Create Dynamic checkboxGroupInput in Shiny package Using R
Last Updated :
09 Jun, 2023
The Shiny is an R package that allows us to build interactive web applications with R Programming Language. One of the core components of a Shiny app is the ability to dynamically update the content of the app based on user input.
- One way to do this is by using the checkboxGroupInput.
- which allows users to select multiple options from a list of checkboxes.
- The checkboxGroupInput can be dynamically updated based on user input.
- The checkboxGroupInput is a UI element that allows the user to select multiple options from a list of choices.
- To create a dynamic checkboxGroupInput in Shiny.
- We can use the updateCheckboxGroupInput() function in the server code.
Syntax :
checkboxGroupInput(inputId, label, choices, selected = NULL, inline = FALSE)
where,
- inputId: the unique identifier for input control.
- label: The text to display above the input control.
- choices: A vector of options to display as checkboxes.
- selected: The default selected options.
- inline: Whether to display the checkboxes inline or on separate lines.
Dynamic checkboxGroupInput Based on User Input
In this example, the user can input a number between 1 and 5 and the app will generate that many checkboxes in the checkboxGroupInput.
R
library (shiny)
ui <- fluidPage (
sidebarLayout (
sidebarPanel (
numericInput ( "num_boxes" , "Number of Boxes" ,
1, min = 1, max = 5)
),
mainPanel (
checkboxGroupInput ( "boxes" , "Select Boxes" )
)
)
)
server <- function (input, output, session) {
observe ({
boxes <- 1:input$num_boxes
updateCheckboxGroupInput (session, "boxes" ,
label = NULL ,
choices = boxes,
selected = NULL )
})
}
shinyApp (ui, server)
|
Output:
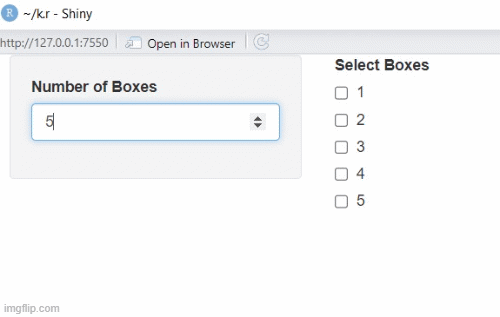
Dynamic checkboxGroupInput based on user input
Dynamic checkboxGroupInput Based on CSV File
Now let’s look at another example in which we will upload a .csv file and then checkboxes will be created based on the columns header which are there in the CSV file.
R
library (shiny)
library (readr)
ui <- fluidPage (
sidebarLayout (
sidebarPanel (
fileInput ( "file" , "Upload CSV file" )
),
mainPanel (
checkboxGroupInput ( "columns" , "Select Columns" )
)
)
)
server <- function (input, output, session) {
data <- reactive ({
req (input$file)
read_csv (input$file$datapath)
})
observe ({
columns <- names ( data ())
updateCheckboxGroupInput (session, "columns" ,
label = NULL ,
choices = columns,
selected = NULL )
})
}
shinyApp (ui, server)
|
Output:
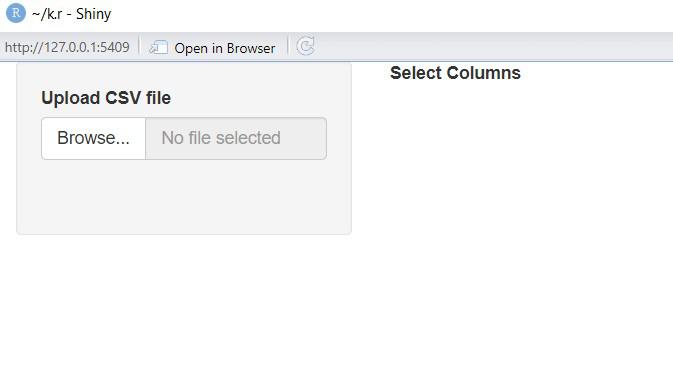
Dynamic checkboxGroupInput based on the CSV file
Here is another a bit simpler example based on the mtcars dataset in R.
R
library (shiny)
library (DT)
data <- mtcars
shinyApp (
ui = fluidPage (
selectInput ( "select1" , "select cyl:" ,
choices = unique (data$cyl)),
uiOutput ( "checkbox" ),
dataTableOutput ( "table" )
),
server = function (input, output) {
output$checkbox <- renderUI ({
choice <- unique (data[data$cyl % in % input$select1, "gear" ])
checkboxGroupInput ( "checkbox" , "Select gear" ,
choices = choice,
selected = choice[1])
})
}
)
|
Output:
Dynamic checkboxGroupInput can be useful in many situations where the user needs to select multiple options. For example, in a survey app, the user may want to select multiple options for a question. In a data analysis app, the user may want to select multiple variables to plot or analyze. Dynamic checkboxGroupInput allows for flexibility in the number and options presented to the user, making the app more user-friendly and efficient.
Share your thoughts in the comments
Please Login to comment...