Angular, is a robust front-end framework, which allows developers to build scalable and maintainable web applications. However, it provides extensive features and tools many times it happens that developers may encounter common pitfalls in their Angular journey.
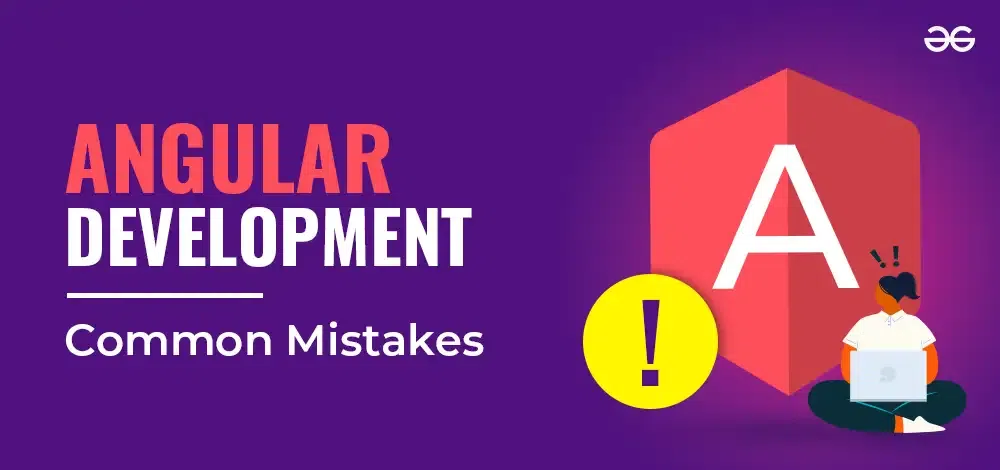
Today in this article we will look into the top 10 common mistakes that developers commit during Angular development.
What is Angular?
Angular is a widely used open-source front-end web application framework developed and maintained by Google. Angular is mainly designed to build dynamic, progressive, and single-page web applications easily. It follows component-based architecture, where applications are built as a tree of components. Angular offers comprehensive solutions for building modern web applications that provide developers structured framework, powerful features, and tools, that streamline the development process and enhance developer’s productivity.
Below are the Key Features of Angular
- Component-Based Architecture
- Two-Way data binding
- Dependency injection
- Routing
- Forms
- Testing support
10 Common Mistakes in Angular Development
These mistakes, if left unaddressed, can lead to issues such as unexpected behavior, memory leaks, and performance degradation. By understanding these common mistakes in Angular development and implementing best practices, developers can enhance their skills, improve application performance, and deliver high-quality, efficient web applications.
1. Misusing ngModel Two-Way binding:
ngModel Two-way binding is a very powerful feature in Angular that allows developers to synchronize the data between template and component classes. It enables developers for seamless interaction between user input in the template and data in component classes.
Using ‘ngModel’ for two-way data binding without considering its limits can result in unexpected behavior.
Example:
<input [(ngModel)]="employee.name">
While using ngModel with complex objects, Angular’s detection might not detect changes correctly which results in inconsistent UI.
Solution:
To avoid these inconsistencies developer should be binding directly to complex object properties, and bind to primitive type ‘ngModel’ directives for each properties
Example:
<input [(ngModel)]="employeeName">
<input [(ngModel)]="employeeEmail">
2. Ignoring RxJS Memory Management
The most common mistake that developers make while developing Angular applications is Ignoring RxJS memory management. RxJS is a powerful library for handling asynchronous operations, which includes HTTP requests and data streams. If subscriptions to observable are not managed properly they can result in memory leaks and application crashes.
Issues Occur Due to Improper RxJS Memory Management
- When observers are subscribed but not unsubscribe after use they continue to hold reference which results in memory leaks.
- Memory leaks can degrade the performance of an application over time, as the browser’s memory usage increases.
- Memory leaks can lead to application crashes, especially in long-running applications.
Solution to RxJS Memory Management
- Unsubscribe the observables when not in use
- Use TakeUntil Operators
- Clean up ngOnDestroy
- Avoid implicit Subscriptions
3. Overusing Angular Directives
Angular directives such as ngIf, ngFor, and ngSwitch are powerful features used for manipulating the DOM and rendering the content based on data and conditions. However excessive use of these directives such as nested data structures can result in slow-running applications and other performance issues in applications.
Below is a List of How it Can Impact Angular Applications.
- Performance degradation
- Template complexity
- Code readability
Solution that Developers Can Adopt to Avoid the Above Impacts
- Component composition
- Container components
- Directives optimization
- Proper code review and code refactoring
4. Not leveraging Angular’s DI(Dependency Injection)
Not leveraging Angular’s DI(Dependency Injection) system is a common mistake that developers make while developing Angular applications. In Angular Dependency Injection is a core feature that allows components, services, and other classes to declare dependencies and inject when needed. Neglect using of Dependency Injection system may result in tightly coupled code which may be difficult to maintain when code becomes complex. Failing to use Dependency Injections in code can result in tightly coupled code that becomes hard to test and maintain.
Below are the Impacts of Not Using Dependency Injection in Angular Applications.
- Neglecting DI use can lead to classes being tightly coupled to their dependency.
- Without DI use developers may manually create the same instance at multiple places which results in code duplication
- Without DI it’s always challenging to replace real dependencies with mock object for unit testing, which results in complex test case writing.
Let’s Look into a Solution to Not Using Dependency Injection in Angular Applications
- Declare a dependency on construction
- Use of provider while registering a dependency
- Leverage Hierarchical Injectors
- Mock Dependency for Testing
5. Overusing Overridden Method ‘ngOnChanges’
While developing Angular applications developers overuse the method ‘ngOnChanges’. The method ndOnChanges gets invoked by the Angular system whenever one or more data-bound input properties of the component get changed. Though ngOnChanges is a very powerful method that developers use frequently while coding overuse of it can result in performance issues, code complexity, and reduced code maintenance.
