Docker is a set of platforms as a service (PaaS) products that use Operating system-level virtualization to deliver software in packages called containers. Containers are isolated from one another and bundle their software, libraries, and configuration files; they can communicate through well-defined channels.
Linear regression is a supervised machine learning algorithm that computes the linear relationship between a dependent variable and one or more independent features.
We can deploy an ML model as a Web Application on Docker. Deploying a Linear Regression ML model as a web application on Docker involves several steps.
- Train the Linear Regression Model
- Build the Flask Web Application
- Dockerize the Flask App
- Build and Run Docker Image
- Test the Application
Deploying Linear Regression Model
Prerequisites: Software and Tools
- Python: The core programming language used for both model training and web application development. Ensure you have Python 3.6 or newer installed, as it includes features and syntax used in modern applications.
- PIP: Python’s package installer, which you’ll use to install Python libraries required for your project.
- Docker: Essential for containerizing your Flask application, making it easy to deploy across different environments without compatibility issues. Install Docker Desktop (for Windows or Mac) or Docker Engine (for Linux).
Python Libraries:
Ensure you have the following Python libraries installed. These can be installed via pip using pip install library-name:
- Flask: A micro web framework for Python, used to build the web application.
- NumPy: A library for numerical computing in Python, often used for handling arrays and numerical operations in data preprocessing.
- Pandas: Provides data structures and data analysis tools in Python. It’s especially useful if you’re manipulating datasets before training your model.
- Scikit-learn (sklearn): A machine learning library for Python, used for training the Linear Regression model and any other preprocessing or machine learning tasks.
- Pickle: While not a library that needs to be installed separately (it’s part of Python’s standard library), it’s crucial for saving and loading your trained model.
Structure of the project
Your project structure should look like this, The Dockerfile should be saved without any extensions and the HTML file should be saved under the templates folder.
/your-flask-app
/templates
index.html
app.py
requirements.txt
Dockerfile
...
Train the Linear Regression Model
The first step in deploying a machine learning model as a web application is to train the model itself. For this project, we use the Boston housing dataset, focusing on three significant features: the average number of rooms per dwelling (RM), the percentage of the lower status of the population (LSTAT), and the per capita crime rate by town (CRIM). These features are chosen for their strong influence on housing prices and their ease of understanding by users.
The two lines involving pd.read_csv
and np.hstack
process and structure the data by extracting relevant columns and combining them into a feature matrix. The model is trained on a subset of features, and the trained model is saved using pickle.
Using Python’s sklearn library, we split the dataset into training and testing sets to validate the model’s performance. The linear regression model from sklearn.linear_model is trained on the training set. After training, the model is saved to disk using Pickle, allowing us to load it into our Flask application later.
Python3
import pandas as pd
import numpy as np
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
import pickle
raw_df = pd.read_csv(data_url, sep = "\s+" , skiprows = 22 , header = None )
data = np.hstack([raw_df.values[:: 2 , :], raw_df.values[ 1 :: 2 , : 2 ]])
target = raw_df.values[ 1 :: 2 , 2 ]
selected_features = data[:, [ 5 , 10 , 12 ]]
X_train, X_test, y_train, y_test = train_test_split(selected_features, target, test_size = 0.2 , random_state = 42 )
model = LinearRegression()
model.fit(X_train, y_train)
with open ( 'model.pkl' , 'wb' ) as model_file:
pickle.dump(model, model_file)
|
Build the Flask Web Application
With the model trained, the next step is to create a Flask web application that can use the model to make predictions. The Flask app includes a simple route that renders an HTML form where users can input values for RM, LSTAT, and CRIM. Another route accepts POST requests from this form, uses the model to predict the Boston housing price based on the input values, and displays the prediction.
