Node.js is an open-source, asynchronous event-driven javascript runtime that is used to run javascript applications. It is widely used for traditional websites and as API servers. At the same time, a docker container is an isolated, deployable unit that packages an application along with its dependencies, making it highly scalable and maintainable. In this article, we will create a docker container for node.js and run a simple express.js application on the container.
What is Docker Container?
A docker is an open platform that provides the docker runtime. It makes the development, running, and deployment of applications straightforward. A docker container is quite popular because it is lightweight and efficient in using the underlying system resources. It shares the host OS’s kernel, unlike a VM machine which makes it faster and capable of handling many simultaneous requests. Read here to learn more about docker.
What is Node.js?
Node.js is a server environment that is open-source and free to use. It provides the javascript runtime so that the javascript applications can run on the server. It also enables javascript code to run outside a browser. It does a lot more, like generating dynamic page content, creating, saving, closing files on the server, and handling databases in the backend.
Why Use Docker Container for Node.js?
One of the problems developers face is having different environments for the development of an application and the deployment of the same. One might develop their local system, which might be totally different from a cloud instance where the application is finally deployed.
A docker container solves this problem, and It uses the concept of images that stores a record of a docker container at a particular time like all the libraries. It is required to run an application along with their versions. Then a developer can share this image with others to have the same environment as the developer does and run the application.
A docker container is the best tool for microservice to know more about Docker architecture adaptation. Many companies are shifting to using dockers because an application running on docker is more maintainable, easily modifiable, and highly scalable.
Dockerizing a Node.js web app
Let’s start with an effortless express application that prints “Hello World! This is Nodejs from a docker container” on visiting the root endpoint.
- To create a folder named express_app and move inside the folder using the following commands.
mkdir express_app
cd express_app
- Now, create a file named app.js as follows.
Create the Node.js app
Create a file that consists of all the files and dependencies required that describe your app and name it as a “package. Jason”.
{
"name": "docker-example",
"version": "1.0.0",
"description": "",
"main": "app.js",
"scripts": {
"start": "nodemon app.js",
"test": "echo \"Error: no test specified\" && exit 1"
},
"author": "",
"license": "ISC",
"dependencies": {
"express": "^4.17.1",
"nodemon": "^2.0.12"
}
}
- Now, initialize the node project using the following command.
npm init
This will add the package.json file, which holds information about our projects like scripts, dependencies, and versions. It will ask for the package’s name, version, and many others (you can go with defaults by pressing ENTER).
- Install the Express library and add it to the package.json file as a dependency.
npm install --save express
- Install a tool called nodemon that automatically restarts the node application when it detects any changes.
npm install --save nodemon
We are explicitly adding these dependencies to our package.json file to download them when we run this application inside a docker container.
- Add a script to the scripts part of the package.json file to run the application with nodemon. The file contents will be as follows:
Express.js
Express is a framework for Node.js that helps developers organize their web applications into MVC architecture on the server side. It makes web applications fast and easy as compared to developing an application using only Node.js.
For example, A node.js application might need MongoDB in the backend, and Express is the framework that helps manage everything like routes, requests, and views.
Then, create a server.js file that defines a web app using the Express.js framework:
// import and create an express app
const express = require('express');
const app = express()
// message as response
msg = "Hello world! this is nodejs in a docker container.."
// create an end point of the api
app.get('/', (req, res) => res.send(msg));
// now run the application and start listening
// on port 3000
app.listen(3000, () => {
console.log("app running on port 3000...");
})
At this stage, we can run our application on our local system using the following command:
npm run start
But actually, we want to dockerize this application. For that, we need to create an image by providing information like which runtime we need, the port the app will use, and the files needed that are available on our local system.
- Create a Dockerfile that contains all the information about the image that will run the application. The docker software understands this special file, and it is used to build an image.
Creating a Dockerfile
First, create a file name as Dockerfile.
FROM node:latest
WORKDIR /app
COPY package.json /app
RUN npm install
COPY . /app
CMD ["npm", "start"]
Explanation of Dockerfile
- The FROM takes the name of the base image to use optionally with its version.
- WORKDIR states the directory that holds the files of the application in the container.
- COPY command copies the package.json file to the app directory.
- The RUN command runs the provided command to install all the dependencies mentioned in the package.json file.
- Then COPY is used to copy the rest of the files to the app directory in the container.
- Finally, we provide the script to run the application.
- The folder structure after creating all the required files is as follows:
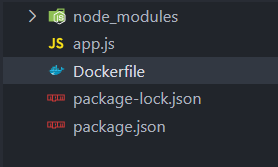
- Finally, use this command to build the image we will run in our docker container.
Building Your Image
After creating a Dockerfile build an image using the help Dockerfile by using the following command.
docker build -t docker-container-nodejs .
The command uses the flag -t to specify the name of the image, and then we have to give the address where. Then, our Dockerfile is situated; since we are in the directory while running the commands, we can use the period, which stands for the current directory.
- Confirm that the image has been created.
docker images
The output will be a list of images in your system. It should contain our recently created image with the name we provided with the -t flag.
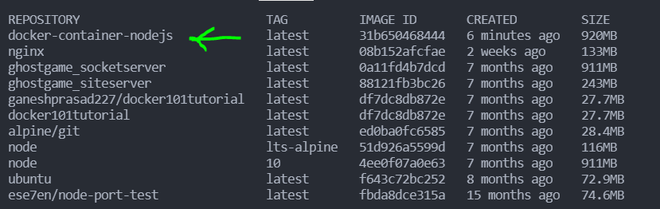
- To run the docker container with this image, use the following command.
Run the image
Run the image as a container and deploy the application in the form of containers.
docker run -d -p 8000:3000 -v address_to_app_locally:/app docker-container-nodejs
The above command runs a docker container. The flag -p is used to map the local port 8000 to the container’s port 3000 where our application is running. The -v flag is used to mount our application files into the app directory of the container. It also needs the image name that we want to run in our container, which is, in this case, docker-container-nodejs that.
Test the Application
Visit this address localhost:8000, and our express application will return the following response.
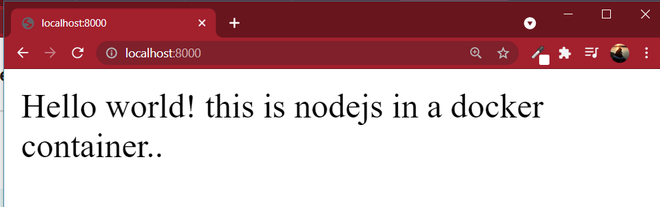
Express app on a docker container
Conclusion
In this article, we learned about Docker containers, images, and their benefits. Then we learned how to create an image that will run on a docker container. Finally, we created a small Express application to demonstrate how to run an application using Node.js running in a docker container.
Share your thoughts in the comments
Please Login to comment...