Design a Login Modal in Tailwind CSS
Last Updated :
12 Mar, 2024
Tailwind CSS allows us to design styled and responsive awesome applications using pre-defined classes that enhance the look and feel of the application. For designing the Login Modal in Tailwind CSS, we can use utility classes that build the responsive user interface and clean elements.
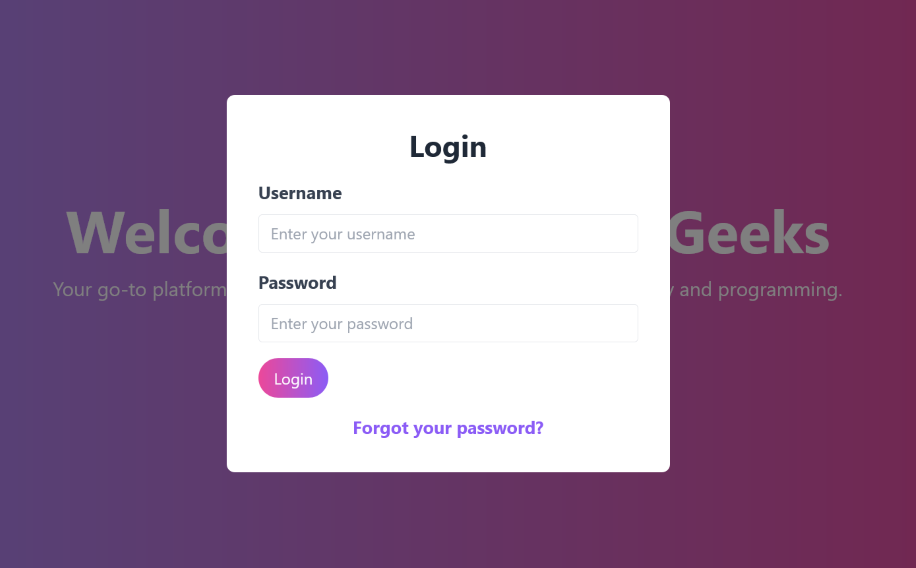
Approach
- Create a basic Landing Page using HTML with a Login Button to open the Login Modal Form.
- Include Tailwind CSS in the project. We can include it by using the CDN link.
- Use the Tailwind CSS classes to style the modal Login form with input fields for Username and Password. The classes will ensure a clean and responsive design.
- In the main JavaScript code, use the addEventListener() to open the Login Modal form when the login button is clicked from the Landing Page.
Example: The below code is an implementation for creating login modal using Tailwind CSS.
HTML<!DOCTYPE html>
<head>
<link rel="stylesheet" href=
"https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.5.1/css/all.min.css">
<link rel="stylesheet" href=
"https://cdn.jsdelivr.net/npm/tailwindcss@2.2.19/dist/tailwind.min.css">
<link rel="stylesheet" href=
"https://cdnjs.cloudflare.com/ajax/libs/animate.css/4.1.1/animate.min.css">
<link rel="stylesheet" href=
"https://fonts.googleapis.com/css2?family=Montserrat:wght@400;700&display=swap">
<title>GeeksforGeeks Form</title>
</head>
<body class="bg-gradient-to-r from-purple-400 to-pink-500
min-h-screen flex items-center justify-center">
<div class="text-center text-white mx-4 md:mx-8 lg:mx-20">
<h1 class="text-4xl md:text-5xl lg:text-6xl
font-bold mb-4
animate__animated animate__fadeInDown">
Welcome to GeeksforGeeks
</h1>
<p class="text-base md:text-lg lg:text-xl
mb-6 animate__animated animate__fadeInDown">
Your go-to platform for learning and exploring
the world of technology and programming.
