Create a Password Strength Checker using HTML CSS and JavaScript
Last Updated :
05 Oct, 2023
This project aims to create a password strength checker using HTML, CSS, and JavaScript that is going to be responsible for checking the strength of a password for the user’s understanding of their password strength by considering the length of the password which will be that the password should contain at least 8 characters and the other is that the password should contain one uppercase letter, one lowercase letter, one number and one special character.
Prerequisites
Final Output
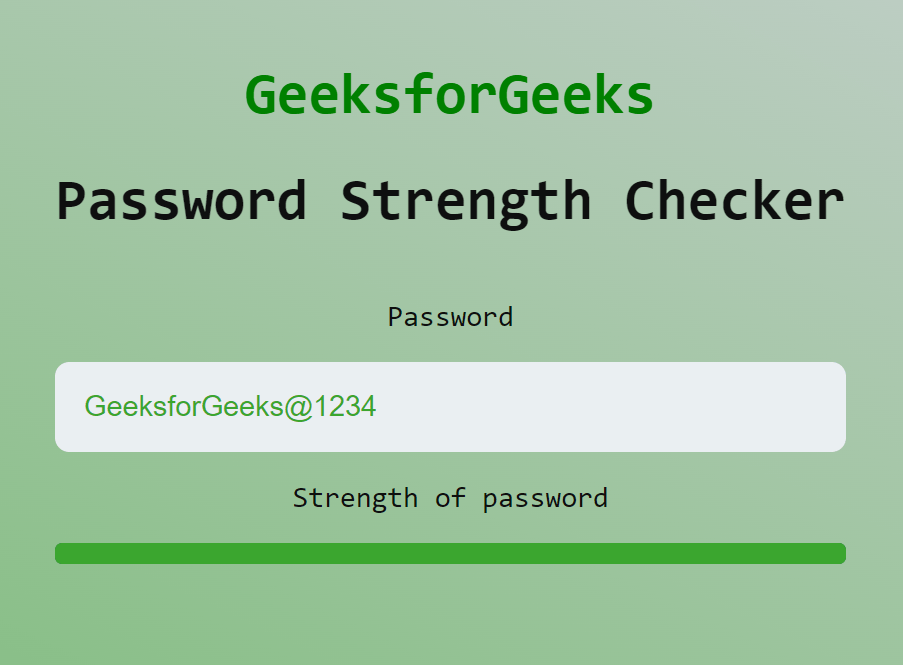
Final Output
Approach
The password strength checker will be implemented using the following steps:
- Create an HTML form that includes an input field for entering passwords using some tags that help to build the structure of the project <form>,<div>,<h>, etc.
- Styling the project using the CSS some properties that are used border, margin, padding, etc.
- Utilize JavaScript to analyze the characteristics of passwords and calculate their strengths.
- Display the assessed strength of passwords using indicators such as colors or progress bars.
- Provide users with feedback, about their password strength based on predefined criteria.
Project Structure
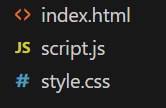
Project structure
Example: This example describes the basic implementation of the Password Strength Checker using HTML, CSS, and JavaScript.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0" />
< link rel = "stylesheet"
href = "style.css" />
< script src = "script.js" ></ script >
</ head >
< body >
< div class = "group" >
< h1 id = "top" >GeeksforGeeks</ h1 >
< h1 >Password Strength Checker</ h1 >
< label for = "" >Password</ label >
< input type = "text"
id = "password"
placeholder = "Type your password here" />
< label for = "" >
Strength of password
</ label >
< div class = "power-container" >
< div id = "power-point" ></ div >
</ div >
</ div >
</ body >
</ html >
|
CSS
body {
margin : 0 ;
font-family : monospace ;
min-height : 100 vh;
display : flex;
justify- content : center ;
align-items: center ;
background-image : linear-gradient(to top right ,
#7abb76 , #cfd2d8 );
color : #0e0f0f ;
font-size : 20px ;
}
# top {
color : green ;
}
.group {
width : auto ;
text-align : center ;
}
.group label {
display : block ;
padding : 20px 0 ;
}
.group input {
border : none ;
outline : none ;
padding : 20px ;
width : calc( 100% - 40px );
border-radius: 10px ;
background-color : #eaeff2 ;
color : #3ba62f ;
font-size : 20px ;
}
.group .power-container {
background-color : #2E424D ;
width : 100% ;
height : 15px ;
border-radius: 5px ;
}
.group .power-container #power-point {
background-color : #D73F40 ;
width : 1% ;
height : 100% ;
border-radius: 5px ;
transition: 0.5 s;
}
|
Javascript
let password = document.getElementById( "password" );
let power = document.getElementById( "power-point" );
password.oninput = function () {
let point = 0;
let value = password.value;
let widthPower =
[ "1%" , "25%" , "50%" , "75%" , "100%" ];
let colorPower =
[ "#D73F40" , "#DC6551" , "#F2B84F" , "#BDE952" , "#3ba62f" ];
if (value.length >= 6) {
let arrayTest =
[/[0-9]/, /[a-z]/, /[A-Z]/, /[^0-9a-zA-Z]/];
arrayTest.forEach((item) => {
if (item.test(value)) {
point += 1;
}
});
}
power.style.width = widthPower[point];
power.style.backgroundColor = colorPower[point];
};
|
Output:
Share your thoughts in the comments
Please Login to comment...