Check if a node is an Internal Node or not
Last Updated :
28 Nov, 2023
Given a binary tree, for each node check whether it is an internal node or not.
Examples:
Input:
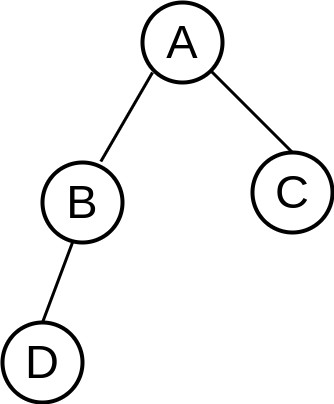
Example-1
Output: Node A: True
Node B: True
Node C: False
Node D: False
Explanation: In this illustration, Node A possesses two child nodes (B and C) which categorizes it as a node. On the other hand, both Nodes B possess one child node D, and C is a leaf node since they lack any child nodes.
Input:
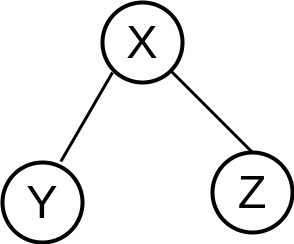
Example-2
Output: Node X: True
Node Y: False
Node Z: False
Explanation: In this illustration, Node X possesses two child nodes (Y and Z) which categorizes it as a node. On the other hand, both Nodes Y and Z are leaf nodes since they lack any child nodes.
Approach: To solve the problem follow the below steps:
- Traverse the tree starting from the root node.
- For every encountered node, during traversal check if it has any child nodes or not.
- If the node has at least one child classify it as an internal node; otherwise consider it a leaf node.
- Check for at least one child if either left or right or both exist then the node is an internal node.
Below is the implementation of the above approach:
C++
#include <iostream>
#include <vector>
class TreeNode {
public :
std::string value;
std::vector<TreeNode*> childNodes;
TreeNode(std::string val) {
value = val;
}
};
bool checkNode(TreeNode* currNode) {
return currNode->childNodes.size() > 0;
}
int main() {
TreeNode* root = new TreeNode( "A" );
root->childNodes.push_back( new TreeNode( "B" ));
root->childNodes.push_back( new TreeNode( "C" ));
root->childNodes[0]->childNodes.push_back( new TreeNode( "D" ));
std::cout << checkNode(root) << std::endl;
std::cout << checkNode(root->childNodes[0]) << std::endl;
std::cout << checkNode(root->childNodes[1]) << std::endl;
std::cout << checkNode(root->childNodes[0]->childNodes[0]) << std::endl;
return 0;
}
|
Java
import java.util.ArrayList;
import java.util.List;
class TreeNode {
String value;
List<TreeNode> childNodes;
public TreeNode(String val) {
value = val;
childNodes = new ArrayList<>();
}
}
public class Main {
public static boolean checkNode(TreeNode currNode) {
return currNode.childNodes.size() > 0 ;
}
public static void main(String[] args) {
TreeNode root = new TreeNode( "A" );
root.childNodes.add( new TreeNode( "B" ));
root.childNodes.add( new TreeNode( "C" ));
root.childNodes.get( 0 ).childNodes.add( new TreeNode( "D" ));
System.out.println(checkNode(root));
System.out.println(checkNode(root.childNodes.get( 0 )));
System.out.println(checkNode(root.childNodes.get( 1 )));
System.out.println(checkNode(root.childNodes.get( 0 ).childNodes.get( 0 )));
}
}
|
Python
class tree:
def __init__( self , val):
self .value = val
self .childnode = []
def checkNode(currNode):
return len (currNode.childnode) > 0
root = tree("A")
root.childnode.append(tree("B"))
root.childnode.append(tree("C"))
root.childnode[ 0 ].childnode.append(tree("D"))
print (checkNode(root))
print (checkNode(root.childnode[ 0 ]))
print (checkNode(root.childnode[ 1 ]))
print (checkNode(root.childnode[ 0 ].childnode[ 0 ]))
|
C#
using System;
using System.Collections.Generic;
class TreeNode
{
public string Value { get ; set ; }
public List<TreeNode> ChildNodes { get ; }
public TreeNode( string val)
{
Value = val;
ChildNodes = new List<TreeNode>();
}
}
class MainClass
{
static bool CheckNode(TreeNode currNode)
{
return currNode.ChildNodes.Count > 0;
}
public static void Main( string [] args)
{
TreeNode root = new TreeNode( "A" );
root.ChildNodes.Add( new TreeNode( "B" ));
root.ChildNodes.Add( new TreeNode( "C" ));
root.ChildNodes[0].ChildNodes.Add( new TreeNode( "D" ));
Console.WriteLine(CheckNode(root));
Console.WriteLine(CheckNode(root.ChildNodes[0]));
Console.WriteLine(CheckNode(root.ChildNodes[1]));
Console.WriteLine(CheckNode(root.ChildNodes[0].ChildNodes[0]));
}
}
|
Javascript
class TreeNode {
constructor(val) {
this .value = val;
this .childNodes = [];
}
}
function checkNode(currNode) {
return currNode.childNodes.length > 0;
}
const root = new TreeNode( "A" );
root.childNodes.push( new TreeNode( "B" ));
root.childNodes.push( new TreeNode( "C" ));
root.childNodes[0].childNodes.push( new TreeNode( "D" ));
console.log(checkNode(root));
console.log(checkNode(root.childNodes[0]));
console.log(checkNode(root.childNodes[1]));
console.log(checkNode(root.childNodes[0].childNodes[0]));
|
Output
True
True
False
False
Time Complexity: O(N), where N is the number of nodes in the tree.
Auxillary Space: O(H), where H is the height of the tree (due to the recursion stack).
Share your thoughts in the comments
Please Login to comment...