</p>
<button id="loginBtn" class="bg-white text-purple-500 py-2
px-6 rounded-full font-bold text-lg
hover:underline focus:outline-none
focus:shadow-outline transform
hover:scale-105 transition
duration-300 animate__animated
animate__fadeInUp">
Login
</button>
</div>
<div id="loginModal" class="hidden fixed top-0 left-0 w-full
h-full bg-black bg-opacity-50 flex items-center justify-center">
<div class="bg-white rounded-lg p-8 w-full
max-w-md animate__animated animate__fadeIn">
<div class="flex justify-end">
<button id="closeBtn" class="text-gray-800 text-xl">
×
</button>
</div>
<h2 class="text-xl md:text-2xl lg:text-3xl
font-bold mb-4 text-center text-gray-800">
Login
</h2>
<form id="loginForm" class="space-y-4">
<div>
<label for="username" class="block text-gray-700 text-sm
md:text-base lg:text-lg font-bold mb-2">
Username
</label>
<input class="appearance-none border rounded
w-full py-2 px-3 leading-tight focus:outline-none
focus:shadow-outline"
id="username" type="text"
placeholder="Enter your username">
<p id="usernameError" class="text-red-500 text-sm"></p>
</div>
<div>
<label class="block text-gray-700 text-sm md:text-base
lg:text-lg font-bold mb-2" for="password">
Password
</label>
<div class="relative">
<input class="appearance-none border rounded w-full
py-2 px-3 leading-tight focus:outline-none
focus:shadow-outline"
id="password" type="password"
placeholder="Enter your password">
<button id="togglePassword" class="absolute top-0 right-0
mt-3 mr-4 text-gray-700 cursor-pointer
focus:outline-none"
type="button">
<i id="eyeIcon" class="far fa-eye"></i>
</button>
</div>
<p id="passwordError" class="text-red-500 text-sm"></p>
</div>
<button type="button" id="loginSubmit" class="bg-gradient-to-r
from-pink-500 to-purple-500 text-white py-2 px-4
rounded-full focus:outline-none focus:shadow-outline
transform hover:scale-105 transition duration-300">
Login
</button>
</form>
<div class="text-center mt-4">
<a href="#" id="forgotPasswordLink"
class="text-purple-500 text-sm
md:text-base lg:text-lg font-bold hover:underline">
Forgot your password?
</a>
<p id="forgotPasswordError" class="text-red-500 text-sm"></p>
</div>
</div>
</div>
<div id="forgotPasswordModal"
class="hidden fixed top-0 left-0 w-full h-full
bg-black bg-opacity-50 flex items-center justify-center">
<div class="bg-white rounded-lg p-8 w-full max-w-md
animate__animated animate__fadeIn">
<div class="flex justify-end">
<button id="closeForgotPasswordBtn"
class="text-gray-800 text-xl">
×
</button>
</div>
<h2 class="text-xl md:text-2xl lg:text-3xl font-bold
mb-4 text-center text-gray-800">
Forgot Password
</h2>
<form id="forgotPasswordForm" class="space-y-4">
<div>
<label class="block text-gray-700 text-sm md:text-base
lg:text-lg font-bold mb-2" for="email">
Email
</label>
<input
class="appearance-none border rounded w-full
py-2 px-3 leading-tight focus:outline-none
focus:shadow-outline" id="email"
type="email" placeholder="Enter your email">
<p id="emailError" class="text-red-500 text-sm"></p>
</div>
<button type="button" id="forgotPasswordSubmit"
class="bg-gradient-to-r from-pink-500 to-purple-500
text-white py-2 px-4 rounded-full focus:outline-none
focus:shadow-outline transform hover:scale-105
transition duration-300">
Submit
</button>
</form>
</div>
</div>
<script src="script.js"></script>
</body>
</html>
Javascriptdocument.getElementById('loginBtn').
addEventListener('click', function () {
document.getElementById('loginModal').
classList.remove('hidden');
});
document.getElementById('closeBtn').
addEventListener('click', function () {
document.getElementById('loginModal').
classList.add('hidden');
});
document.getElementById('togglePassword').
addEventListener('click', function (event) {
event.preventDefault();
let passwordInput =
document.getElementById('password');
let eyeIcon =
document.getElementById('eyeIcon');
if (passwordInput.type === 'password') {
passwordInput.type = 'text';
eyeIcon.classList.remove('fa-eye');
eyeIcon.classList.add('fa-eye-slash');
} else {
passwordInput.type = 'password';
eyeIcon.classList.remove('fa-eye-slash');
eyeIcon.classList.add('fa-eye');
}
});
document.getElementById('loginSubmit').
addEventListener('click', function (event) {
event.preventDefault();
let username =
document.getElementById('username').value;
let password =
document.getElementById('password').value;
document.getElementById('usernameError').innerText = '';
document.getElementById('passwordError').innerText = '';
document.getElementById('forgotPasswordError').innerText = '';
if (username.trim() === '') {
document.getElementById('usernameError').
innerText = 'Username cannot be empty';
return;
}
if (password.trim() === '') {
document.getElementById('passwordError').
innerText = 'Password cannot be empty';
return;
}
else if (password.length < 8) {
document.getElementById('passwordError').
innerText = 'Password must be at least 8 characters long';
return;
}
else if (!/[0-9]/.test(password) ||
!/[!@#$%^&*(),.?":{}|<>]/.test(password)) {
document.getElementById('passwordError').
innerText = 'Password must contain at least one' +
'numeric digit and one special character';
return;
}
alert('Form submitted!');
});
document.getElementById('forgotPasswordLink').
addEventListener('click', function () {
document.getElementById('forgotPasswordModal').
