Build a Markdown Previewer
Last Updated :
15 Apr, 2024
The Markdown Previewer is a simple web application that allows users to input Markdown syntax and preview the rendered HTML output in real-time. This article will cover the implementation of a Markdown Previewer using HTML, CSS, and JavaScript.Markdown is a lightweight markup language that is commonly used for formatting text on the web.
- Markdown is a lightweight markup language with plain-text formatting syntax. Common Markdown syntax includes headings, links, inline code, code blocks, lists, blockquotes, images, and emphasis (bold and italic text).
- The Markdown syntax consists of simple formatting rules such as using # for headers, * or _ for italics, ** or __ for bold, and so on.
- # Hello, Markdown!’
In this approach, you directly manipulate the DOM elements using JavaScript to capture user input and update the preview container. This approach is suitable for small-scale applications and provides more control over the implementation details.
Explanation:
The provided code demonstrates a basic Markdown Previewer implemented using HTML, CSS, and JavaScript. The HTML file contains text for inputting Markdown syntax and a div element to display the rendered HTML content. JavaScript code listens for input events on the text, converts the Markdown to HTML using the marked library, and updates the preview div accordingly.
Example: Below is an example of building a Markdown Previewer.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>Markdown Previewer</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="container">
<div class="editor-container">
<textarea id="editor"></textarea>
</div>
<div class="preview-container">
<div id="preview"></div>
</div>
</div>
<script src=
"https://cdnjs.cloudflare.com/ajax/libs/marked/2.0.2/marked.min.js"></script>
<script src="script.js"></script>
</body>
</html>
CSS
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
}
.container {
display: flex;
justify-content: space-around;
align-items: flex-start;
margin-top: 20px;
}
.editor-container,
.preview-container {
width: 45%;
}
textarea {
width: 100%;
height: 300px;
border: 1px solid #ccc;
padding: 10px;
box-sizing: border-box;
}
#preview {
border: 1px solid #ccc;
padding: 10px;
min-height: 300px;
}
Javascript
document.addEventListener("DOMContentLoaded", () => {
const editor = document.getElementById("editor");
const preview = document.getElementById("preview");
function updatePreview() {
const markdown = editor.value;
const html = marked(markdown, { breaks: true });
preview.innerHTML = html;
}
editor.addEventListener("input", updatePreview);
// Initial load
const defaultMarkdown = `
# Heading 1
## Heading 2
[Link](https://www.example.com)
\`inline code\`
\`\`\`
// Code block
const greeting = 'Hello';
console.log(greeting);
\`\`\`
- List item 1
- List item 2
> Blockquote
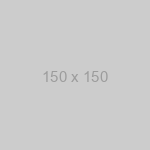
**Bold text**
`;
editor.value = defaultMarkdown;
updatePreview();
});
Output:
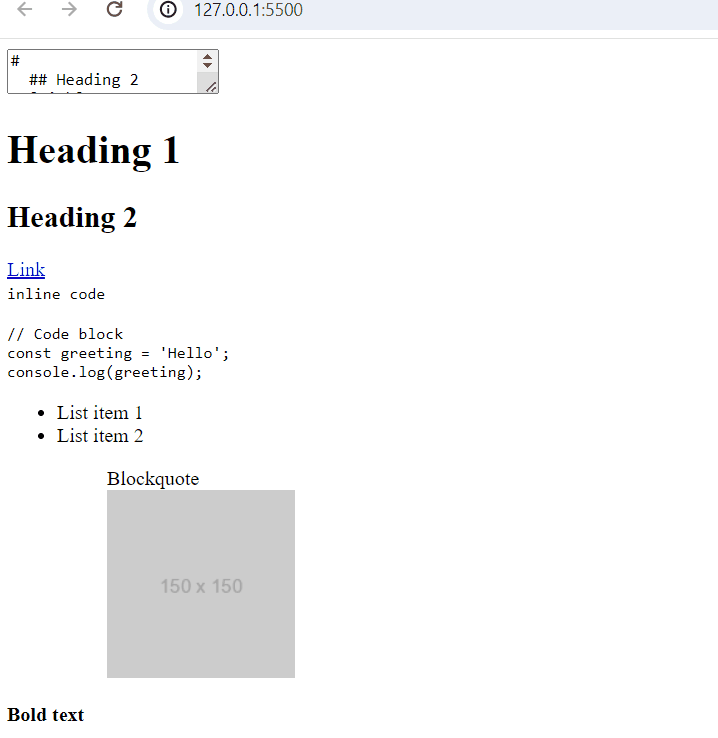
Build a Markdown Previewer
Approach 2: Framework/Library Integration (e.g., React):
Using a framework like React simplifies the development process by providing reusable components, state management, and a virtual DOM for efficient rendering. You can create separate components for the input text and preview container, making the code more modular and maintainable.
Steps to Build a MarkDown Previewer using ReactJS:
Step 1: Create a React app using the following command.
npx create-react-app markdown-previewer
cd markdown-previewer
Step 2: Install the react-markdown library to parse and render Markdown content.
npm install react-markdown
Step 3: Create two components: Editor for inputting Markdown content and Previewer for displaying the parsed Markdown.
Example: Below is an example of MarkDown Previewer using ReactJS.
CSS
.app {
display: flex;
justify-content: space-between;
align-items: flex-start;
}
textarea {
width: 45%;
height: 80vh;
padding: 10px;
font-size: 16px;
border: 1px solid #ccc;
}
.preview {
width: 45%;
height: 80vh;
padding: 10px;
border: 1px solid #ccc;
overflow-y: auto;
}
JavaScript
// Create the main App component to render
// both the Editor and Previewer components.
// App.js
import React, { useState } from 'react';
import Editor from './Editor';
import Previewer from './Previewer';
import './App.css';
function App() {
const [markdown, setMarkdown] = useState('');
return (
<div className="app">
<Editor markdown={markdown} setMarkdown={setMarkdown} />
<Previewer markdown={markdown} />
</div>
);
}
export default App;
JavaScript
//Editor.js
import React from 'react';
function Editor({ markdown, setMarkdown }) {
return (
<textarea
value={markdown}
onChange={(e) => setMarkdown(e.target.value)}
placeholder="Enter Markdown here..."
/>
);
}
export default Editor;
JavaScript
// Previewer.js
import React from 'react';
import ReactMarkdown from 'react-markdown';
function Previewer({ markdown }) {
return (
<div>
<h2>Preview</h2>
<div className="preview">
<ReactMarkdown>{markdown}</ReactMarkdown>
</div>
</div>
);
}
export default Previewer;
Start your app using the following command:
npm start
Output:
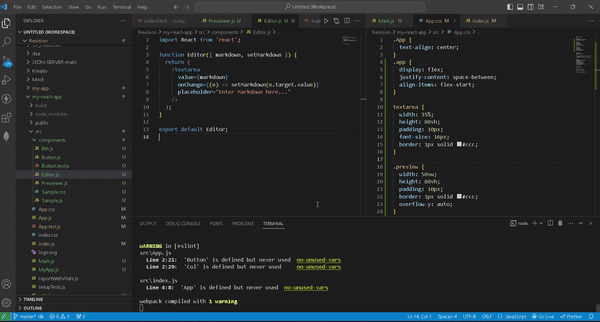
Markdown Previewer Using React
Share your thoughts in the comments
Please Login to comment...