In Django, soft delete is a clever way to manage data removal. Instead of getting rid of records forever in the database, it takes a gentler approach. Here’s the idea: When you want to delete certain data, you don’t wipe it out completely. Instead, you place a special marker, like a flag, to show that it’s not active or “deleted”. This way, you have a record of what you removed, and you can easily bring it back if you need to. Think of it as moving things to a virtual trash can rather than tossing them into oblivion, so you have the option to recover them later if you change your mind. It’s a flexible and safer way to manage your data.
Implement Soft Delete in Django
To install Django follow these steps.
Starting the Project
To start the project use this command
django-admin startproject core
cd core
To start the app use this command
python manage.py startapp home
File Structure :
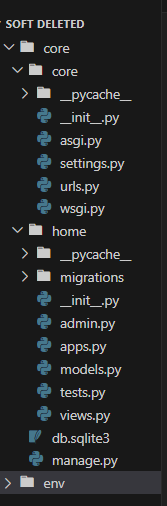
File Structure
Register app in ‘settings.py’ file like shown below in lnstalled apps :
INSTALLED_APPS = [
"django.contrib.admin",
"django.contrib.auth",
"django.contrib.contenttypes",
"django.contrib.sessions",
"django.contrib.messages",
"django.contrib.staticfiles",
"home", ..//App name
]
Setting up the files
views.py: The code is part of a Django web application, a Python web framework.It imports the render and redirect functions from django.shortcuts.Defines a view function named login_view to handle login-related functionality. It Contains a placeholder comment for the login view logic.Returns an HTTP response by rendering the ‘login.html’ template using the render function.
Python3
from django.shortcuts import render
from django.shortcuts import render, redirect
def login_view(request):
return render(request, 'login.html' )
|
models.py: In this code, we define a ‘SoftDelete’ base model with a boolean field ‘is_deleted’ to mark records as deleted or not. It also includes two methods, soft_deleted and restore, for soft deletion and restoration of records, respectively. The Color model is a concrete model that inherits from ‘SoftDelete’. It includes fields for storing color names and hexadecimal codes.The ‘NonDeleted’ manager is used to filter out non-deleted records when querying the database, while the everything manager retrieves all records, including deleted ones. The ‘abstract = True’ option in the Meta class makes the ‘SoftDelete’ model abstract, preventing it from creating a separate database table. Instead, other models can inherit from it to enable soft deletion functionality.
Python3
from django.db import models
class NonDeleted(models.Manager):
def get_queryset( self ):
return super ().get_queryset(). filter (is_deleted = False )
class SoftDelete(models.Model):
is_deleted = models.BooleanField(default = False )
everything = models.Manager()
objects = NonDeleted()
def soft_deleted( self ):
self .is_deleted = True
self .save()
def restore( self ):
self .is_deleted = False
self .save()
class Meta:
abstract = True
class Color(SoftDelete):
color_name = models.CharField(max_length = 100 )
color_hex_code = models.CharField(max_length = 100 )
def __str__( self ):
return self .color_name
|
admin.py: Here we are registering the models.
Python3
from django.contrib import admin
from .models import *
admin.site.register(Color)
|
urls.py: This file is used to define the URLs .
Python3
from django.contrib import admin
from django.urls import path
urlpatterns = [
path( 'admin/' , admin.site.urls),
]
|
Deployement of the project
Run these commands to apply the migrations:
python3 manage.py makemigrations
python3 manage.py migrate
Run the server with the help of following command:
python3 manage.py runserver
Output
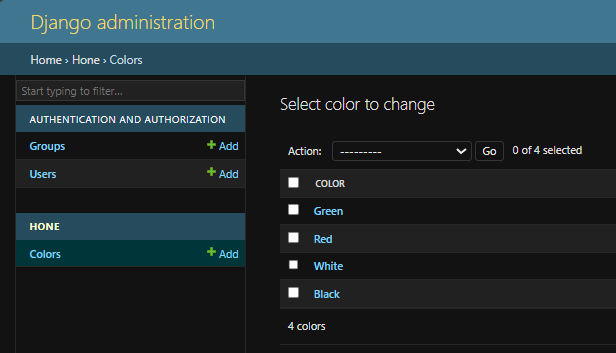
Database
Checking Django Soft Delete functionality
In this portion, we’ll explore how to perform a soft delete operation in a Django application using the Python shell. We’ll walk through the steps, from opening the Python shell to executing commands that demonstrate soft deletion and restoration of a model object.
1) Open the Python shell in your Django project by running the following command in your terminal:
python manage.py shell
This command starts the Python shell within the context of your Django project.
2) Import the necessary models from your home app. In this example, we assume there’s a model named Color in the home app:
from home.models import Color
This step allows you to work with the Color model in the Python shell.
3) Retrieve all the Color objects and display them:
colors = Color.objects.all()
colors
This command fetches all the color objects from the database and displays them in the shell.
4) Soft delete a specific color, for example, the first color in the list:
colors[0].soft_delete()
This command calls a custom soft_delete method on the selected color object, marking it as “soft-deleted” by setting the is_deleted field to True.
5) Verify the soft delete operation by retrieving all the Color objects again:
colors = Color.objects.all()
colors
After the soft delete, you’ll see that the first color object’s is_deleted field is now set to True.
6) Check the is_deleted field of the first color to confirm it’s marked as deleted:
colors[0].is_deleted
This command specifically checks the is_deleted field of the first color in the list.
7) If you want to restore a soft-deleted color (assuming there’s a restore method for this purpose), you can do it like this:
colors = Color.everything.all()
colors[0].restore()
This command calls a custom restore method on the soft-deleted color, making it accessible again.
8) Finally, check the Color objects once more to see if the color has been successfully restored
colors = Color.objects.all()
colors
The restored color should now appear in the list of colors.
These commands demonstrate how to perform a soft delete and restoration of a color object in Django using the Python shell. Please ensure that you replace Color with the actual name of your model and adapt the commands to your specific Django application’s structure.
Complete video:
Conclusion:
In conclusion, we’ve covered the essential steps for performing a soft delete operation in a Django application using the Python shell. By following these instructions, you can effectively mark records as “soft-deleted” without physically removing them from your database. This approach allows for better data management and retention while maintaining data integrity. Remember to adapt these steps to your specific Django project’s structure and model names, and feel free to explore further customization as needed to fit your application’s requirements
Share your thoughts in the comments
Please Login to comment...