Create a Basketball Ground Using Turtle Library in Python
Last Updated :
21 Mar, 2024
We have the task of how to make basketball ground using Turtle graphics in Python and print the ground. In this article, we will see how to create a basketball ground using Python Turtle Library.
Create a Basketball Ground Using Python Turtle
Below, is the Implementation of Basketball using the Turtle library in Python:
Step 1: Setting up the Environment
In the below code, the turtle module is imported, and a screen is created with a specified background color and size.
Python3
import turtle
screen = turtle.Screen()
screen.bgcolor("#eb8c34")
screen.setup(width=1357, height=760)
Step 2: Drawing the Background Rectangle
Below, code creare a turtle named ‘t’ and hides it, preparing it for drawing. It then moves the turtle backward by 500 units and shifts it 200 units to the left. After setting the pen size to 7 and showing the turtle, it fills a rectangle with a white outline and blue interior.
Python3
t = turtle.Turtle()
t.hideturtle()
t.penup()
t.backward(500)
t.left(90)
t.forward(200)
t.right(90)
t.pensize(7)
t.pendown()
t.pencolor("white")
t.fillcolor("blue")
t.begin_fill()
t.forward(1000)
t.right(90)
t.forward(500)
t.right(90)
t.forward(1000)
t.right(90)
t.forward(500)
t.end_fill()
Step 3: Drawing the Left Half of the Roof
Below, code lifts the pen, moves the turtle backward by 450 units, and turns it to the right by 90 degrees. Then, it places the pen down and draws a semicircle with a radius of 200 units. The turtle then turns left by 90 degrees and draws a red trapezoid.
Python3
t.penup()
t.backward(450)
t.right(90)
t.pendown()
t.circle(200, 180)
t.left(90)
t.forward(150)
t.left(90)
t.fillcolor("#ed2d05")
t.begin_fill()
t.forward(150)
t.circle(-40)
t.right(90)
t.forward(80)
t.right(90)
t.forward(150)
t.end_fill()
Step 4: Drawing the Chimney
Below, code lifts the pen, turns the turtle to the right by 90 degrees, and moves it forward by 70 units. Then, it turns the turtle to the right by 90 degrees again and moves it forward by 30 units. After placing the pen down, it turns the turtle to the right by 90 degrees and finally moves forward by another 30 units.
Python3
t.penup()
t.right(90)
t.forward(70)
t.right(90)
t.forward(30)
t.pendown()
t.right(90)
t.forward(30)
t.circle(10)
t.forward(30)
Step 5: Drawing the Right Half of the Roof
In below code lifts the pen, turns the turtle right by 90 degrees, and moves it 30 units forward. Then, it positions the turtle to draw a rectangle by moving it 271 units forward, then 500 units while turning right 90 degrees each time. After drawing a 500-unit line, it lifts the pen, moves the turtle left 90 degrees and forward 500 units.
Python3
t.penup()
t.right(90)
t.forward(30)
t.right(90)
t.forward(271)
t.right(90)
t.forward(500)
t.right(90)
t.pendown()
t.forward(500)
t.penup()
t.left(90)
t.forward(500)
t.left(90)
t.forward(50)
t.left(90)
t.pendown()
t.circle(-200, 180)
t.right(90)
t.forward(150)
t.right(90)
t.fillcolor("#ed2d05")
t.begin_fill()
t.forward(150)
t.circle(40)
t.left(90)
t.forward(80)
t.left(90)
t.forward(150)
t.end_fill()
Complete Code
Below, are the complete code of basketball ground which we created using Turtle Library in Python.
main.py
Python3
import turtle # importing turtle module
screen = turtle.Screen() # creating screen
screen.bgcolor("#eb8c34")
screen.setup(width=1357, height=760)
t = turtle.Turtle() # creating turtle object
t.hideturtle()
t.penup()
t.backward(500)
t.left(90)
t.forward(200)
t.right(90)
t.pensize(7)
t.pendown()
t.pencolor("white")
t.fillcolor("blue")
t.begin_fill()
t.forward(1000)
t.right(90)
t.forward(500)
t.right(90)
t.forward(1000)
t.right(90)
t.forward(500)
t.end_fill()
t.penup()
t.backward(450)
t.right(90)
t.pendown()
t.circle(200, 180)
t.left(90)
t.forward(150)
t.left(90)
t.fillcolor("#ed2d05")
t.begin_fill()
t.forward(150)
t.circle(-40)
t.right(90)
t.forward(80)
t.right(90)
t.forward(150)
t.end_fill()
t.penup()
t.right(90)
t.forward(70)
t.right(90)
t.forward(30)
t.pendown()
t.right(90)
t.forward(30)
t.circle(10)
t.forward(30)
t.penup()
t.right(90)
t.forward(30)
t.right(90)
t.forward(271)
t.right(90)
t.forward(500)
t.right(90)
t.pendown()
t.forward(500)
t.penup()
t.left(90)
t.forward(500)
t.left(90)
t.forward(50)
t.left(90)
t.pendown()
t.circle(-200, 180)
t.right(90)
t.forward(150)
t.right(90)
t.fillcolor("#ed2d05")
t.begin_fill()
t.forward(150)
t.circle(40)
t.left(90)
t.forward(80)
t.left(90)
t.forward(150)
t.end_fill()
t.penup()
t.left(90)
t.forward(70)
t.left(90)
t.forward(30)
t.pendown()
t.left(90)
t.forward(30)
t.circle(-10)
t.forward(30)
t.penup()
t.left(90)
t.forward(30)
t.left(90)
t.forward(270)
t.left(90)
t.forward(500)
t.left(90)
t.forward(350)
t.pendown()
t.left(90)
t.begin_fill()
t.circle(100, 180)
t.circle(100, 180)
t.end_fill()
t.penup()
t.goto(0, 200)
t.right(90)
t.pensize(10)
t.pendown()
t.forward(500)
turtle.done()
Run the Server
Run the following program by running the below command in your terminal:
python main.py
Output
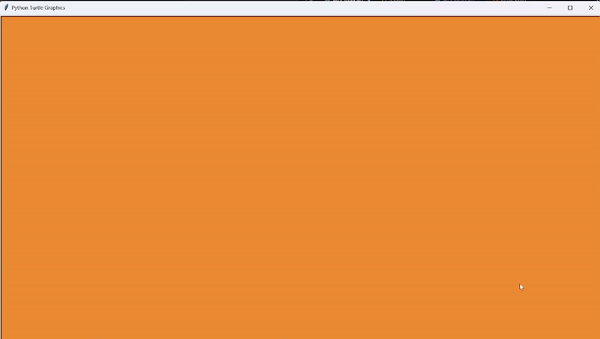
Share your thoughts in the comments
Please Login to comment...