Aitken’s Array or Bell Triangle
Last Updated :
13 Apr, 2023
The Bell triangle or Aitken’s array or the Pierce Triangle is the number triangle obtained by beginning the first row with the number one, then beginning subsequent rows with the last number of the previous row. The following spaces are filled out by adding the number in the preceding column to the number above it. Follow the image below to better understand it.
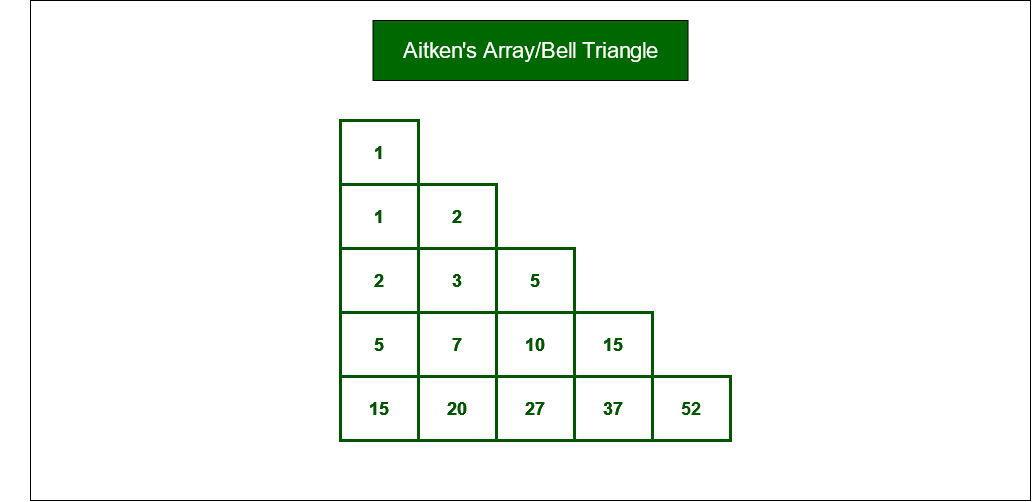
Aitken’s Array/Bell Triangle for N = 5
Problem:
Given an integer ‘n’, we need to print Aitken’s array upto ‘n’ rows.
Examples:
Input: 5
Output:
[1]
[1, 2]
[2, 3, 5]
[5, 7, 10, 15]
[15, 20, 27, 37, 52]
Input: 7
Output:
[1]
[1, 2]
[2, 3, 5]
[5, 7, 10, 15]
[15, 20, 27, 37, 52]
[52, 67, 87, 114, 151, 203]
[203, 255, 322, 409, 523, 674, 877]
Approach to create/print Aitken’s array:
The idea to create Aitken’s array is based on series/pattern printing.
If we look into the examples above, we can find a pattern between any two rows, i.e.
- The 1st element of the 1st row in the Aitken’s array is always equal to 1
- The 1st element of any row r in the Aitken’s array is equal to the last element of the previous row.
- The ith element of any row r in the Aitken’s array (aitkens[r][i]) = aitkens[r][i-1] + aitkens[r-1][i-1]
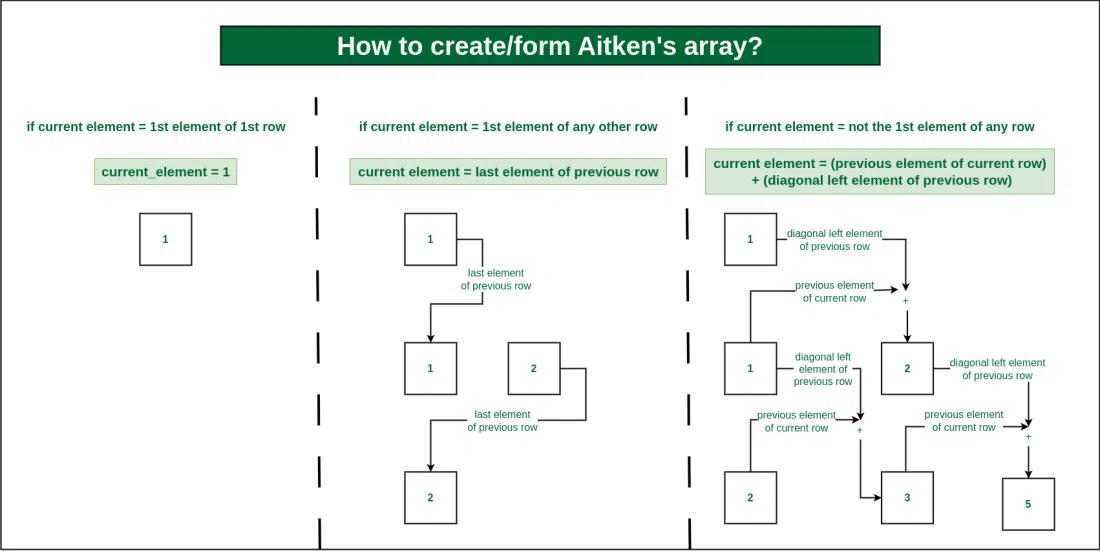
Approach to create/print Aitken’s array
Steps that were to follow the above approach:
- First, we have the base case, when n==1, we will return an array of only one element which is 1.
- Next, we have the recursive call which returns the answer for (n-1) rows, in the form of an array, which we store in arrayFromLastCall[].
- Next, we put in elements for the current row, arrayForCurrentCall[] in the following manner:
- The first element, we put into the current array is the last element of the previous array.
- For, the next elements, we form them by adding up the previous cell element from the current array and the element at the same position from the previous array.