classList.remove('hidden');
});
document.getElementById('closeForgotPasswordBtn').
addEventListener('click', function () {
document.getElementById('forgotPasswordModal').
classList.add('hidden');
});
document.getElementById('forgotPasswordSubmit').
addEventListener('click', function (event) {
event.preventDefault();
let email = document.getElementById('email').value;
document.getElementById('emailError').innerText = '';
if (email.trim() === '' || !isValidEmail(email)) {
document.getElementById('emailError').
innerText = 'Please enter a valid email address';
return;
}
alert('Reset Link Sent Successfully!');
document.getElementById('forgotPasswordModal').
classList.add('hidden');
});
function isValidEmail(email) {
let emailRegex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
return emailRegex.test(email);
}
Output:
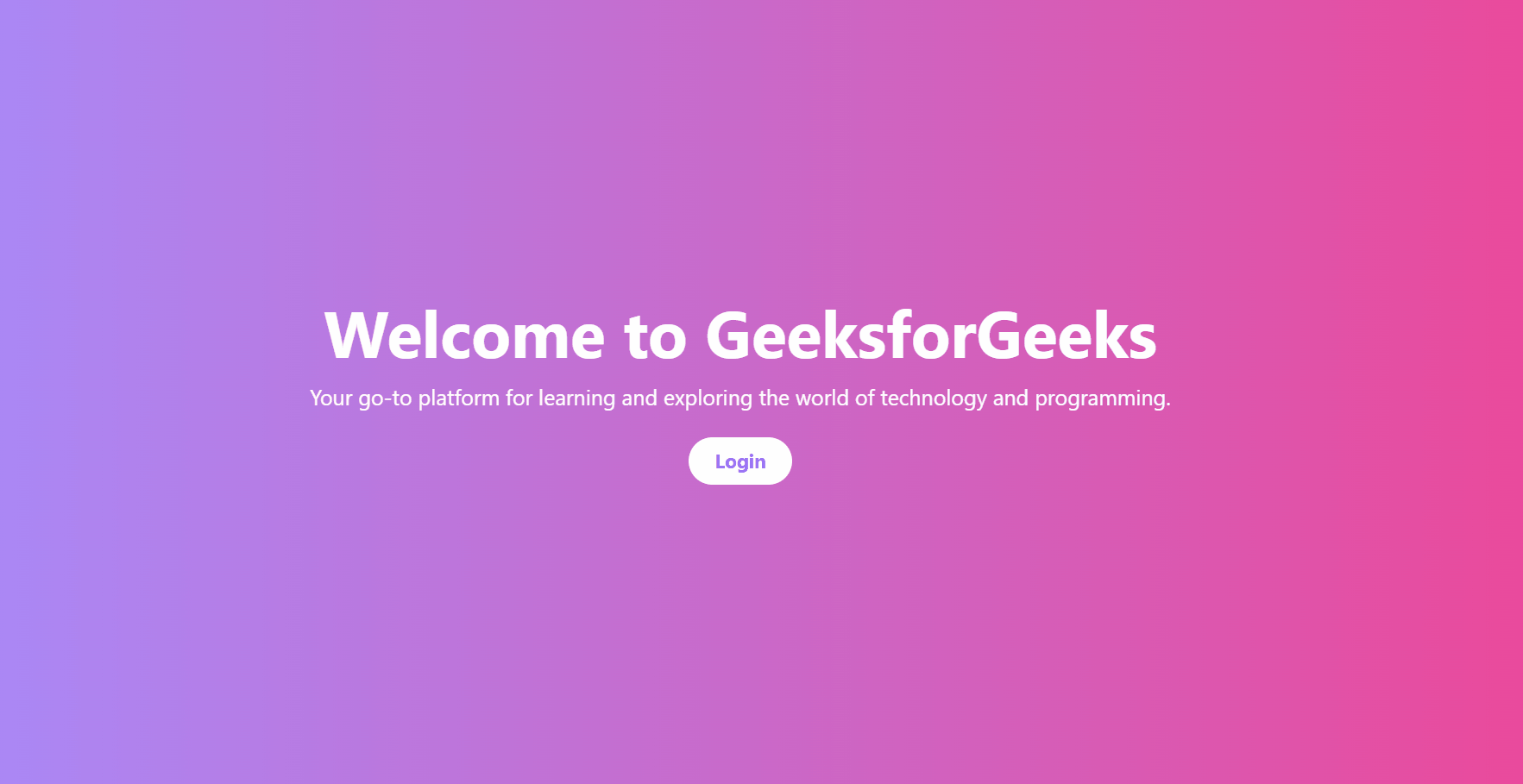
Share your thoughts in the comments
Please Login to comment...