Angular is a client-side TypeScript-based, front-end web framework developed by the Angular Team at Google, that is mainly used to develop scalable single-page web applications(SPAs) for mobile & desktop.
Angular is a great, reusable UI (User Interface) library for developers that helps in building attractive, steady, and utilitarian web pages and web applications. It is a continuously growing and expanding framework that provides better ways for developing web applications. It changes the static HTML to dynamic HTML. Its features like dynamic binding and dependency injection eliminate the need for code that we have to write otherwise.
TypeScript is a Strict Super Set of JavaScript, which means anything that is implemented in JavaScript can be implemented using TypeScript.
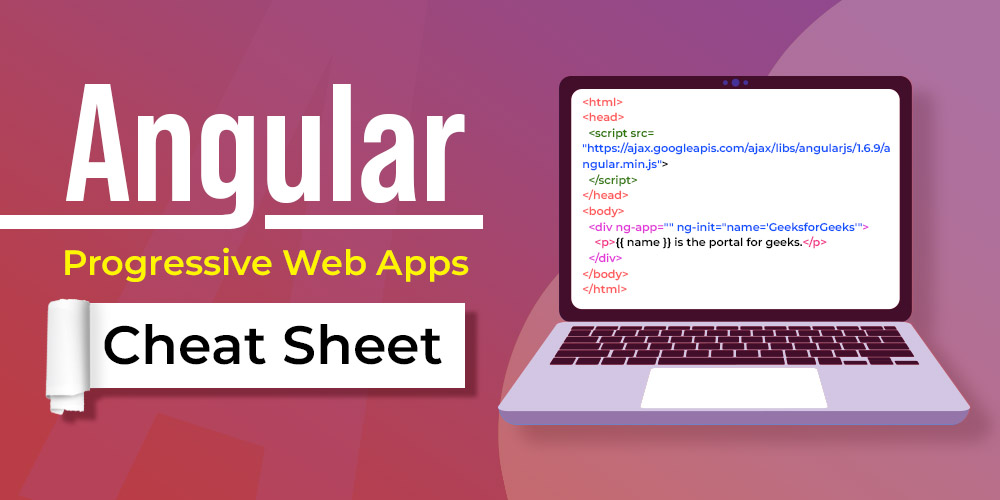
Angular Cheat Sheet
What is Angular Cheat Sheet?
The Angular Cheat Sheet will give quick ideas related to the topics like Basics, Lifecycle Hooks, Components & Modules, Directives, Decorators, Angular Forms, Pipes, Services, Routing & many more, which will provide you a gist of Angular with their basic implementation.
The purpose of the Cheat Sheet is to provide you with the content at a glance with some quick accurate ready-to-use code snippets that will help you to build a fully-functional web application.
Angular Introduction
Angular is a component-based application design framework, build on Typescript, for creating a sophisticated & a scalable single-page web application that has a well-integrated collection of libraries that helps to develop the code in module-wise with an easy-to-update, debug module. In order to work with Angular, we must have node.js, Node Package Manager(NPM) & Angular CLI installed in the system.
Please refer to the Installation of Node.js on Windows/Linux/Mac article for the detailed installation procedure.
Creating a New Angular Project
After successful installation of the node.js & npm, we need to install the Angular CLI, which is described below:
Open the terminal/Command prompt & type the below command:
npm install -g @angular/cli
For checking the version of the node.js & angular CLI installed, type the below command in the cmd:
node -v
ng -v or ng --version
Now, we will create the Angular application using the Angular CLI, as given below:
ng new Project-Name
You may navigate to any of the directories where you want to create the project.
Run the application: Navigate the folder where the project has been created & type the below command to run the app:
cd Project-Name
ng serve --open
The ng serve command will launch the server & will open your browser to http://localhost:4200/.
Boilerplate
Example 1: This example illustrates the basic Angular web app.
HTML
<!-- app.component.html -->
<div>
<h1>{{name}}</h1>
<h3>{{details}} </h3>
</div>
CSS
/* app.component.css */
div {
text-align: center;
font-family: Arial, Helvetica, sans-serif;
}
h1 {
color: green;
}
JavaScript
// app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
name = 'GeeksforGeeks';
details = 'A Computer Science portal for geeks';
}
JavaScript
//app.module.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Angular Fundamental
This section will cover the Components, Creation of Components, Lifecycle of the Component, and various directives provided by Angular & lastly, we will discuss the Decorator.