For instance, using ngOnChanges for complex object or array changes can lead to performance issues.
Below are the Impacts of Overusing bgOnChanges
- Application Code complexity
- Reduced maintainability
- Application performance issues
Best Practices to Avoid Pitfalls Associated with Overuse of ‘ngOnChanges’
- Use ngOnChange when needed only
- Breakdown the complex login into smaller modules
- Minimize the performance impact of ngOnChange’
- When possible use an immutable data structure
6. Neglecting Lazy Loading
Neglecting Lazy loading is also a common mistake that developers make in Angular project implementation. The lazy loading technique is used to show the loading of certain parts of the application until data is received. Avoiding the use of lazy loading can result in slower application startup times, long initial loading times, and larger bundle sizes, ultimately poor user experience.
Below are the Impacts of Neglecting Lazy Loading
- Without lay loading, the application takes a longer startup time as the browser needs to download and parse JS and CSS files.
- Neglecting lazy loading can result in a larger bundle size, all modules and dependencies are bundled into a single file.
- Slow application startup time and initial loading time result in poor user experience.
Solutions to Neglecting Lazy Loading
- Divide application code into feature modules based on functionality. Use Lazy loading to load this module asynchronously.
- Use performance monitoring tool to measure lazy loading impact on initial and startup time
- Use tools like Angular CLI’s production build optimization and tree shaking to remove unused code and reduce bundle size
7. Tight Coupling with Angular Material
Angular material is the most commonly used and popular UI component library for Angular. It provides pre-built components and styles to create responsive user interfaces. However, tightly coupling components with Angular material can result in several drawbacks, including increased dependencies, and less flexibility, and makes it difficult to migrate to other UI libraries and themes.
Below are the Impacts of Tight Coupling with Angular Material
- Tight coupling components with Angular material makes it more difficult to customize UI which results in reduced flexibility.
- The use of Angular material introduces additional dependencies in the project, resulting in increased dependencies and project bundle size.
- This tight coupling also can result in Vendors in, making it difficult to migrate to another UI library.
Solution to Tight Coupling with Angular Material
- Abstract Angular Material component behind custom wrapper to decouple them from application’s business logic, makes the customization easy.
- Adopting a design pattern such as an Adapter or Facade can help to switch to another implementation in the future.
- Maintaining separate concerns can help in making testing easy.
8. Not Optimizing Change Detection
Angular’s change detection is a very powerful and commonly used mechanism by developers but it can become a bottleneck if not used efficiently. Fail to optimize in use of these change detections can result in decreased application performance and introduced long rendering UI components and higher resource utilization which can also result in poor application UI.
Below are the Impacts of Not Optimizing Change Detection
- Angular’s change detection strategy traverses the entire component tree on each detection cycle, which results in performance overhead.
- If change detection is not used efficiently then it can lead to unnecessary re-rendering of components in DOM elements, even no change in actual data.
- Inefficient change detection can consume more memory and CPU, mainly in devices with less memory and power processing.
Solution to Not Optimizing Change Detection
- Use change detection strategy ChangeDetectionStrategy.OnPush whenever possible, instructs Angular to only run change detection for components on input property change.
- Be careful while using observable patterns in Angular. Excessive use of it can lead to unnecessary change detection cycles.
- Review component tree structure and refactor it to minimum depth and complexity of hierarchy.
9. Neglecting Testing
Testing is a crucial part of software development. It helps developers ensure the reliability, stability, and maintainability of applications. Failing to implement thorough testing in of application can result in higher bug counts, lower code quality, and higher technical debt in applications. It can also result in the loss of the Application’s active users.
Below are the Impacts of Not Optimizing Change Detection:
- Without proper testing bugs and errors are most likely to go unnoticed, which results in higher bug counts.
- Untested code may lack proper error handling and edge case coverage makes it difficult to maintain code
- Lack of testing can also contribute to increased technical debt and untested code.
The Solution to Neglecting Testing:
- Have a practice of writing unit test cases for individual components and services to identify bugs in advance.
- Adopt TDD(Test-driven development), which helps in driving the design of code and encourages better test coverage.
- Set up automation testing by using tools like Karma, Jasmine, and Protractor to make the testing approach automatic.
10. Poor Component Architecture
Component architecture refers to the structure and organization of Angular components within in project. The inefficient and poor design of this architecture can result in code duplication, tight code coupling, and difficulty in managing and maintaining the applications. Poor project architecture also makes it difficult to manage complex projects in Angular.
Below are the Impacts of Poor Component Architecture
- Without proper design of components, architecture may duplicate code across multiple components which results in redundancy and increases maintenance overhead.
- The poorly structured architecture of components may become tightly coupled, making it difficult to modify or extend without affecting another part of the application.
- As the application grows in size poor component design may impede scalability to add new features.
Solution to Poor Component Architecture
- Adopting a single responsibility principle where each component has its own responsibility and purpose. This prompts code modularity, reusability, and maintainability.
- Use Angular service to encapsulate shared business logic and data manipulation, it facilitates code reuse and testability.
- Use of hierarchical component structure and Encapsulate UI logic.
Must Read:
Conclusion
By following best practices of code standards and avoiding code developers can build more robust, efficient, and easy maintainable Angular applications. Adopting the solution defined above in the article can help developers avoid these 10 Common Mistakes in Angular Development and build high-quality and performance Angular applications. Continued learning, code review, and following design patterns are essential for successful Angular development.
Â
Share your thoughts in the comments
Please Login to comment...