index.html
HTML
<!DOCTYPE html>
< html >
< head >
< title >Linear Regression Model Prediction</ title >
< style >
body {
font-family: Arial, sans-serif;
margin: 20px;
padding: 20px;
background-color: #f4f4f4;
}
h2 {
color: #333;
}
form {
background: #fff;
padding: 20px;
border-radius: 8px;
box-shadow: 0 2px 4px rgba(0, 0, 0, 0.1);
}
div {
margin-bottom: 10px;
}
label {
margin-bottom: 5px;
display: block;
color: #666;
}
input[type="text"] {
width: 100%;
padding: 8px;
margin: 4px 0;
box-sizing: border-box;
border-radius: 4px;
border: 1px solid #ddd;
font-size: 14px;
}
button {
background-color: #007bff;
color: white;
padding: 10px 24px;
border: none;
border-radius: 4px;
cursor: pointer;
font-size: 16px;
}
button:hover {
background-color: #0056b3;
}
h3 {
color: green;
}
</ style >
</ head >
< body >
< h2 >Predict Boston Housing Prices</ h2 >
< form action = "/predict" method = "post" >
< div >
< label for = "RM" >Average number of rooms (RM):</ label >
< input type = "text" id = "RM" name = "RM" required>
</ div >
< div >
< label for = "PTRATIO" >Pupil-teacher ratio by town (PTRATIO):</ label >
< input type = "text" id = "PTRATIO" name = "PTRATIO" required>
</ div >
< div >
< label for = "LSTAT" >% lower status of the population (LSTAT):</ label >
< input type = "text" id = "LSTAT" name = "LSTAT" required>
</ div >
< button type = "submit" >Predict</ button >
</ form >
{% if prediction_text %}
< h3 >{{ prediction_text }}</ h3 >
{% endif %}
</ body >
</ html >
|
Save the HTML file in the templates folder.
app.py
Here, the Flask app deploys a trained linear regression model to predict house prices. The model, loaded from ‘model.pkl’, is applied when the user submits a form on the website.
In this Flask web application, the predict route is defined to handle POST requests. When a user submits a form on the web page, it sends a POST request to the ‘/predict’ endpoint. The values from the form are extracted, converted to floats, and reshaped into a NumPy array to ensure that the input data has the correct format for making predictions using the trained model. The request.form.values()
method returns the values submitted through the form as strings. Converting them to floats is necessary because the model expects numerical input. Reshaping into a NumPy array with reshape(1, -1)
is done to create a 2D array with a single row, matching the expected input shape for the predict
method of the linear regression model, which typically requires a 2D array as input.
The trained linear regression model (model) then predicts the house price based on these features. Finally, the predicted price is rendered on the web page using the ‘index.html’ template. The result is then displayed on the webpage. The app runs on the local server when executed.
Python3
from flask import Flask, request, jsonify, render_template
import pickle
import numpy as np
app = Flask(__name__)
with open ( 'model.pkl' , 'rb' ) as model_file:
model = pickle.load(model_file)
@app .route( '/' )
def home():
return render_template( 'index.html' )
@app .route( '/predict' , methods = [ 'POST' ])
def predict():
features = [ float (x) for x in request.form.values()]
final_features = np.array(features).reshape( 1 , - 1 )
prediction = model.predict(final_features)
return render_template( 'index.html' , prediction_text = 'Predicted House Price: ${:.2f}' . format (prediction[ 0 ]))
if __name__ = = '__main__' :
app.run(debug = False , host = '0.0.0.0' )
|
The Flask app is straightforward, with app.py serving as the main file. It loads the pickled model, defines routes for the homepage (with the form) and the prediction endpoint, and uses templates for the HTML content.
Dockerize the Flask App
To ensure that our Flask application is easy to deploy and runs consistently across different environments, we dockerize it. Dockerizing involves creating a Dockerfile that specifies the environment, dependencies, and commands needed to run the app.
Dockerfile
FROM python:3.8-slim
WORKDIR /app
COPY requirements.txt requirements.txt
RUN pip install -r requirements.txt
COPY . .
EXPOSE 5000
CMD [“python”, “app.py”]
Requirements.txt
Flask
scikit-learn
numpy
requests
pandas
The Dockerfile for our Flask app is based on python:3.8-slim, installs dependencies from a requirements.txt file, copies the application files into the container, and sets the command to run the Flask app. This setup encapsulates the application and its environment, making deployment seamless.
Note: Save the Dockerfile with no extension selected.
Build and Run Docker Image
With the Dockerfile in place, we build the Docker image using the command executed in the directory containing the Dockerfile and application code.
docker build -t linear-regression-web-app .
This command creates a Docker image named linear-regression-web-app.
.gif)
creating a Docker image
After building the image, we run it with
docker run -p 5000:5000 linear-regression-web-app
This command starts a container from the image, mapping port 5000 inside the container to port 5000 on the host, allowing us to access the Flask app by visiting http://localhost:5000 in a web browser.