The Component is the basic building block for developing UI for any Angular app, which contains the tree of Angular Component. The Directives are the subset for the components, where the Components are always associated with the template. Only a single Component would be instantiated for a given element in the template.
The Components should belong to the NgModule, which helps to avail the component to another component or application.
In order to do this, the component should be listed in the declarations field of the NgModule metadata.Â
Creating Component:
The Angular application is composed of a different set of components, where every component has different roles & functionality that is designed to perform the specific task. The Components can be created with the following command:
ng generate component <component-name>
Please refer to the Components in Angular 8 article for further detailed descriptions.
Lifecycle Hooks of Component:
Every Component has its own lifecycle, which will be started when Angular instantiates the particular Component class that renders the Component view along with the child view. It is basically timed methods that differ when and why they execute. These methods will be triggered with the change detection, i.e., depending upon the conditions, the corresponding cycle will be executed.
Angular will constantly check when the data-bound properties changes & accordingly update both the view & the Component instances, as required. The order of the execution for these lifecycle hooks is important.
The Component instance will be destroyed & will be removed its rendered templates from the DOM by Angular when the lifecycle ends.
The different lifecycle hook methods for the Component are described below:
Methods | Descriptions | Syntax |
---|
ngOnChanges()
| Triggered when the Angular set or reset the data-bound input properties
| export class AppComponent implements OnChanges { @Input() geeks: string; lifecycleCount: number = 20; ngOnChanges() { Â this.lifecycleCount–; } }
|
ngOnInit()
| Used to initialize the Component or Directive after Angular sets the initial display of data-bound properties and sets input properties.
| export class AppComponent implements OnInit { @Input() geeks: string; lifecycleCount: number = 20; ngOnInit() { Â Â this.lifecycleCount–; } }
|
ngDoCheck()
| Called immediately after changes detected by ngOnChanges() and after the initial execution of ngOnInit().
| ngDoCheck() { Â console.log(++this.lifecycleCount); Â … Â } }
|
ngAfterContentInit()
| Used to perform initialization after Angular projects external content into the component’s view.
| ngAfterContentInit() { … }
|
ngAfterContentChecked()
| ngAfterContentChecked() is utilized to perform checks after Angular checks the content projected into the component’s view.
| ngAfterContentChecked() { … }
|
ngAfterViewInit()
| ngAfterViewInit() is employed to execute logic after Angular initializes the component’s views and child views.
| ngAfterViewInit() { … }
|
ngAfterViewChecked()
| ngAfterViewChecked() is utilized to execute logic after Angular checks the component’s views and child views for changes.
| ngAfterViewChecked() { … }
|
ngOnDestroy()
| ngOnDestroy() is used to perform cleanup logic when the component is destroyed or removed from the DOM.
| export class AppComponent { destroy: boolean = true; DataDestroy() { Â this.destroy = !this.destroy; }
|
Modules:
Every Angular application contains the root module, called NgModules, which acts as the container for the cohesive block of code, that is specifically designed to that application domain, a workflow, or a closely related set of capabilities. It consists of the components, services, & other code files, whose scope is decided by the NgModules.
The NgModules in the AppModule, reside in the app.module.ts file. The  @NgModule() class decorator is used to define the NgModule. It is a function that accepts one metadata object, whose properties describe the module.
Some of the important properties are described below:
Properties | Descriptions |
---|
declarations
| The components, directives, and pipes will belong to this NgModule.
|
exports
| It is a subset of declarations, which will be visible & usable should in the component templates of other NgModules.
|
imports
| It depicts the other modules whose exported classes are required by the component templates declared in this NgModule
|
providers
| It is the Creator of services, that is contributed to the global collection of services & becomes accessible in all parts of the application.
|
bootstrap
| The root component hosts all other application views. The bootstrap property should be set by the root NgModule only.
|
The data can be shared between the parent component and one or more child components by implementing the @Input() and @Output() decorators, which provide the child component a way to communicate with its parent component.
The @Input() decorator helps to update the data by the parent component in the child component, while the @Output() decorator helps to send the data by the child component to the parent component.
Angular Template:
The Template is a blueprint of the Angular application for developing the enhanced user interface, written in HTML & special syntax can be used within a template. Basically, each Template represents the section of the code on an HTML page that will be rendered in the browser with a lot more functionality.
