Check if N can be represented as sum of squares of two consecutive integers
Last Updated :
20 Sep, 2023
Given an integer N, the task is to check whether N can be represented as a sum of squares of two consecutive integers or not.
Examples:
Input: N = 5
Output: Yes
Explanation:
The integer 5 = 12 + 22 where 1 and 2 are consecutive numbers.
Input: 13
Output: Yes
Explanation:
13 = 22 + 32
Approach: This equation can be represented as:
=> 
=> 
=> 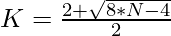
Finally, check the value of computed using this formula is an integer, which means that N can be represented as the sum of squares 2 consecutive integers
Below is the implementation of the above approach:
C++
#include <bits/stdc++.h>
using namespace std;
bool isSumSquare( int N)
{
float n
= (2 + sqrt (8 * N - 4))
/ 2;
return (n - ( int )n) == 0;
}
int main()
{
int i = 13;
if (isSumSquare(i)) {
cout << "Yes" ;
}
else {
cout << "No" ;
}
return 0;
}
|
Java
import java.lang.Math;
class GFG{
public static boolean isSumSquare( int N)
{
double n = ( 2 + Math.sqrt( 8 * N - 4 )) / 2 ;
return (n - ( int )n) == 0 ;
}
public static void main(String[] args)
{
int i = 13 ;
if (isSumSquare(i))
{
System.out.println( "Yes" );
}
else
{
System.out.println( "No" );
}
}
}
|
Python3
import math
def isSumSquare(N):
n = ( 2 + math.sqrt( 8 * N - 4 )) / 2
return (n - int (n)) = = 0
if __name__ = = '__main__' :
i = 13
if isSumSquare(i):
print ( 'Yes' )
else :
print ( 'No' )
|
C#
using System;
class GFG{
public static bool isSumSquare( int N)
{
double n = (2 + Math.Sqrt(8 * N - 4)) / 2;
return (n - ( int )n) == 0;
}
public static void Main(String[] args)
{
int i = 13;
if (isSumSquare(i))
{
Console.WriteLine( "Yes" );
}
else
{
Console.WriteLine( "No" );
}
}
}
|
Javascript
<script>
function isSumSquare(N)
{
var n = (2 + Math.sqrt(8 * N - 4)) / 2;
return (n - parseInt( n)) == 0;
}
var i = 13;
if (isSumSquare(i))
{
document.write( "Yes" );
}
else
{
document.write( "No" );
}
</script>
|
Note: In order to print the integers, we can easily solve the above equation to get the roots.
Time Complexity: O(logN) because it is using inbuilt sqrt function
Auxiliary Space: O(1)
Approach 2: Iterative Method:
Here’s another approach to check whether a number is the sum of squares of two consecutive numbers or not:
- Iterate through all possible pairs of consecutive integers (x, x+1) such that x is less than the square root of N.
- For each pair (x, x+1), compute their sum of squares as x^2 + (x+1)^2.
- If the sum of squares is equal to N, return true. Otherwise, continue iterating through the pairs of consecutive integers.
- If no pair of consecutive integers satisfies the condition, return false.
Here’s the implementation of this approach in C++:
C++
#include <bits/stdc++.h>
using namespace std;
bool isSumSquare( int N) {
for ( int x = 0; x * x < N; x++) {
int sum = x * x + (x + 1) * (x + 1);
if (sum == N) {
return true ;
}
}
return false ;
}
int main() {
int N = 13;
if (isSumSquare(N)) {
cout << "Yes" ;
} else {
cout << "No" ;
}
return 0;
}
|
Java
public class Main {
static boolean isSumSquare( int N) {
for ( int x = 0 ; x * x < N; x++) {
int sum = x * x + (x + 1 ) * (x + 1 );
if (sum == N) {
return true ;
}
}
return false ;
}
public static void main(String[] args) {
int N = 13 ;
if (isSumSquare(N)) {
System.out.println( "Yes" );
} else {
System.out.println( "No" );
}
}
}
|
Python3
def isSumSquare(N):
for x in range ( 0 , N):
sum = x * x + (x + 1 ) * (x + 1 )
if sum = = N:
return True
elif sum > N:
return False
return False
N = 13
if isSumSquare(N):
print ( "Yes" )
else :
print ( "No" )
|
C#
using System;
public class Program {
public static bool IsSumSquare( int N)
{
for ( int x = 0; x * x < N; x++) {
int sum = x * x + (x + 1) * (x + 1);
if (sum == N) {
return true ;
}
}
return false ;
}
public static void Main()
{
int N = 13;
if (IsSumSquare(N)) {
Console.WriteLine( "Yes" );
}
else {
Console.WriteLine( "No" );
}
}
}
|
Javascript
function isSumSquare(N) {
for (let x = 0; x * x < N; x++) {
let sum = x * x + (x + 1) * (x + 1);
if (sum === N) {
return true ;
}
}
return false ;
}
let N = 13;
if (isSumSquare(N)) {
console.log( "Yes" );
} else {
console.log( "No" );
}
|
Output:
Yes
Time Complexity: O(N)
Auxiliary Space: O(1)
Share your thoughts in the comments
Please Login to comment...