Draw Colorful Spiral Web Using Turtle Graphics in Python
Last Updated :
20 Oct, 2020
Prerequisite: Turtle Basics
“Turtle” is a Python feature like a drawing board, which lets us command a turtle to draw all over it. This comes packed with the standard Python package and need not be installed externally.
Methods used:
- forward(value): moves the turtle in the forward direction.
- turtle.Pen(): setup the turtle pen
- speed(value): changes the speed of the turtle
- width(value): set the width
- left(value): moves the turtle left.
- bgcolor(color_name): changes background-color
Approach:
- Import turtle.
- Define colors using the list data structure in python.
- Setup a turtle pen for drawing the Spiral Web.
- Start making the Spiral Web according to your logic.
Below is the implementation of the above approach.
Python3
import turtle
colors = [ 'red' , 'yellow' , 'green' , 'purple' , 'blue' , 'orange' ]
t = turtle.Pen()
t.speed( 10 )
turtle.bgcolor( "black" )
for x in range ( 200 ):
t.pencolor(colors[x % 6 ])
t.width(x / 100 + 1 )
t.forward(x)
t.left( 59 )
turtle.done()
t.speed( 10 )
turtle.bgcolor( "black" )
for x in range ( 200 ):
t.pencolor(colors[x % 6 ])
t.width(x / 100 + 1 )
t.forward(x)
t.left( 59 )
turtle.done()
|
Output:
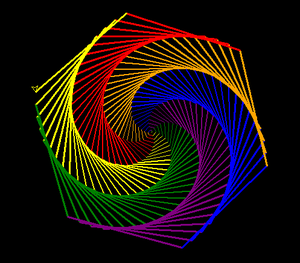
Share your thoughts in the comments
Please Login to comment...