While generating the application through the Angular CLI, the app.component.html will be the default template that will contain the HTML code. The Template Syntax helps to control the UX/UI by coordinating the data between the class and the template.
Angular provides several template syntax, which is described below:
Templates | Descriptions | Syntax |
---|
Text Interpolation
| It displays component properties in the view template, allowing properties from the component class to be reflected in the template.
| class=”{{variable_name}}” |
Template Statement
| Refers to event bindings or event handling mechanisms within the template syntax, allowing you to respond to user actions like clicks, input changes, etc.
| <element type=”button” (click)=”ChangeData()”>Delete</element> |
Binding
| Binding in Angular connects the HTML template with the model, syncing the view and model. It facilitates communication between them, focusing on the target receiving the value and the template expression producing it.
| <element [(expression)]=”ClassName”></element> |
Pipes
| It is used to transform the strings, currency amounts, dates, and other data, without affecting the actual content.Â
| {{ Expression | data }} |
We will learn about the various concepts of Binding & Pipes in more detail, in the underneath section.
Directives:
The Directive is generally a built-in class that includes the additional behavior to elements in the Angular Applications.
There are 2 types of directives:
- Attribute Directive: The Attribute Directive is used to modify the appearance or change the behavior of DOM elements & the components in Angular. In this case, the element, component, or another directive can be styled. The list of most commonly used attribute directives is:
Directives | Descriptions | Syntax |
---|
ngClass
| Built-in Angular directive used for dynamically adding or removing CSS classes from an HTML element based on certain conditions.
| <element [ng-class]=”expression”>Â Â Â Contents…Â </element>
|
ngStyle
| Â This directive is used to manipulate the styles for the HTML elements. It is a set of more than one style property, that is specified with colon-separated key-value pairs.
| <element [ngStyle] = “typescript_property”>
|
ngModel
| This directive is used to create a top-level form group Instance, and it binds the form to the given form value.
| <Input [(NgModel)= ‘name’]>
|
- Structural Directive: The Structural Directive is used to change the structure of the element or the component, i.e. it is used for modifying the structure of the DOM by applying or removing the elements from the DOM. The set of most commonly used built-in structural directives is:
Directives | Descriptions | Syntax |
---|
NgIf
| This directive is used to remove or recreate a portion of an HTML element based on an expression. If the expression inside it is false then the element is removed and if it is true then the element is added to the DOM.
| <element *ngIf=’condition’></element>
|
NgFor
| This directive is used to render each element for the given collection each element can be displayed on the page.
| <element *ngFor=’condition’></element>
|
NgSwitch
| This directive is used to specify the condition to show or hide the child elements.
| <element *NgSwitch=’condition’></element>
|
Decorators:
The Decorators are the function that is called with the prefix @ symbol, immediately followed by the class, methods, or property. The Service, filter, or directive are allowed to be modified by the Decorators before they are utilized.
It facilitates the metadata for configuration that decides how the components, function or class are to be processed, instantiated & utilized during the runtime.
All the Decorators can be categorized in 2 ways & each type of Decorator contains its own subset of the decorators, which are described below:
- Class Decorators: This decorator is facilitated by Angular having some class, which permits us to specify whether the particular class is a component or a module, along with permitting us to define this effect without having any code inside of it. For eg., the @Component that provides the metadata as part of the class & @NgModule clicks the widely used classes.
Some other Class decorators are:
Class Decorator | Descriptions | Syntax |
---|
import
| This is usually used to import the Directives from the @angular/core.
| import { NgModule } from ‘@angular/core’;
|
@Directive
| It is used to define the class as the Directive & provides its metadata of it.
| @Directive({ … })
|
@Pipe
| This is used to define the class as the Pipe, along with providing its metadata of it.
| @Pipe({ Â … })
|
@Injectable
| This is used to declare the class to be injected. When this is injected somewhere, the compiler can’t generate sufficient metadata that allows for the creation of the class appropriately, without having this decorator.
| @Injectable({ Â … })
|
- Class Field Decorator: This is another category of Decorator, which can contain the following types:
Class Field Decorators | Descriptions | Syntax |
---|
Property decorator
| This decorator can be utilized to decorate the particular properties declared inside the class. With this decorator, we can easily detect the purpose of using any particular property of the class. For eg., @Input(), @Output(), ReadOnly(), @Override() are most used property decorators.
