Angular PrimeNG Skeleton DataTable
Last Updated :
03 Oct, 2022
Angular PrimeNG is an open-source front-end UI library that has many native Angular UI components which help developers to build a fast and scalable web solution. In this article, we will be seeing Angular PrimeNG Skeleton DataTable.
The Skeleton Component is used as a placeholder while the data for the actual component is loading in the background. To show a skeleton data table we can replace the column data with the p-skeleton component in the body template of the table.
Syntax:
<p-table [value]="..." responsiveLayout="scroll">
<ng-template pTemplate="header">
<tr>
....
</tr>
</ng-template>
<ng-template pTemplate="body" let-x>
<tr>
<td>
<p-skeleton></p-skeleton>
</td>
<td>
<p-skeleton></p-skeleton>
</td>
....
</tr>
</ng-template>
</p-table>
Creating Angular Application and Installing the Modules:
Step 1: Create an Angular application using the following command.
ng new appname
Step 2: After creating your project folder i.e. appname, move to it using the following command.
cd appname
Step 3: Finally, Install PrimeNG in your given directory.
npm install primeng --save
npm install primeicons --save
Project Structure: The project Structure will look like this after following the above steps:
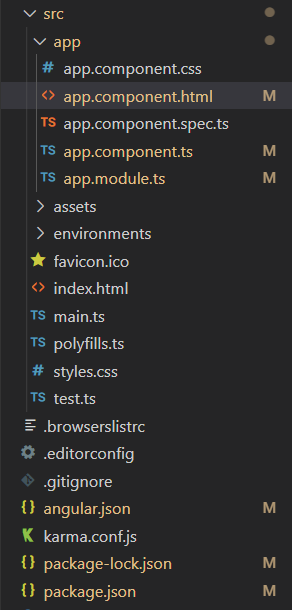
Project Structure
Run the below command to run the application:
ng serve --open
Example 1: This is a basic example illustrating how to show a skeleton data table with 2 columns and 3 rows.
app.component.html
< div
class = "page-content"
style = "height: calc(100vh - 149px)" >
< h2 style = "color: green" >GeeksforGeeks</ h2 >
< h4 >Angular PrimeNG Skeleton DataTable</ h4 >
< p-table [value]="tableData" responsiveLayout = "scroll" >
< ng-template pTemplate = "header" >
< tr >
< th >First Name</ th >
< th >Last Name</ th >
</ tr >
</ ng-template >
< ng-template pTemplate = "body" let-product>
< tr >
< td >
< p-skeleton ></ p-skeleton >
</ td >
< td >
< p-skeleton ></ p-skeleton >
</ td >
</ tr >
</ ng-template >
</ p-table >
</ div >
|
app.component.ts
import { Component } from '@angular/core' ;
interface People {
firstname?: string;
lastname?: string;
}
@Component({
selector: 'app-root' ,
templateUrl: './app.component.html' ,
})
export class AppComponent {
tableData: People[] = [];
constructor() { }
ngOnInit() {
this .tableData = [
{
firstname: 'Varun' ,
lastname: 'Pratap' ,
},
{
firstname: 'Badal' ,
lastname: 'Mishra' ,
},
{
firstname: 'Abhishek' ,
lastname: 'Thakur' ,
}
];
}
}
|
app.module.ts
import { NgModule } from '@angular/core' ;
import { BrowserModule } from '@angular/platform-browser' ;
import { FormsModule } from '@angular/forms' ;
import { BrowserAnimationsModule }
from '@angular/platform-browser/animations' ;
import { AppComponent } from './app.component' ;
import { TableModule } from 'primeng/table' ;
import { SkeletonModule } from 'primeng/skeleton' ;
@NgModule({
imports: [
BrowserModule,
BrowserAnimationsModule,
TableModule,
FormsModule,
SkeletonModule
],
declarations: [AppComponent],
bootstrap: [AppComponent],
})
export class AppModule { }
|
Output:
Example 2: This is another example showing how to use a skeleton data table in Angular PrimeNG. Here we have 4 Columns and 8 Rows.
app.component.html
< div
class = "page-content"
style = "height: calc(100vh - 149px)" >
< h2 style = "color: green" >GeeksforGeeks</ h2 >
< h4 >Angular PrimeNG Skeleton DataTable</ h4 >
< p-table [value]="tableData" responsiveLayout = "scroll" >
< ng-template pTemplate = "header" >
< tr >
< th >First Name</ th >
< th >Last Name</ th >
< th >Age</ th >
< th >Occupation</ th >
</ tr >
</ ng-template >
< ng-template pTemplate = "body" let-product>
< tr >
< td >
< p-skeleton ></ p-skeleton >
</ td >
< td >
< p-skeleton ></ p-skeleton >
</ td >
< td >
< p-skeleton ></ p-skeleton >
</ td >
< td >
< p-skeleton ></ p-skeleton >
</ td >
</ tr >
</ ng-template >
</ p-table >
</ div >
|
app.component.ts
import { Component } from '@angular/core' ;
interface People {
firstname?: string;
lastname?: string;
age?: number;
occupation?: string;
}
@Component({
selector: 'app-root' ,
templateUrl: './app.component.html' ,
})
export class AppComponent {
tableData: People[] = [];
constructor() { }
ngOnInit() {
this .tableData = [
{
firstname: 'Varun' ,
lastname: 'Pratap' ,
age: 20,
occupation: "Student"
},
{
firstname: 'Badal' ,
lastname: 'Mishra' ,
age: 21,
occupation: "Engineer"
},
{
firstname: 'Abhishek' ,
lastname: 'Thakur' ,
age: 23,
occupation: "Businessman"
},
{
firstname: 'Karan' ,
lastname: 'Patel' ,
age: 19,
occupation: "Sportsman"
},
{
firstname: 'Pallavi' ,
lastname: 'Yadav' ,
age: 20,
occupation: "Student"
},
{
firstname: 'Abhinav' ,
lastname: 'Yadav' ,
age: 21,
occupation: "Software Developer"
},
{
firstname: 'Abhay' ,
lastname: 'Rathore' ,
age: 23,
occupation: "Intern"
},
{
firstname: 'Abhishek' ,
lastname: 'Kumar' ,
age: 34,
occupation: "Doctor"
},
];
}
}
|
app.module.ts
import { NgModule } from '@angular/core' ;
import { BrowserModule } from '@angular/platform-browser' ;
import { FormsModule } from '@angular/forms' ;
import { BrowserAnimationsModule }
from '@angular/platform-browser/animations' ;
import { AppComponent } from './app.component' ;
import { TableModule } from 'primeng/table' ;
import { SkeletonModule } from 'primeng/skeleton' ;
@NgModule({
imports: [
BrowserModule,
BrowserAnimationsModule,
TableModule,
FormsModule,
SkeletonModule
],
declarations: [AppComponent],
bootstrap: [AppComponent],
})
export class AppModule { }
|
Output:
Reference: http://primefaces.org/primeng/skeleton
Share your thoughts in the comments
Please Login to comment...