Angular Components Overview
Last Updated :
16 Apr, 2024
Angular Components are the building blocks of Angular applications, containing the template, styles, and behavior of a part of the user interface. This article provides an overview of Angular components, including their structure, features, and how to create and use them effectively.
What are Angular Components ?
Angular components are the building blocks of Angular applications. They encapsulate a part of the user interface, including its template, styles, and behavior. Each component represents a reusable piece of UI functionality and can be composed together to create complex applications. Components in Angular follow the principles of encapsulation, reusability, and maintainability, making them essential in Angular development.
Component Structure
The structure of an Angular component consists of three main parts:
- Template: The template defines the HTML markup of the component’s view. It contains placeholders and Angular directives that are replaced with dynamic data and logic during runtime.
- Styles: The styles define the component’s visual appearance, including CSS rules and stylesheets. Styles can be defined using inline styles, external CSS files, or CSS preprocessors like Sass or Less.
- TypeScript Code: The TypeScript code defines the component’s behavior and logic. It includes properties, methods, and lifecycle hooks that control how the component interacts with the DOM, handles user input, and responds to changes in its state or props.
Component Lifecycle
Angular components have a lifecycle consisting of various lifecycle hooks that are executed at different stages of the component’s lifecycle. These lifecycle hooks allow to hook into specific moments in the component’s lifecycle and perform actions such as initialization, cleanup, or handling changes.
Some of the most commonly used lifecycle hooks include:
- ngOnInit: Called after the component’s inputs are initialized and the component’s view has been initialized.
- ngOnChanges: Called whenever the component’s inputs change.
- ngOnDestroy: Called when the component is being destroyed and cleaned up.
Data Binding
Data binding in Angular allows for communication between the component’s TypeScript code and its template. There are three types of data binding in Angular:
- One-Way Binding: Data flows from the component’s TypeScript code to its template. Changes in the component’s properties are reflected in the template, but changes in the template do not affect the component’s properties.
- Two-Way Binding: Data flows both ways between the component’s TypeScript code and its template. Changes in the component’s properties are reflected in the template, and changes in the template are propagated back to the component’s properties.
- Event Binding: Allows the template to listen for events triggered by the user or the browser and execute corresponding methods in the component’s TypeScript code.
Input properties allow data to be passed into a component from its parent component, while output properties allow a component to emit events to its parent component. Input properties are defined using the @Input decorator, while output properties are defined using the @Output decorator along with EventEmitter.
Component communication
Component communication in Angular involves passing data between components and coordinating their behavior. There are several methods for component communication:
- Parent-Child Communication: Data can be passed from a parent component to a child component using input properties, and events can be emitted from the child component to the parent component using output properties.
- Sibling Communication: Sibling components can communicate indirectly through their common parent component by passing data through input and output properties or using a shared service to store and exchange data.
- Communication Between Unrelated Components: Components that are not directly related can communicate through a shared service. The shared service acts as a mediator, allowing components to exchange data and coordinate their behavior without having a direct relationship.
Features
- Reusability: Components promote code reusability by encapsulating functionality and UI elements.
- Encapsulation: Components have their own scope and encapsulate their template, styles, and behavior.
- Maintainability: Components make it easier to maintain and organize code by breaking it into smaller, manageable pieces.
Steps to Create App, Example with Output:
Step 1: Create a new Angular app:
ng new my-angular-app
Step 2: Move to the Project Directory:
cd my-angular-app
Step 3: Generate a new component:
ng generate component my-component
Project Structure:
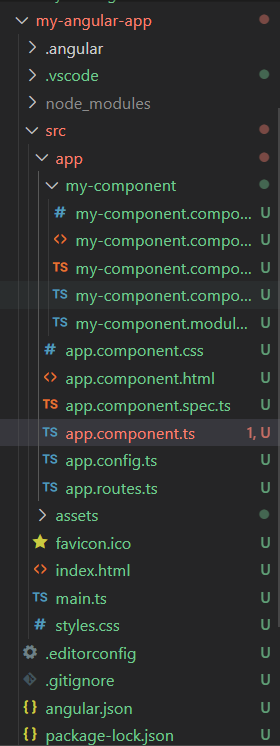
Step 4: Edit the component files:
Edit the generated component files (`my-component.component.html`, `my-component.component.css`, `my-component.component.ts`) to define the template, styles, and behavior of the component.
HTML
<!-- app.component.html -->
<app-my-component></app-my-component>
HTML
< !-- my - component.component.html-- >
<div>
<h1>Welcome to My Component!</h1>
<p>{{ message }}</p>
<button (click)="changeMessage()">Change Message</button>
</div >
JavaScript
//component.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-my-component',
standalone: true,
imports: [],
templateUrl: './my-component.component.html',
styleUrl: './my-component.component.css'
})
export class MyComponentComponent {
message: string = 'Initial message';
changeMessage() {
this.message = 'New message';
}
}
Step 5: Run your Application:
ng serve
Output:
When you run your Angular app (`ng serve`), you’ll see the message “Welcome to My Comonent!” displayed on the screen. Clicking the “Change Message” button will update the message to “New message” dynamically.
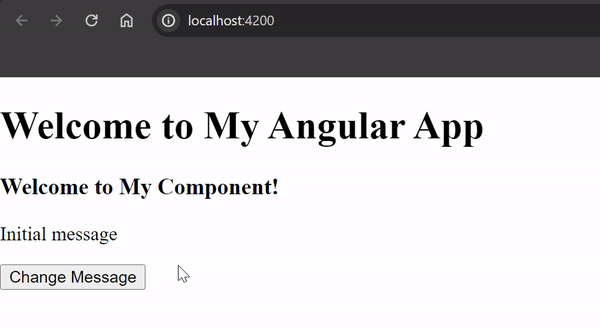
Conclusion
Angular components are powerful building blocks for creating dynamic and interactive web applications. Understanding their structure, features, and how to create and use them effectively is essential for Angular development. This article provides a comprehensive overview of Angular components, helping developers get started with building Angular applications.
FAQs
1. What is the difference between a component and a directive in Angular?
In Angular, a component is a type of directive with a template. Components are typically used to create reusable UI elements with associated behavior and styling. Directives, on the other hand, are used to add behavior to elements in the DOM or to modify the structure of the DOM itself.
2. Can a component have multiple templates?
No, a component in Angular can only have one template associated with it. However, you can use structural directives like ngIf, ngFor, and ngSwitch within the template to conditionally render different parts of the UI based on certain conditions.
3. What is the purpose of the @ViewChild decorator in Angular components?
The @ViewChild decorator in Angular is used to access child components, directives, or DOM elements from the parent component. It allows the parent component to query and interact with its child components programmatically.
4. How can I optimize the performance of Angular components?
To optimize the performance of Angular components, you can use techniques like lazy loading, code splitting, and preloading modules to minimize initial load times. Additionally, you can implement change detection strategies like OnPush and memoization to reduce unnecessary re-renders and improve rendering performance.
Share your thoughts in the comments
Please Login to comment...