| class GFG { Â @ReadOnly(‘check’)Â Â name: string = ‘DSA’; }
|
Method Decorators
| Method decorators like @HostListener and @HostBinding are used to decorate methods inside classes to add functionality, commonly linking host element properties with directive methods or binding host element properties with component properties.
| export class AppComponent { Â @HostListener(‘click’, [‘$event’]) Â onHostClick(event: Event) { code… Â } }
|
Parameter Decorator
| This decorator is permitted to decorate the parameter in the class constructors. The commonly used decorator of this type is @Inject, which is used for injecting the services in the Angular Class.Â
| export class GFGExample{ Â Â constructor(@Inject(gfgService) gfgServ) Â Â console.log(gfgServ); Â }
|
Example 2: This example describes the basic implementation of the Directives & the Decorators in Angular.
HTML
<!-- app.component.html File-->
<!-- ngClass & ngIf -->
<div *ngIf="true" [ngClass]="'gfgclass'">
This text will be displayed
<!-- ngStyle -->
<h1 [ngStyle]="{'color':'#00FF00'}">
GFG Structural Directive Example
</h1>
</div>
<hr>
<!-- ngFor -->
<div *ngFor="let item of items">
<p> {{item}} </p>
</div>
<hr>
<!-- ngSwitch -->
<div [ngSwitch]="'one'">
<div *ngSwitchCase="'one'">One is Displayed</div>
<div *ngSwitchCase="'two'">Two is Displayed</div>
<div *ngSwitchDefault>Default Option is Displayed</div>
</div>
<hr>
<h1>
GeeksforGeeks
</h1>
<hr>
<h2>GeeksforGeeks</h2>
<!-- ngModel -->
<input [(ngModel)]="gfg" #ctrl="ngModel" required>
<p>Value: {{ gfg }}</p>
<button (click)="setValue()">Set value</button>
JavaScript
// app.component.ts File
import { Component, VERSION } from "@angular/core";
@Component({
selector: "my-app",
templateUrl: "./app.component.html",
styleUrls: ["./app.component.css"],
})
export class AppComponent {
items = ["GfG 1", "GfG 2", "GfG 3", "GfG 4"];
gfg: string = "";
setValue() {
this.gfg = "GeeksforGeeks";
}
}
JavaScript
// app.module.ts File
import { NgModule } from "@angular/core";
import { BrowserModule }
from "@angular/platform-browser";
import { FormsModule } from "@angular/forms";
import { AppComponent } from "./app.component";
@NgModule({
imports: [BrowserModule, FormsModule],
declarations: [AppComponent],
bootstrap: [AppComponent],
})
export class AppModule {}
Data Bindings
Data Binding is a technique to automatically synchronize the data between the model and view components. Angular implements data-binding that treats the model as the single source of truth in your application & for all the time, the view is a projection of the model.
Unlike React, Angular supports two-way binding. In this way, we can make the code more loosely coupled.
Angular broadly categorizes the data flow sequence in 2 ways:
1. One-way Binding:
This type of binding is unidirectional, i.e. this binds the data flow from the component class to the view of the same Component or vice-versa. There are various techniques through which the data flow can be bound from component to view or vice-versa. If the data flow from component to view, then this task can be accomplished with the help of String Interpolation & Property Binding.
Binding | Description | Syntax |
---|
Attribute Binding
| It is used to set the values for attributes directly, which helps to improve accessibility, styling it dynamically, along with the ability to manage multiple CSS classes for styling simultaneously.
| <element [attr.attribute-you-are-targeting]=”expression”></element> |
Class & Style BindingÂ
| This binding is used to add or remove the used class name and styles from an HTML element’s class attribute, in order to set the styles dynamically.
| [class]=”classExpression” |
Event Binding | Event Binding in Angular allows handling user-triggered events such as button clicks, mouse movements, and keystrokes by listening and responding to these actions. It involves calling specified methods in the component when DOM events occur, enabling data binding between the DOM and the component for further processing.
| <element (event) = function()> |
Property Binding
| Property Binding in Angular is a one-way data-binding technique where we can bind a property of a DOM element to a field which is a defined property in our component TypeScript code.
| [class]=”variable_name” |
2. Two-way Binding:
The flow of data is bidirectional i.e. when the data in the model changes, the changes are reflected in the view and when the data in the view changes it is reflected in the model. Two-way data binding is achieved by using the ng-model directive.
