Count the number of circles at edges
Last Updated :
17 Feb, 2023
Given a line. At each level, that line can be divided into either two new lines or end up with a circle at its edge. An array X[] of size N is given to you, in which each X[i] denotes the number of circles at the ith level for (1? i ? N). Then you must determine whether X[] meets the above condition and return VALID or NOT VALID.
Examples:
Input: N = 4, X[] = {0, 0, 3, 2}
Output: VALID
Explanation:
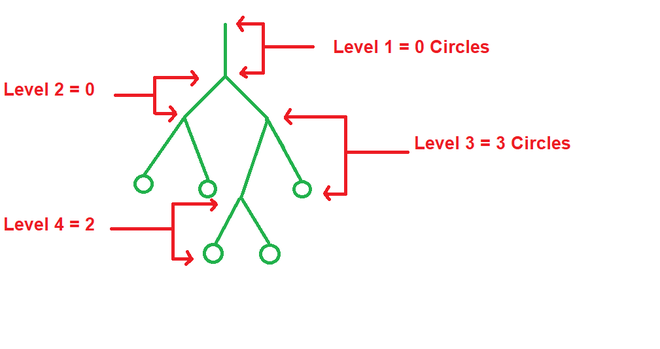
Example 1
- At Level 1 the line is divided into 2 new lines for Level 2. Therefore, the number of circles at Level 1 = 0.
- At Level 2 both the two lines were divided into two new lines to form a total of 4 lines for Level 3. Therefore, the number of circles at Level 2 = 0.
- At Level 3, 3 lines ended up with circles on its edge, and one line was divided into two new lines for Level 4. Therefore, the number of circles at Level 3 = 3.
- At Level 4, Both the lines ended up with circles on their edge. Therefore, the number of circles at Level 4 = 2.
The circles from levels 1 to 4 are 0, 0, 3 and 2 respectively, Which are the same as X[i] for (1 <= i <= N). Therefore, X[] is VALID.
Input: N = 3, X[] = {1, 2, 3}
Output: NOT VALID
Explanation: It can be verified that the given X[] is not valid according to the given condition in the statement.
Approach: Implement the idea below to solve the problem:
The problem is observation based and can be solved by using some mathematics.
Steps were taken to solve the problem:
- Create two variables ST and LE and initialize them equal to 1 and 0 respectively.
- Run a loop from i = 0 to less than N and follow the below-mentioned steps under the scope of the loop:
- If (ST < 0) return NOT VALID.
- If (X[i] == 0) ST *= 2
- else:
- If(X[i] > ST) then return NOT VALID
- ST -= X[i]
- ST *= 2
- If (ST != 0) then return NOT VALID else return VALID
Below is the code to implement the above approach:
C++
#include <iostream>
using namespace std;
string Is_Valid( int X[], int N)
{
int st = 1;
int le = 0;
for ( int i = 0; i < N; i++) {
if (st < 0)
return "NOT VALID" ;
if (X[i] == 0) {
st = st * 2;
}
else {
if (X[i] > st)
return "NOT VALID" ;
st -= X[i];
st = st * 2;
}
}
if (st != 0)
return "NOT VALID" ;
return "VALID" ;
}
int main()
{
int N = 5;
int X[] = { 0, 1, 0, 3, 2 };
cout << Is_Valid(X, N);
return 0;
}
|
Java
import java.io.*;
import java.lang.*;
import java.util.*;
class GFG {
public static String Is_Valid( int [] X, int N)
{
int st = 1 ;
int le = 0 ;
for ( int i = 0 ; i < N; i++) {
if (st < 0 )
return "NOT VALID" ;
if (X[i] == 0 ) {
st = st * 2 ;
}
else {
if (X[i] > st)
return "NOT VALID" ;
st -= X[i];
st = st * 2 ;
}
}
if (st != 0 )
return "NOT VALID" ;
return "VALID" ;
}
public static void main(String[] args)
throws java.lang.Exception
{
int N = 5 ;
int [] X = { 0 , 1 , 0 , 3 , 2 };
System.out.println(Is_Valid(X, N));
}
}
|
Javascript
function Is_Valid(X, N)
{
let st = 1;
let le = 0;
for (let i = 0; i < N; i++) {
if (st < 0)
return "NOT VALID" ;
if (X[i] == 0) {
st = st * 2;
}
else {
if (X[i] > st)
return "NOT VALID" ;
st -= X[i];
st = st * 2;
}
}
if (st != 0)
return "NOT VALID" ;
return "VALID" ;
}
let N = 5;
let X = [ 0, 1, 0, 3, 2 ];
console.log(Is_Valid(X, N));
|
Python3
import sys
class main( object ):
n = 3
X = [ 0 , 0 , 4 ]
nob = 1
found = True
i = 0
while i < n:
if X[i] > nob:
found = False
break
nob = nob - X[i]
nob = nob * 2
i + = 1
if found and nob = = 0 :
print ( "VALID" )
else :
print ( "NOT VALID" )
|
C#
using System;
class Program
{
static string Is_Valid( int [] X, int N)
{
int st = 1;
int le = 0;
for ( int i = 0; i < N; i++)
{
if (st < 0)
return "NOT VALID" ;
if (X[i] == 0)
{
st = st * 2;
}
else
{
if (X[i] > st)
return "NOT VALID" ;
st -= X[i];
st = st * 2;
}
}
if (st != 0)
return "NOT VALID" ;
return "VALID" ;
}
static void Main( string [] args)
{
int N = 5;
int [] X = { 0, 1, 0, 3, 2 };
Console.WriteLine(Is_Valid(X, N));
}
}
|
Time Complexity: O(N)
Auxiliary Space: O(1)
Share your thoughts in the comments
Please Login to comment...