C# | Jump Statements (Break, Continue, Goto, Return and Throw)
Last Updated :
15 Feb, 2023
In C#, Jump statements are used to transfer control from one point to another point in the program due to some specified code while executing the program. There are five keywords in the Jump Statements:
- break
- continue
- goto
- return
- throw
break statement
The break statement is used to terminate the loop or statement in which it present. After that, the control will pass to the statements that present after the break statement, if available. If the break statement present in the nested loop, then it terminates only those loops which contains break statement.
Flowchart:
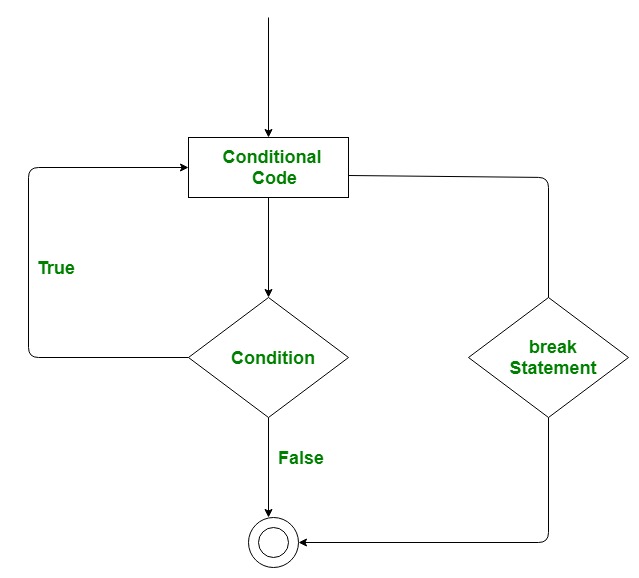
Example:
CSharp
using System;
class Geeks {
static public void Main()
{
for ( int i = 1; i < 4; i++)
{
if (i == 3)
break ;
Console.WriteLine("GeeksforGeeks");
}
}
}
|
Output:
GeeksforGeeks
GeeksforGeeks
continue statement
This statement is used to skip over the execution part of the loop on a certain condition. After that, it transfers the control to the beginning of the loop. Basically, it skips its following statements and continues with the next iteration of the loop.
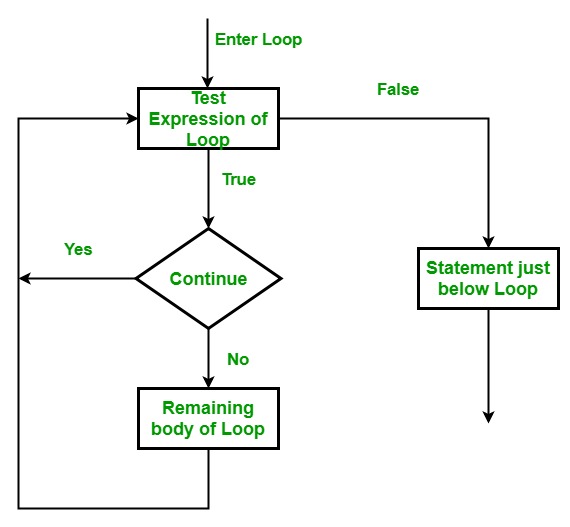
Example:
CSharp
using System;
class Geeks {
public static void Main()
{
for ( int i = 1; i <= 10; i++) {
if (i == 4)
continue ;
Console.WriteLine(i);
}
}
}
|
Output:
1
2
3
5
6
7
8
9
10
goto statement
This statement is used to transfer control to the labeled statement in the program. The label is the valid identifier and placed just before the statement from where the control is transferred.
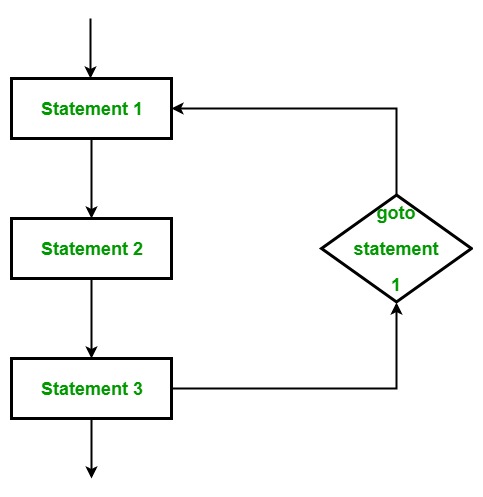
Example:
CSharp
using System;
class Geeks {
static public void Main()
{
int number = 20;
switch (number) {
case 5:
Console.WriteLine(" case 5");
break ;
case 10:
Console.WriteLine(" case 10");
break ;
case 20:
Console.WriteLine(" case 20");
goto case 5;
default :
Console.WriteLine("No match found");
}
}
}
|
return statement
This statement terminates the execution of the method and returns the control to the calling method. It returns an optional value. If the type of method is void, then the return statement can be excluded.
Example:
CSharp
using System;
class Geeks {
static int Addition( int a)
{
int add = a + a;
return add;
}
static public void Main()
{
int number = 2;
int result = Addition(number);
Console.WriteLine("The addition is {0}", result);
}
}
|
Output:
The addition is 4
throw statement
This is used to create an object of any valid exception class with the help of new keyword manually. The valid exception must be derived from the Exception class.
Example:
CSharp
using System;
class Geeks {
static string sub = null ;
static void displaysubject( string sub1)
{
if (sub1 == null )
throw new NullReferenceException("Exception Message");
}
static void Main( string [] args)
{
try
{
displaysubject(sub);
}
catch (Exception exp)
{
Console.WriteLine(exp.Message );
}
}
}
|
Output:
Exception Message
Share your thoughts in the comments
Please Login to comment...