Accessing State & Dispatch in Connected Components
Last Updated :
24 Apr, 2024
Accessing state and dispatch in connected components is a fundamental concept in React-Redux applications, where components are connected to the Redux store to access the application’s state and dispatch actions to update the state. In this article, a simple counter is displayed, allowing users to increase, decrease, or reset the count value by connecting React components to the Redux store.
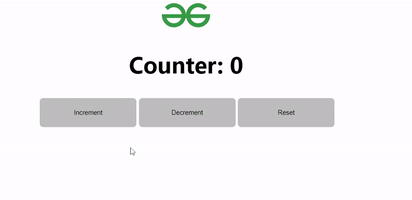
Redux Counter Project
Prerequisites:
Approach
- Project Setup – Creating a new React application using Create React App and install necessary dependencies like Redux and React-Redux.
- Set up Redux store: Use createStore() to create a Redux store and define initial state, reducers and actions (increment , decrement ).
- Creating Components – Designing the counter component for this application and utilizing React-Redux connect() function to connect the components to the Redux store.
- Accessing State – Using mapStateToProps to retrieve state properties from the Redux store.
- Dispatching – Using mapDispatchToProps function to dispatch actions to update the Redux store’s state.
Steps to Create Application
Step 1: Create React Application named redux-counter-app and navigate to it using this command.
npx create-react-app redux-counter-app
cd redux-counter-app
Step 2: Install required packages and dependencies.
npm install react-redux redux
Updated dependencies:
All required dependencies required for project will look like in package.json file.
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-redux": "^9.1.1",
"redux": "^5.0.1",
},
Project Structure:
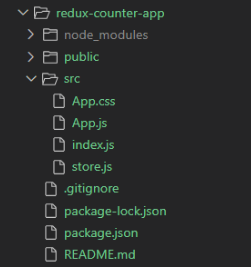
Project Structure
Example: To demonstrate accessing state and dispatch in connected components using counter app.
CSS
/* app.css */
#counter {
text-align: center;
}
button {
background-color: rgb(190, 190, 190);
padding: 10px 20px;
border-radius: 7px;
border: 1px solid transparent;
cursor: pointer;
margin-left: 5px;
}
button:hover {
background-color: rgba(0, 128, 0, 0.534);
}
JavaScript
// store.js
import { createStore } from 'redux'
// Initial state
const initialState = {
count: 0
};
// Reducer function
const counterReducer = (state = initialState, action) => {
switch (action.type) {
case 'INCREMENT':
return {
...state,
count: state.count + 1
};
case 'DECREMENT':
return {
...state,
count: state.count - 1
};
case 'RESET':
return {
...state,
count: 0
};
default:
return state;
}
};
export default createStore(counterReducer)
JavaScript
// App.js
import './App.css';
import React from 'react';
import { connect } from 'react-redux';
import { bindActionCreators } from 'redux';
const Counter = ({ count, increment, decrement, reset }) => {
return (
<div id="counter">
<img src="https://media.geeksforgeeks.org/gfg-gg-logo.svg" alt="gfg_logo" />
<h2>Counter: {count}</h2>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
<button onClick={reset}>Reset</button>
</div>
);
};
const mapStateToProps = (state) => {
return {
count: state.count
};
};
const mapDispatchToProps = (dispatch) => {
return bindActionCreators({
increment: () => dispatch({ type: 'INCREMENT' }),
decrement: () => dispatch({ type: 'DECREMENT' }),
reset: () => dispatch({ type: 'RESET' })
}, dispatch);
};
export default connect(mapStateToProps, mapDispatchToProps)(Counter);
JavaScript
// index.js
import React from 'react';
import ReactDOM from 'react-dom/client';
import App from './App';
import { Provider } from 'react-redux';
import store from './store'
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<Provider store={store}>
<App />
</Provider>
);
Output:
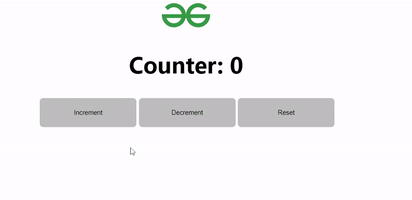
Redux Counter Project
Share your thoughts in the comments
Please Login to comment...