The ng-model directive transfers data from the view to the model and from the model to the view. Basically, it is shorthand for a combination of property binding and event binding.
According to the direction of data flow, Angular provides 3 categories to bind the data:
- From data source to view target (One-way Binding)
- From view target to data source (One-way Binding)
- In a two-way data flow sequence of view target to the data source to view (Two-way Binding)
Depending upon the direction of data flow, Angular provides the following concepts that help to bind the data flow either from source to view, view to source, or two-way sequence of view to the source to view, which are described below:
Binding | Description | Syntax |
---|
Two-way Binding
| This binding provides a way to share the data. It listens for events and updates values simultaneously between child & parent components.
| <app-component [(expression)]=”DataValue”></app-component> |
The different binding types with their category, are described below:
Binding Types | Category |
---|
Interpolation, Property, Attribute, Class, Style | One-way from the data source to view-target |
Event | One-way from view target to data source |
Two-way | Two-way |
Except for the Text interpolation, the Binding has the target name that is declared on the left-hand side of the equal sign, where the target can be property or the event, that is surrounded by a square bracket ([ ]) characters, parenthesis (( )) characters, or both ([( )]) characters.
The prefix of the binding punctuation of [], (), [()] specifies the direction of data flow, i.e.,
- The square bracket ([]) can be used to bind from source to view.
- The parenthesis (()) can be used to bind from view to a source.
- The [()] can be used to bind in a two-way sequence of view to the source to view.
The expression or statement declared on the right-hand side of the equal sign, is represented within double quote (“”) characters.
Please refer to the Difference between One-way Binding and Two-way Binding article for detailed differences between them.
Example 3: This example describes the basic implementation of the Data Binding In Angular.
HTML
<!--app.component.html File -->
<!--Interpolation -->
<h1>{{ title }}</h1>
<hr>
<!--Property Binding -->
<input style="color:green;
margin-top: 40px;
margin-left: 100px;"
[value]='title'>
<hr>
<!--Event Binding -->
<h1>GeeksforGeeks</h1>
<input (click)="gfg($event)"
value="Geeks">
<hr>
<!--Two-way Data Binding -->
<div style="text-align: center">
<h1 style="color: green">
GeeksforGeeks
</h1>
<h3>Two-way Data Binding</h3>
<input type="text"
placeholder="Enter text"
[(ngModel)]="val" />
<br />{{ val }}
</div>
JavaScript
//app.component.ts File
import { Component } from "@angular/core";
@Component({
selector: "my-app",
templateUrl: "./app.component.html",
styleUrls: ["./app.component.css"],
})
export class AppComponent {
title = "GeeksforGeeks";
gfg(event) {
console.log(event.toElement.value);
}
val: string;
}
JavaScript
//app.module.ts File
import { NgModule } from '@angular/core';
import { BrowserModule }
from '@angular/platform-browser';
import { FormsModule } from '@angular/forms';
import { AppComponent } from './app.component';
@NgModule({
imports: [ BrowserModule, FormsModule ],
declarations: [ AppComponent],
bootstrap: [ AppComponent ]
})
export class AppModule { }
The Angular Form is an integral part of any web application, which may contain the collection of controls for an input field, buttons, checkboxes, etc, along with having the validation check that enables to validate data entered by the user in an appropriate form.
There are 2 different approaches that are facilitated by Angular, to handle the user input through the forms, i.e., the reactive form & the template-driven form.
1. Template-Driven Approach:
The conventional form tag is used to make the form. The Form object representation for the tag will be interpreted & created by Angular. The various form controls can be included with the help of ngModel & the grouping for multiple control can be done by using the ngControlGroup module. For validating the form field, basic HTML validation can be used. In simple words, it completely depends on the directive, in order to create & manipulate the basic object model.
2. Reactive Forms: This form facilitates the model-driven approach, in order to handle the various form inputs whose values vary with time. This form is more robust, scalable, reusable & testable, as it provides direct & access explicitly to the basic form’s object model.
Example 4: This example describes the basic implementation of the Angular Forms.