- Finally, print the current array, and return this array.
Implementation of Aitken’s array
C++
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
vector< int > rec( int remainingNumberOfRows) {
if (remainingNumberOfRows == 1) {
vector< int > array {1};
cout << "[1]" << endl;
return array;
}
vector< int > arrayFromLastCall = rec(remainingNumberOfRows - 1);
vector< int > arrayForCurrentCall(remainingNumberOfRows);
arrayForCurrentCall[0] = arrayFromLastCall[arrayFromLastCall.size() - 1];
for ( int currentRow = 0; currentRow < remainingNumberOfRows - 1; currentRow++) {
arrayForCurrentCall[currentRow + 1] = arrayForCurrentCall[currentRow] + arrayFromLastCall[currentRow];
}
cout << "[" ;
for ( int i = 0; i < arrayForCurrentCall.size(); i++) {
cout << arrayForCurrentCall[i];
if (i != arrayForCurrentCall.size() - 1) {
cout << ", " ;
}
}
cout << "]" << endl;
return arrayForCurrentCall;
}
int main() {
int numberOfRows = 7;
cout << "Aitken's array of " << numberOfRows << ": " << endl << endl;
rec(numberOfRows);
return 0;
}
|
Java
import java.io.*;
import java.util.*;
public class AitkensArray {
static int [] rec( int remainingNumberOfRows)
{
if (remainingNumberOfRows == 1 ) {
int [] array = { 1 };
System.out.println(Arrays.toString(array));
return array;
}
int [] arrayFromLastCall
= rec(remainingNumberOfRows - 1 );
int [] arrayForCurrentCall
= new int [remainingNumberOfRows];
arrayForCurrentCall[ 0 ]
= arrayFromLastCall[arrayFromLastCall.length
- 1 ];
for ( int currentRow = 0 ;
currentRow < remainingNumberOfRows - 1 ;
currentRow++) {
arrayForCurrentCall[currentRow + 1 ]
= arrayForCurrentCall[currentRow]
+ arrayFromLastCall[currentRow];
}
System.out.println(
Arrays.toString(arrayForCurrentCall));
return arrayForCurrentCall;
}
public static void main(String[] args)
{
int numberOfRows = 7 ;
System.out.println( "Aitken's array of "
+ numberOfRows + ": \n" );
rec(numberOfRows);
}
}
|
Python3
def rec(remainingNumberOfRows):
if remainingNumberOfRows = = 1 :
array = [ 1 ]
print ( "[1]" )
return array
arrayFromLastCall = rec(remainingNumberOfRows - 1 )
arrayForCurrentCall = [ 0 ] * remainingNumberOfRows
arrayForCurrentCall[ 0 ] = arrayFromLastCall[ - 1 ]
for currentRow in range (remainingNumberOfRows - 1 ):
arrayForCurrentCall[currentRow + 1 ] = arrayForCurrentCall[currentRow] + arrayFromLastCall[currentRow]
print ( "[" , end = "")
for i in range ( len (arrayForCurrentCall)):
print (arrayForCurrentCall[i], end = "")
if i ! = len (arrayForCurrentCall) - 1 :
print ( ", " , end = "")
print ( "]" )
return arrayForCurrentCall
if __name__ = = "__main__" :
numberOfRows = 7
print (f "Aitken's array of {numberOfRows}: \n" )
rec(numberOfRows)
|
C#
using System;
public class AitkensArray
{
static int [] Rec( int remainingNumberOfRows)
{
if (remainingNumberOfRows == 1)
{
int [] array = { 1 };
Console.WriteLine( string .Join( ", " , array));
return array;
}
int [] arrayFromLastCall = Rec(remainingNumberOfRows - 1);
int [] arrayForCurrentCall = new int [remainingNumberOfRows];
arrayForCurrentCall[0] = arrayFromLastCall[arrayFromLastCall.Length - 1];
for ( int currentRow = 0; currentRow < remainingNumberOfRows - 1; currentRow++)
{
arrayForCurrentCall[currentRow + 1] = arrayForCurrentCall[currentRow] + arrayFromLastCall[currentRow];
}
Console.WriteLine( string .Join( ", " , arrayForCurrentCall));
return arrayForCurrentCall;
}
public static void Main()
{
int numberOfRows = 7;
Console.WriteLine( "Aitken's array of " + numberOfRows + ": \n" );
Rec(numberOfRows);
}
}
|
Javascript
function rec(remainingNumberOfRows) {
if (remainingNumberOfRows == 1) {
const array = [1];
console.log(array);
return array;
}
const arrayFromLastCall = rec(remainingNumberOfRows - 1);
const arrayForCurrentCall = new Array(remainingNumberOfRows);
arrayForCurrentCall[0] = arrayFromLastCall[arrayFromLastCall.length - 1];
for (let currentRow = 0; currentRow < remainingNumberOfRows - 1; currentRow++) {
arrayForCurrentCall[currentRow + 1] = arrayForCurrentCall[currentRow] + arrayFromLastCall[currentRow];
}
console.log(arrayForCurrentCall);
return arrayForCurrentCall;
}
const numberOfRows = 7;
console.log(`Aitken's array of ${numberOfRows}: \n`);
rec(numberOfRows);
|
Output
Aitken's array of 7:
[1]
[1, 2]
[2, 3, 5]
[5, 7, 10, 15]
[15, 20, 27, 37, 52]
[52, 67, 87, 114, 151, 203]
[203, 255, 322, 409, 523, 674, 877]
Time complexity : O(n^2)
Space complexity : O(n)
Share your thoughts in the comments
Please Login to comment...