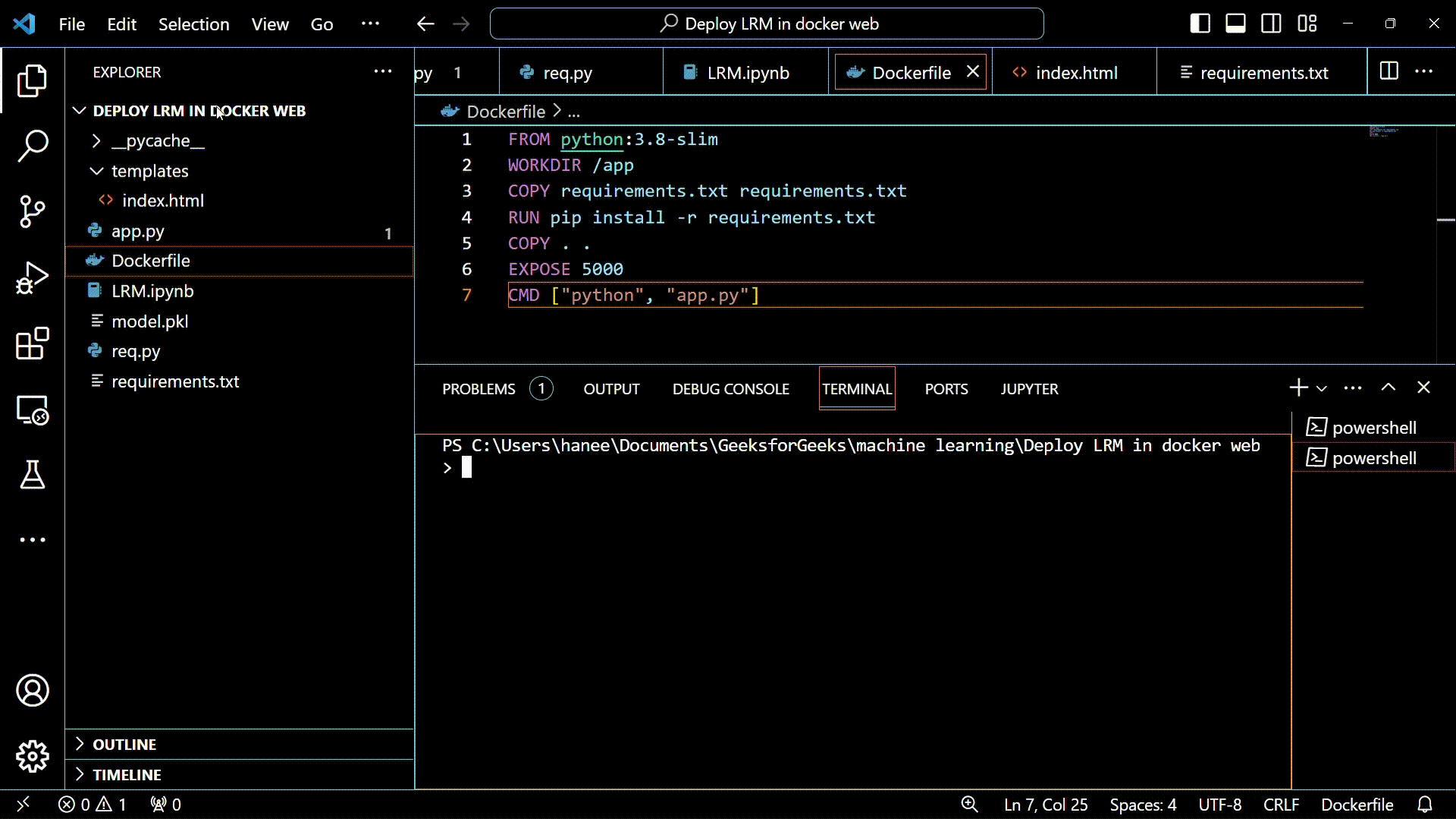
starting container from the image
Test the Application
The final step is to test the web application to ensure it works as expected. This involves navigating to http://localhost:5000, entering values for RM, LSTAT, and CRIM into the form, and submitting it. The application should display the predicted housing price based on the input values.