HTML
<!-- app.component.html File-->
<h1>GeeksforGeeks</h1>
<input [(ngModel)]="gfg"
#ctrl="ngModel" required>
<p>Value: {{ gfg }}</p>
<button (click)="setValue()">
Set value
</button>
JavaScript
//app.component.ts File
import { Component, Inject } from "@angular/core";
import { PLATFORM_ID } from "@angular/core";
import { isPlatformWorkerApp } from "@angular/common";
@Component({
selector: "my-app",
templateUrl: "./app.component.html",
styleUrls: ["./app.component.css"],
})
export class AppComponent {
gfg: string = "";
setValue() {
this.gfg = "GeeksforGeeks";
}
}
JavaScript
//app.module.ts File
import { NgModule } from "@angular/core";
import { BrowserModule }
from "@angular/platform-browser";
import { FormsModule } from "@angular/forms";
import { AppComponent } from "./app.component";
@NgModule({
imports: [BrowserModule, FormsModule],
declarations: [AppComponent],
bootstrap: [AppComponent],
})
export class AppModule {}
Angular Pipes
Pipes in Angular can be used to transform the strings, currency amounts, dates, and other data, without affecting the actual content. This is a simple function that can be used with the interpolation(template expression), in order to accept the input value & return the transformed data.
In order to use the Pipe, add the pipe ( | ) symbol with the expression inside the template expression. Each Pipe can be declared only once & can be used throughout the application. Angular facilitates 2 kinds of Pipes, i.e., built-in pipes & custom pipes.
1. Built-in Pipes: These are the predefined pipes provided by Angular.
The list of built-in Pipes provided by Angular is described below:
Pipe | Descriptions | Syntax |
---|
DatePipe
| This pipe is used to format the date to the specified format.
| {{ value | date }}
|
DecimalPipeÂ
| This pipe is used to format a value according to digit options and locale rules.
| {{ value | number}}
|
PercentPipe
| This pipe is used to Transform a number into a percentage string.
| {{ value | percent [ : digitsInfo [ : locale ] ] }}
|
UpperCasePipe
| This pipe is used to Transform all the text to uppercase.
| {{ value | uppercase }}
|
LowerCasePipe
| This pipe is used to Transform all the text to lowercase.
| {{ value | lowercase }}
|
CurrencyPipe
| This pipe is used to transform a number to a currency string, formatted according to locale rules that determine group sizing and separator, decimal-point character.
| {{ value | currency }}
|
2. Custom Pipe:
The Custom pipes can be used for various use cases like formatting the phone number, highlighting the search result keyword, returning the square of a number, etc.
For generating the custom pipes, we can follow 2 ways:
By creating a separate file for the pipe, we have to manually set up & configure the pipe function with the component file & need to import it into the module file.
By using Angular CLI, it will set up all the necessary configurations in the component & module files automatically.
The Custom Pipes can further be defined by specifying as the pure & impure pipes, which are described below:
- Pure Pipes: It is the pipes that execute when it detects a pure change in the input value. A pure change is when the change detection cycle detects a change to either a primitive input value (such as String, Number, Boolean, or Symbol) or object reference (such as Date, Array, Function, or Object).
- Impure Pipes: It is the pipes that execute when it detects an impure change in the input value. An impure change is when the change detection cycle detects a change to composite objects, such as adding an element to the existing array.
Please refer to the Explain pure and impure pipe in Angular article for further details.
Example 5: This example describes the basic implementation of the Angular Pipes.
HTML
<!-- app.component.html File-->
<!-- DatePipe -->
<p>Date {{today | date}}</p>
<p>Time {{today | date:'h:mm a z'}}</p>
<!-- DemicalPipe -->
<p> Number: {{pi | number}} </p>
<p> Number with 4 digits: {{pi | number:'4.1-5'}} </p>
<!-- percentPipe -->
<div>
<p>1st: {{gfg | percent}}</p>
<p>2nd: {{geeks | percent:'4.3-5'}}</p>
</div>
<!-- Custom Pipe -->
<h2>
Product code without using
custom pipe is {{productCode}}
</h2>
<h2>
Product code using custom pipe
is {{productCode | arbitrary:'-'}}
</h2>
JavaScript
//app.component.ts File
import { Component } from "@angular/core";
@Component({
selector: "my-app",
templateUrl: "./app.component.html",
})
export class AppComponent {
today: number = Date.now();
pi: number = 3.14159;
geeks: number = 0.4945;
gfg: number = 2.343564;
productCode = "200-300";
}
JavaScript
//app.module.ts File
import { BrowserModule }
from "@angular/platform-browser";
import { NgModule } from "@angular/core";
import { AppComponent } from "./app.component";
import { ArbitraryPipe } from "./arbitrary.pipe";
@NgModule({
declarations: [AppComponent,
ArbitraryPipe],
imports: [BrowserModule],
providers: [],
bootstrap: [AppComponent],
})
export class AppModule {}
JavaScript
//arbitrary.pipe.ts File
import { Pipe, PipeTransform } from "@angular/core";
@Pipe({
name: "arbitrary",
})
export class ArbitraryPipe implements PipeTransform {
transform(value: string, character: string): string {
return value.replace(character, " ");
}
}
Services & Dependency Injection
The Service facilitates the services to various components, in order to complete a certain task or to perform the specific operation that needs to be accomplished by the application.
