PHP Program to Find Simple Interest
Last Updated :
27 Feb, 2024
Simple interest is the method to calculate the interest where we only take the principal amount each time without changing it for the interest earned in the previous cycle.
The formula to calculate Simple Interest is –
SI = P * R * T / 100
Where –
- I is the interest.
- P is the principal amount.
- R is the rate of interest per annum.
- T is the time in years.
In this article, we will explore how to calculate simple interest in PHP using different approaches.
Find Simple Interest using Inline Calculation
If you only need to perform the conversion once or in a specific context, you can do the calculation inline without defining a separate function.
Example: Illustration of program to find Simple Interest in PHP using inline calculations.
PHP
<?php
$principal = 1000;
$rate = 9;
$time = 5;
$interest = ( $principal * $rate * $time ) / 100;
echo "Simple Interest: $interest" ;
?>
|
Output
Simple Interest: 450
Time Complexity:Â O(1)
Auxiliary Space:Â O(1)
Find Simple Interest using a Function
Defining a function to calculate simple interest is a reusable and modular way to perform the calculation. The simpleInterest( )
function takes the principal, rate, and time as arguments and returns the simple interest.
Example: Illustration of program to find Simple Interest in PHP using a function.
PHP
<?php
function simpleInterest( $principal , $rate , $time ) {
$interest = ( $principal * $rate * $time ) / 100;
return $interest ;
}
$principal = 1000;
$rate = 9;
$time = 5;
$interest = simpleInterest( $principal , $rate , $time );
echo "Simple Interest: $interest" ;
?>
|
Output
Simple Interest: 450
Time Complexity:Â O(1)
Auxiliary Space:Â O(1)
In a more interactive scenario, you might want to calculate simple interest based on user input. This can be done using an HTML form to get the principal, rate and time as input and PHP to process the input.
Example: Illustration of program to find Simple Interest in PHP through User Input using HTML.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Simple Interest Calculator</ title >
</ head >
< body >
< form action = "" method = "post" >
< table >
< tr >
< td >< label for = "principal" >Principal:</ label ></ td >
< td >< input type = "text" id = "principal" name = "principal" ></ td >
</ tr >
< tr >
< td >< label for = "rate" >Rate (%):</ label ></ td >
< td >< input type = "text" id = "rate" name = "rate" ></ td >
</ tr >
< tr >
< td >< label for = "time" >Time (years):</ label ></ td >
< td >< input type = "text" id = "time" name = "time" ></ td >
</ tr >
< tr >
< td >< input type = "submit" value = "Calculate" ></ td >
</ tr >
</ table >
</ form >
<? php
if (isset($_POST['principal'])
&& isset($_POST['rate'])
&& isset($_POST['time'])) {
$principal = $_POST['principal'];
$rate = $_POST['rate'];
$time = $_POST['time'];
$interest = ($principal * $rate * $time) / 100;
echo "<p>Simple Interest: $interest</ p >";
}
?>
</ body >
</ html >
|
Output
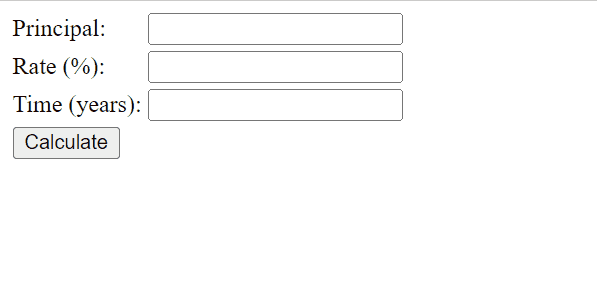
Time Complexity:Â O(1)
Auxiliary Space:Â O(1)
Share your thoughts in the comments
Please Login to comment...