Create URL Bookmark Manager Using Django
Last Updated :
07 Mar, 2024
This article explains how to make a tool called a Bookmark Organizer with Django for a website. With this tool, we can add, create, update, delete, and edit bookmarks easily. We just need to input the title and link, and we can save them. Then, we can click on the link anytime to visit the website.
What is a Bookmark Organizer?
A Bookmark Organizer is a tool or application that helps users manage and keep track of their bookmarks or favorite web links. It allows users to add, create, update, delete, and edit bookmarks in an organized manner. Typically, users can save the title and link of a website, making it convenient to revisit the websites they find interesting or frequently visit. The Bookmark Organizer aims to simplify and enhance the user’s experience.
Create URL Bookmark Manager Using Django
Below, are the step-by-step guide to creating URL Bookmark Manager Using Django:
Starting the Project Folder
To start the project use this command
django-admin startproject core
cd core
To start the app use this command
python manage.py startapp home
Now add this app to the ‘settings.py’
INSTALLED_APPS = [
"django.contrib.admin",
"django.contrib.auth",
"django.contrib.contenttypes",
"django.contrib.sessions",
"django.contrib.messages",
"django.contrib.staticfiles",
"home",
]
File Structure
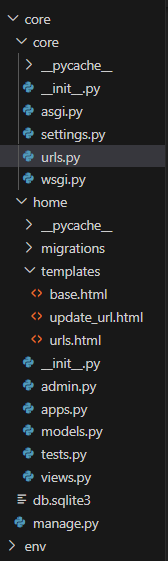
File Structure
Setting Necessary Files
models.py: This Django code defines a `User` model with two fields: `name` as a character field with a maximum length of 255, and `url` as a URL field. The `__str__` method specifies that the string representation.
Python3
from django.db import models
class User(models.Model):
name = models.CharField(max_length = 255 )
url = models.URLField()
def __str__( self ):
return self .name
|
views.py:The Django views in this code manage a web application for handling URLs. The urls view creates, lists, and filters User instances. The delete_url view removes a specific User instance. The update_url view edits a User instance, allowing users to modify the associated name and URL.
Python3
from django.shortcuts import render, redirect
from .models import User
from django.http import HttpResponse
def urls(request):
if request.method = = 'POST' :
data = request.POST
name = data.get( 'name' )
url = data.get( 'url' )
User.objects.create(
name = name,
url = url,
)
return redirect( '/' )
queryset = User.objects. all ()
if request.GET.get( 'search' ):
queryset = queryset. filter (
name__icontains = request.GET.get( 'search' ))
context = { 'urls' : queryset}
return render(request, 'urls.html' , context)
def delete_url(request, id ):
queryset = User.objects.get( id = id )
queryset.delete()
return redirect( '/' )
def update_url(request, id ):
queryset = User.objects.get( id = id )
if request.method = = 'POST' :
data = request.POST
name = data.get( 'name' )
url = data.get( 'url' )
queryset.name = name
queryset.url = url
queryset.save()
return redirect( '/' )
context = { 'url' : queryset}
return render(request, 'update_url.html' , context)
|
urls.py: The Django `urlpatterns` configures routes for the admin interface, main page (`urls` view), and routes to update or delete specific User instances. These paths are associated with their corresponding views.
Python3
from django.contrib import admin
from django.urls import path
from home import views
urlpatterns = [
path( 'admin/' , admin.site.urls),
path('', views.urls),
path( 'update_url/<id>' , views.update_url, name = 'update_url' ),
path( 'delete_url/<id>' , views.delete_url, name = 'delete_url' ),
]
|
Creating GUI
base.html : This HTML template defines a basic structure with a title dynamically set by the {{page}} variable. It includes a styled table, and the {% block start %}{% endblock %} allows extension
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content = "width=device-width, initial-scale=1.0" >
< title >{{page}}</ title >
< style >
table {
width: 80%;
margin: 20px auto;
border-collapse: collapse;
}
th,
td {
padding: 10px;
text-align: left;
border: 1px solid #ccc;
}
th {
background-color: #f2f2f2;
}
tr:nth-child(even) {
background-color: #f2f2f2;
}
tr:hover {
background-color: #ddd;
}
</ style >
</ head >
< body >
{% block start %}
{% endblock %}
< script >
console.log('Hey Django')
</ script >
</ body >
</ html >
|
update_url.html : The provided Django template extends ‘base.html,’ incorporating Bootstrap styles for a form to update URL information. The form, centered in a card layout, pre-populates fields with existing data from the url variable.
HTML
{% extends "base.html" %}
{% block start %}
< style >
.text{
color: green;
font-weight: bold;
}
</ style >
< div class = "container mt-5" >
< form class = "col-6 mx-auto card p-3 shadow-lg" method = "post" enctype = "multipart/form-data" >
{% csrf_token %}
< h2 class = "text text-center" > GeeksforGeeks </ h2 >
< h4 >Update URL</ h4 >
< hr >
< div class = "form-group" >
< label for = "exampleInputEmail1" > Website Name</ label >
< input name = "name" value = "{{url.name}}" type = "text" class = "form-control" required>
</ div >
< div class = "form-group" >
< label for = "exampleInputPassword1" > URL</ label >
< textarea name = "url" value = "" class = "form-control"
required>{{url.url}}</ textarea >
</ div >
< button type = "submit" class = "btn btn-success" >Update URL</ button >
</ form >
</ div >
{% endblock %}
base.html: It is the base HTML file which is extended by all other HTML files.