In simple words, Service is a class having a narrow, well-defined purpose. In order to increase the modularity and reusability, Angular help us to distinguish the Component from the Services.
The purpose of using the service is to organize & share the main application logic, data or functions, and models, in order to accomplish the requirement-specific task for the different components of Angular applications.
For this, we usually implement it through Dependency Injection.
Features of Service:
- The Service is a typescript class that has a @injectable decorator in it, which notifies that the class is a service that can be injected into the components which utilize that specific service. The other Services can also be injected as dependencies.
- The Services can be used to contain the business logic, as it can be shared with multiple components.
- The Components are singleton, i.e., the single instance of the Service will be created only, & the same instance will be utilized by every building block in the Angular application.
Dependency Injection:
Dependency Injection is part of Angular Framework, which is a coding pattern, where the dependencies are received by the class, from external sources, instead of creating them itself.
It allows the classes along with the Angular Decorators, like, Components, Directives, Pipes, and Injectables, that help to configure the dependencies, as required.
Angular facilitates the ability to inject the service into the Components, in order to provide access to that component to the service.
The Injectable() decorator describes the class as a service, which allows Angular to inject it into the Component as a dependency. The injector is the main technique, where it is used to create the dependencies & maintain the container of dependency instances, which will be reutilized when required.
A provider is an object which notifies the injectors about the process to create or obtain the dependency.
Creating a Service: The Service can be created by using the below syntax:
Syntax:
ng generate service <service-name>
Please refer to the Explain the steps for creating a services in Angular 2 article for the detailed description.
Example 6: This example describes the basic implementation of the Services & Dependency Injection in Angular.
HTML
<!-- app.component.html File-->
<h2>GeeksforGeeks Course Lists:</h2>
<p *ngFor="let c of courses">{{ c }}</p>
JavaScript
//app.component.ts File
import { Component } from "@angular/core";
import { CoursesService } from "./courses.service";
@Component({
selector: "my-app",
templateUrl: "./app.component.html",
styleUrls: ["./app.component.css"],
})
export class AppComponent {
courses: string[];
constructor(private _coursesService: CoursesService) {}
ngOnInit() {
this.courses = this._coursesService.getdata();
}
}
JavaScript
//app.module.ts File
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { AppComponent } from './app.component';
import { CoursesService } from './courses.service';
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule],
providers: [CoursesService], //FOR GLOBAL SERVICE
bootstrap: [AppComponent]
})
export class AppModule {}
JavaScript
//courses.service.ts File
import { Injectable } from '@angular/core';
@Injectable()
export class CoursesService {
constructor() {}
getdata(): string[] {
return [
'Data Structures,
'Algorithms',
'Programming Language',
'Aptitude',
];
}
}
Routing
The Router helps to navigate between pages that are being triggered by the user’s actions. The navigation happens when the user clicks on the link or enters the URL from the browser address bar.
The link can contain the reference to the router on which the user will be redirected. We can also pass other parameters with a link through angular routing.
For this, it helps to create a single-page application with multiple views and allows navigation between them. The routes are the JSON array where each object in the array defined the route. Angular allows for creating nested routing, which means sub-navigation or sub-page navigation between the available components.
Creation of Routing Modules: Routing can only be possible to create if we have at least 2 components. The following command can be used to create the routing:
ng generate module module_name --flat --module=a
Here,
- The flat flag depicts that this module file is required to be there inside the app folder & we don’t require to separate the folder for this module.
