Create an Anagram Checker App using HTML CSS and JavaScript
Last Updated :
05 Oct, 2023
In this article, we will see how to create a web application that can verify if two input words are Anagrams or not, along with understanding the basic implementation through the illustration.
An Anagram refers to a word or phrase that’s created by rearranging the letters of another word or phrase using each letter once, i.e. An anagram of a string is another string that contains the same characters, only the order of characters can be different. For instance, all the characters of “silent” & “listen” are the same.
Prerequisites
Final Output:
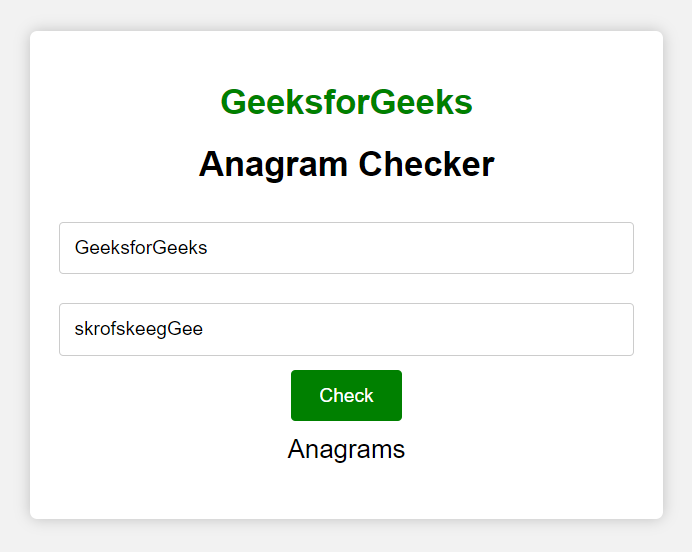
Preview of Final Output
Approach:
- Create a web page that includes two input fields where users can enter their words.
- Create an HTML file with input fields a “Check” button and a designated space, for displaying the result.
- Style the required HTML elements, in order to provide users with an interface and ensure they’re centered.
- After clicking on a “Check” button, the JavaScript code will determine whether the entered words are anagrams or not and accordingly will display the result on the page.
- Include the JavaScript function that will handle both anagram checking and input validation.
Example: This example describes the basic implementation of the Anagram Checker App using HTML, CSS, and JavaScript.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" />
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0" />
< link rel = "stylesheet"
href = "styles.css" />
< script src = "script.js" ></ script >
< title >Anagram Checker</ title >
</ head >
< body >
< div class = "container" >
< h1 id = "top" >GeeksforGeeks</ h1 >
< h1 >Anagram Checker</ h1 >
< input type = "text"
id = "word1"
placeholder = "Enter word 1" />
< input type = "text"
id = "word2"
placeholder = "Enter word 2" />
< button onclick = "checkAnagram()" >
Check
</ button >
< p id = "result" ></ p >
</ div >
</ body >
</ html >
|
CSS
body {
font-family : Arial , sans-serif ;
background-color : #f2f2f2 ;
margin : 0 ;
padding : 0 ;
display : flex;
justify- content : center ;
align-items: center ;
height : 100 vh;
}
# top {
color : green ;
}
.container {
text-align : center ;
background-color : white ;
padding : 20px ;
border-radius: 5px ;
box-shadow: 0 0 10px rgba( 0 , 0 , 0 , 0.2 );
max-width : 400px ;
margin : 0 auto ;
}
h 1 {
font-size : 24px ;
}
input {
width : 100% ;
padding : 10px ;
margin : 10px 0 ;
border : 1px solid #ccc ;
border-radius: 3px ;
box-sizing: border-box;
}
button {
background-color : green ;
color : white ;
border : none ;
padding : 10px 20px ;
border-radius: 3px ;
cursor : pointer ;
}
button:hover {
background-color : #43aa2e ;
}
p {
font-size : 18px ;
margin-top : 10px ;
}
|
Javascript
function checkAnagram() {
const word1 = document
.getElementById( "word1" )
.value.trim().toLowerCase()
.replace(/[^a-z]/g, "" );
const word2 = document
.getElementById( "word2" ).value.trim()
.toLowerCase().replace(/[^a-z]/g, "" );
if (word1 === "" || word2 === "" ) {
alert( "Please enter both words." );
return ;
}
if (word1.length !== word2.length) {
document.getElementById( "result" )
.textContent = "Not anagrams" ;
return ;
}
const sortedWord1 = word1.split( "" )
.sort().join( "" );
const sortedWord2 = word2.split( "" )
.sort().join( "" );
if (sortedWord1 === sortedWord2) {
document.getElementById( "result" )
.textContent = "Anagrams" ;
} else {
document.getElementById( "result" )
.textContent = "Not anagrams" ;
}
}
|
Output:
Share your thoughts in the comments
Please Login to comment...