Count the total number of triangles after Nth operation
Last Updated :
07 Dec, 2022
Given an equilateral triangle, the task is to compute the total number of triangles after performing the following operation N times.
For every operation, the uncolored triangles are taken and divided into 4 equal equilateral triangles. Every inverted triangle formed is colored. Refer to the below figure for more details.
For N=1 the triangle formed is:
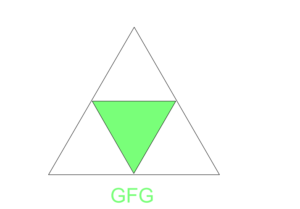
For N=2 the triangle formed is:
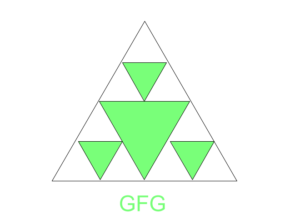
Examples:
Input :N = 10
Output : 118097
Input : N = 2
Output : 17
Approach:
- At every operation, 3 uncolored triangles, 1 colored triangle, and the triangle itself is formed
- On writing the above statement mathematically; count of triangles at Nth move = 3 * count of triangles at (N-1)th move + 2
- Therefore, initializing a variable curr = 1 and tri_count = 0
- Next, a loop is iterated from 1 to N
- For every iteration, the operation mentioned above is performed.
- Finally, the tri_count is returned
Below is the implementation of the above approach:
C++
#include <bits/stdc++.h>
using namespace std;
int CountTriangles( int n)
{
int curr = 1;
int Tri_count = 0;
for ( int i = 1; i <= n; i++) {
Tri_count = (curr * 3) + 2;
curr = Tri_count;
}
return Tri_count;
}
int main()
{
int n = 10;
cout << CountTriangles(n);
return 0;
}
|
Java
import java.io.*;
public class Gfg {
public static int CountTriangles( int n)
{
int curr = 1 ;
int Tri_count = 0 ;
for ( int i = 1 ; i <= n; i++) {
Tri_count = (curr * 3 ) + 2 ;
curr = Tri_count;
}
return Tri_count;
}
public static void main(String[] args)
{
int n = 10 ;
System.out.println(CountTriangles(n));
}
}
|
Python
def countTriangles(n):
curr = 1
Tri_count = 0
for i in range ( 1 , n + 1 ):
Tri_count = (curr * 3 ) + 2
curr = Tri_count
return Tri_count
n = 10
print (countTriangles(n))
|
C#
using System;
class Gfg
{
public static int CountTriangles( int n)
{
int curr = 1;
int Tri_count = 0;
for ( int i = 1; i <= n; i++)
{
Tri_count = (curr * 3) + 2;
curr = Tri_count;
}
return Tri_count;
}
public static void Main(String[] args)
{
int n = 10;
Console.WriteLine(CountTriangles(n));
}
}
|
Javascript
<script>
function CountTriangles(n)
{
var curr = 1;
var Tri_count = 0;
for (i = 1; i <= n; i++)
{
Tri_count = (curr * 3) + 2;
curr = Tri_count;
}
return Tri_count;
}
var n = 10;
document.write(CountTriangles(n));
</script>
|
Time Complexity: O(n)
Auxiliary Space: O(1)
Share your thoughts in the comments
Please Login to comment...