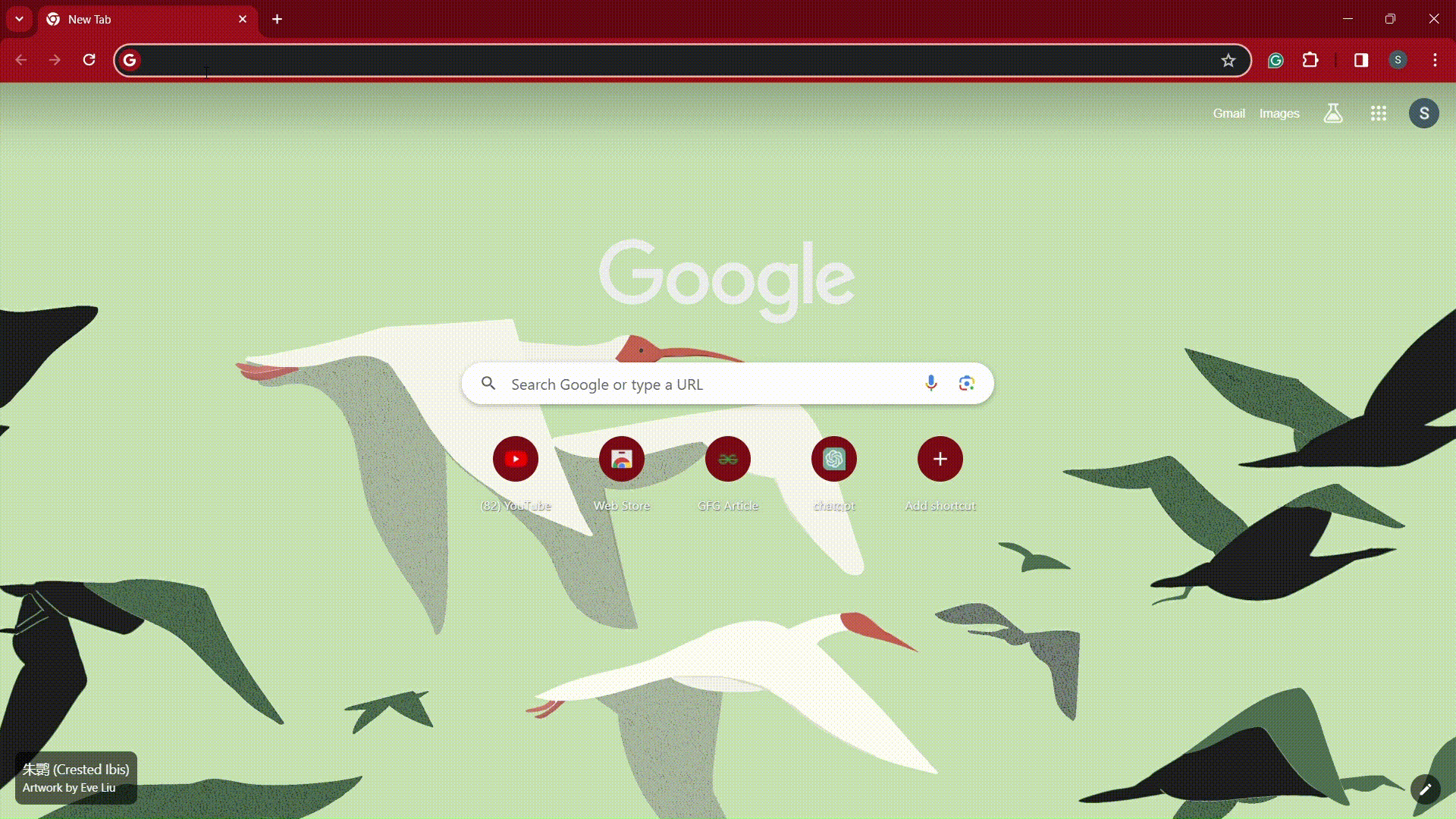
http://localhost:5000
Conclusion
Deploying a Linear Regression model as a web application involves training the model, creating a Flask application to use the model, dockerizing the Flask app for easy deployment, and testing to ensure everything works as expected. This process demonstrates how machine learning models can be made accessible and useful in real-world applications, providing valuable insights or predictions based on user input.
Share your thoughts in the comments
Please Login to comment...