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content = "width=device-width, initial-scale=1.0" >
< title >{{page}}</ title >
< style >
table {
width: 80%;
margin: 20px auto;
border-collapse: collapse;
}
th,
td {
padding: 10px;
text-align: left;
border: 1px solid #ccc;
}
th {
background-color: #f2f2f2;
}
tr:nth-child(even) {
background-color: #f2f2f2;
}
tr:hover {
background-color: #ddd;
}
</ style >
</ head >
< body >
< script >
console.log('Hey Django')
</ script >
</ body >
</ html >
|
urls.html : This Django template extends ‘base.html,’ featuring a Bootstrap-styled form to add URLs and a table displaying existing entries. The form includes fields for website name and URL. The table dynamically lists URLs with options to view, delete, and update each entry.
HTML
{% extends "base.html" %}
{% block start %}
< style >
.text{
color: green;
font-weight: bold;
}
</ style >
< div class = "container mt-5" >
< form class = "col-6 mx-auto card p-3 shadow-lg" method = "post" enctype = "multipart/form-data" >
{% csrf_token %}
< h2 class = "text text-center" > GeeksforGeeks </ h2 >
< br >
< h3 >BookMark Manager</ h3 >
< hr >
< div class = "form-group" >
< label for = "exampleInputEmail1" >Website Name</ label >
< input name = "name" type = "text" class = "form-control" required>
</ div >
< div class = "form-group" >
< label for = "exampleInputPassword1" >URL </ label >
< input name = "url" type = "url" class = "form-control" required>
</ div >
< button type = "submit" class = "btn btn-success" >Add URL</ button >
</ form >
< hr >
< div class = "class mt-5" >
< form action = "" >
< div class = "max-auto col-6" >
</ form >
</ div >
</ div >
< table class = "table mt-5" >
< thead >
< tr >
< th scope = "col" >#</ th >
< th scope = "col" >Website Name</ th >
< th scope = "col" > URL</ th >
< th scope = "col" >Actions</ th >
</ tr >
</ thead >
< tbody >
{% for url in urls %}
< tr >
< th scope = "row" >{{forloop.counter}}</ th >
< td >{{url.name}}</ td >
< td >< a href = "{{url.url}}" target = "_blank" class = "btn btn-warning" >View</ a ></ td >
< td >
< a href = "/delete_url/{{url.id}}" class = "btn btn-danger m-2" >Delete</ a >
< a href = "/update_url/{{url.id }}" class = "btn btn-success" >Update</ a >
</ td >
</ tr >
{% endfor %}
</ tbody >
</ table >
</ div >
{% endblock %}
|
admin.py:Here we are registering our models.
Python3
from django.contrib import admin
from .models import *
from django.db.models import Sum
admin.site.register(User)
|
Deployment of the Project
Run these commands to apply the migrations:
python3 manage.py makemigrations
python3 manage.py migrate
Run the server with the help of following command:
python3 manage.py runserver
Output
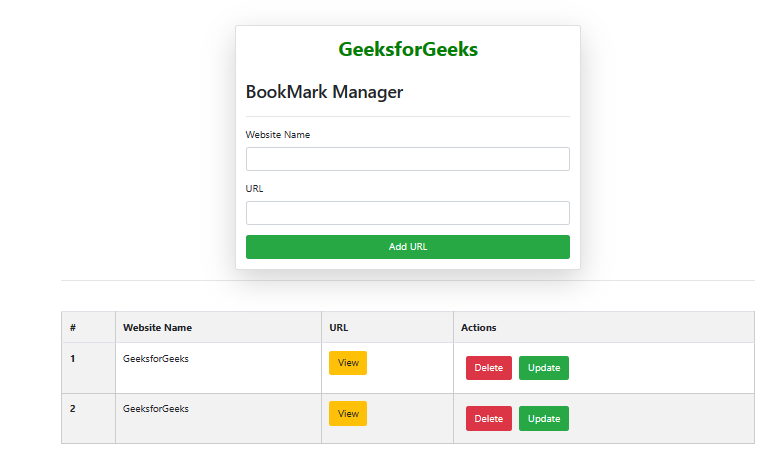
urls.html
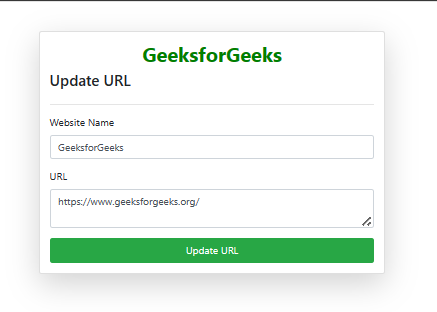
update_url.html
Share your thoughts in the comments
Please Login to comment...