility for application while development and testing independent of system and its envriment. scalability for applications, their user growth is in very high
Share your thoughts in the comments
Please Login to comment...