Working with Text Files in Julia
Last Updated :
25 Aug, 2020
Julia is Programming Language that is fast and dynamic in nature (most suitable for performing numerical and scientific applications) and it is optionally typed (the rich language of descriptive type and type declarations which is used to solidify our program) and general-purpose and open-sourced. Julia supports file handling like other programming languages with much higher efficiency and associated methods i.e reading, writing, and closing a file. It is a combination of C language ( powerful performance) and Python( simplicity).
Creation of a File
In order to work with a file in Julia, firstly we need to create a new file using “touch”(used to create a new empty file) method, “pwd”(used for checking the present working directory of the system) method and “cd”(used to change directory and create the file where we want) method.
touch("example.txt")
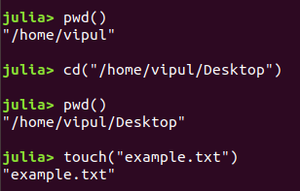
File Created
Opening an existing File
To open the file in Julia we have “open” method. The open method takes in two arguments i.e filename (file to open) and mode of operation (read, write or append).
For read mode we use (“r”), write mode(“w”), and append mode(“a”).
Julia
abc = open ( "example.txt" , "r" )
efg = open ( "example.txt" , "w" )
hij = open ( "example.txt" , "a" )
|
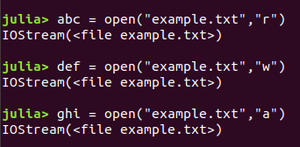
file open in different mode
Just like Python, Julia also has a “do“ method that helps us to avoid the mode of operation and prevent us from the issue of closing the file every time after modifying it.
Julia
open ( "example.txt" ) do file
end
|
Reading Contents of a File
Reading entire file
Here we will use “read” method to read the content of a file and it takes in two arguments i.e file to read and method to read the file as (string, integer, etc).
Julia
mydata = read(abc, String)
mydata = open ( "example.txt" ) do file
read( file , String)
end
|

File Read
Reading file line by line
Here we will use “readlines” method to ready the file as an array with each line of a file as an array element.
Julia
mni = open ( "example.txt" , "r" );
line_by_line = readlines(mni)
|

Writing to a File
Here we will change the mode of the file from read to write in order to write file inside “open” method.
Using write mode
Julia
abc = open ( "example.txt" , "w" )
|
Now , we will use write method in Julia to write content to the “example.txt ” file, and write method will delete the previous content from file or will create the file if it does not exist.
Julia
write(abc, " Hello World , Julia welcomes you" )
close(abc)
|
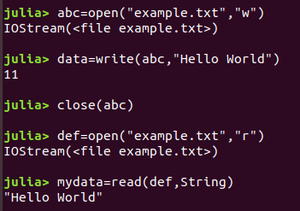
Using append mode
Julia
abc = open ( "example.txt" , "a" )
write(abc, " Hello World , Julia welcomes you" )
close(abc)
|
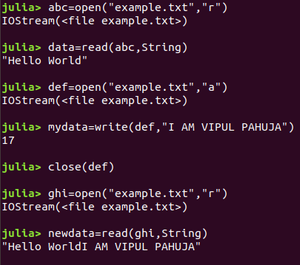
Modifying the contents of a file
Here we will learn to modify the content of a file i.e we will change the case of content in a file from uppercase to lowercase using the lowercase method.
Julia
open ( "example.txt" ) do abc
lowercase(read(abc, String))
end
|
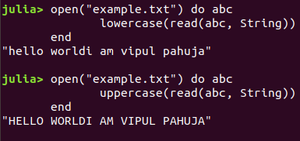
Closing the file
Here we will use the close method in Julia to close the file after modifying its content.
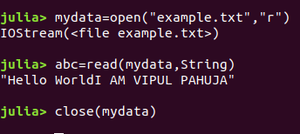
Share your thoughts in the comments
Please Login to comment...