Checking if a figure is empty using Matplotlib
Last Updated :
06 Dec, 2023
In this article, we will explore how to identify and handle empty plots in Matplotlib. While Matplotlib enables us to create various graphs and charts, it’s important to address situations where the generated plots might turn out to be empty. These empty plots could potentially introduce risks when included in a report. Therefore, in this article, we will learn how to detect and manage empty plots in Matplotlib.
Here’s how you can install Matplotlib:
pip install matplotlib
Running the above command will download and install Matplotlib, for making it available . This library is indispensable for creating a wide range of data visualizations, from basic line plots to complex 3D visualizations, making it a valuable tool for data scientists, engineers, and researchers.
How to Check for an Empty Plot ?
To determine if a plot is empty or not, we will utilize the get_children()
method. This method allows us to retrieve the children of the figure, and if their count is less than or equal to 1, then the plot is considered empty.
Syntax of is_empty()
This code defines a function, is_empty(figure)
, which takes a Matplotlib figure as input, retrieves the contained artists using get_children()
, and checks if the count of these artists is less than or equal to
1. If this condition is met, the plot is classified as empty.
def is_empty(figure):
contained_artists = figure.get_children()
return len(contained_artists) <= 1
Example 1: Not Empty Plot
In below code the Function is_empty
(figure)
that checks if a Matplotlib figure is empty or not. It does so by counting the number of contained artists within the figure using get_children
()
. If the count is less than or equal to 1, the plot is considered empty.
The code then proceeds to create a simple bar chart using Matplotlib, and after that, it calls the is_empty
function with the figure object as an argument. Depending on whether the plot is empty or not, it prints the corresponding message.
Python3
import matplotlib.pyplot as plt
def is_empty(figure):
contained_artists = figure.get_children()
return len (contained_artists) < = 1
x = [ 1 , 2 , 3 , 4 , 5 ]
y = [ 10 , 20 , 30 , 40 , 50 ]
fig = plt.figure()
plt.bar(x, y)
if is_empty(fig):
print ( 'Plot is Empty' )
else :
print ( 'Plot is not empty' )
|
Output:
Plot is not empty
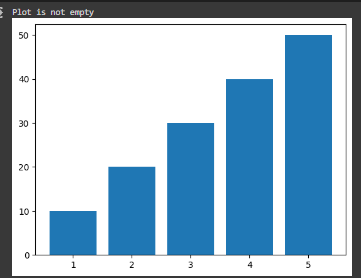
Plot is create means not empty
Example 2: Empty Plot
Function is_empty
(figure)
that checks if a Matplotlib figure is empty by counting its contained artists, where a count of one or fewer signifies an empty figure. It then creates a Matplotlib figure, calls the is_empty
function to determine if the figure is empty, and prints either ‘Plot is Empty’ or ‘Plot is not empty’ based on the result.
Python3
import matplotlib.pyplot as plt
def is_empty(figure):
contained_artists = figure.get_children()
return len (contained_artists) < = 1
fig = plt.figure()
if is_empty(fig):
print ( 'Plot is Empty' )
else :
print ( 'Plot is not empty' )
|
Output:
Plot is Empty
In summary, Matplotlib, a versatile Python library for data visualization, provides us with the tools needed to create a wide range of plots and charts. However, in situations where data may result in an empty plot, it becomes crucial to efficiently verify the plot’s content. Using the get_children()
method and a simple function like is_empty
()
, we can quickly assess whether a generated plot contains meaningful data or not. This capability enhances the reliability and usability of Matplotlib in producing accurate and informative visualizations for data analysis and presentation.
Share your thoughts in the comments
Please Login to comment...