- The module flag denotes that this module is going to be part of the main app module.
Router-Outlet:
The Router-outlet component basically renders any component that the router module returns, i.e. it tells the router where to display the routed views. The below syntax is used to add the router component:
<router-outlet></router-outlet>
The routerLink directive takes a path & navigates the user to the corresponding component.
In other words, it is similar to href in HTML, which takes the path & compared it to all the paths defined in the routing module & the component that matches will be displayed in the router outlet.
The wildcard routes help to restrict the users to navigate to a part of the application that does not exist, i.e. when none of the path matches then the wildcard route will be used & it should always be the last route in the routes array.
The router helps to select the route any time the requested URL doesn’t match any router paths.
We can set up the wildcard route with the following command:
{ path: '**', component: <component-name> }
Here, the 2 asterisks (**) indicate this route’s definition is a wildcard route.
The Router CanActivate interface that a class can implement to be a guard deciding if a route can be activated. The navigation only continues, if all guards return true, otherwise the navigation will be canceled. If UrlTree is returned by any guard, then the current navigation will be canceled & new navigation will be initiated to the UrlTree returned from the guard.
The Router CanDeactivate interface that a class can implement to be a guard deciding if a route can be deactivated. The navigation only continues, if all guards return true, otherwise the navigation will be canceled.Â
Example 7: This example describes the basic implementation of Routing in Angular.
HTML
<!--app.component.html File-->
<!DOCTYPE html>
<html>
<head>
<style>
ul {
list-style-type: none;
margin: 0;
padding: 0;
overflow: hidden;
background-color: #333;
}
li {
float: left;
}
li a {
display: block;
color: white;
text-align: center;
padding: 14px 16px;
text-decoration: none;
}
li a:hover {
background-color: #04aa6d;
}
.active {
background-color: #333;
}
</style>
</head>
<body>
<ul>
<li>
<a class="active" href="#home"
routerLink="/">Home</a>
</li>
<li>
<a href="#contact"
routerLink="/contactus">Contact</a>
</li>
<li>
<a href="#about"
routerLink="/aboutus">About</a>
</li>
</ul>
<div style="text-align: center;
font-weight: bolder;
font-size: 50px">
<router-outlet></router-outlet>
</div>
</body>
</html>
HTML
<!--about-us.component.html File-->
<p>about-us works!</p>
<a class="btn btn-primary"
routerLink="/aboutus/our_employees">
Our Employees
</a>
<br>
<a class="btn btn-primary"
routerLink="/aboutus/our_company">
Our Company
</a>
HTML
<!--our-company.component.html File-->
<p>our-company works!</p>
<a class="btn btn-primary"
routerLink="/aboutus">
Back
</a>
HTML
<!--our-employees.component.html File-->
<p>our-employees works!</p>
<a class="btn btn-primary"
routerLink="/aboutus">
Back
</a>
JavaScript
//app-routing.module.ts File
import { NgModule } from "@angular/core";
import { RouterModule, Routes } from "@angular/router";
import { AboutUsComponent }
from "./about-us/about-us.component";
import { OurCompanyComponent } from
"./about-us/our-company/our-company.component";
import { OurEmployeesComponent } from
"./about-us/our-employees/our-employees.component";
import { ContactUsComponent }
from "./contact-us/contact-us.component";
import { HomeComponent } from "./home/home.component";
const routes: Routes = [
{
path: "",
component: HomeComponent,
},
{
path: "aboutus",
children: [
{
path: "",
component: AboutUsComponent,
},
{
path: "our_employees",
component: OurEmployeesComponent,
},
{
path: "our_company",
component: OurCompanyComponent,
},
],
},
{
path: "contactus",
component: ContactUsComponent,
},
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule],
})
export class AppRoutingModule {}
JavaScript
//app.module.ts File
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { FormsModule } from '@angular/forms';
import { RouterModule, Router } from '@angular/router';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { HomeComponent } from './home/home.component';
import { ContactUsComponent }
from './contact-us/contact-us.component';
import { AboutUsComponent }
from './about-us/about-us.component';
@NgModule({
declarations: [
AppComponent,
HomeComponent,
ContactUsComponent,
AboutUsComponent
],
imports: [BrowserModule, FormsModule],
bootstrap: [AppComponent]
})
export class AppModule {}
Share your thoughts in the comments
Please Login to comment...