Why is Unit Testing Harder in OOP?
Last Updated :
10 Mar, 2024
Unit testing is a crucial aspect of software development, serving as the first line of defense against bugs and ensuring the reliability and maintainability of code.
However, when it comes to object-oriented programming (OOP), unit testing often presents unique challenges that can make the process more complex and time-consuming. In this article, we’ll delve into the reasons why unit testing is harder in OOP and explore strategies to overcome these challenges.
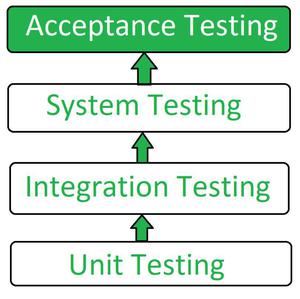
Types of Acceptance Testing
Understanding Object-Oriented Programming:
Object-oriented programming is a programming paradigm based on the concept of “objects,” which encapsulate data and behavior. OOP encourages the use of classes and objects to model real-world entities and relationships, leading to code that is modular, reusable, and easier to maintain.
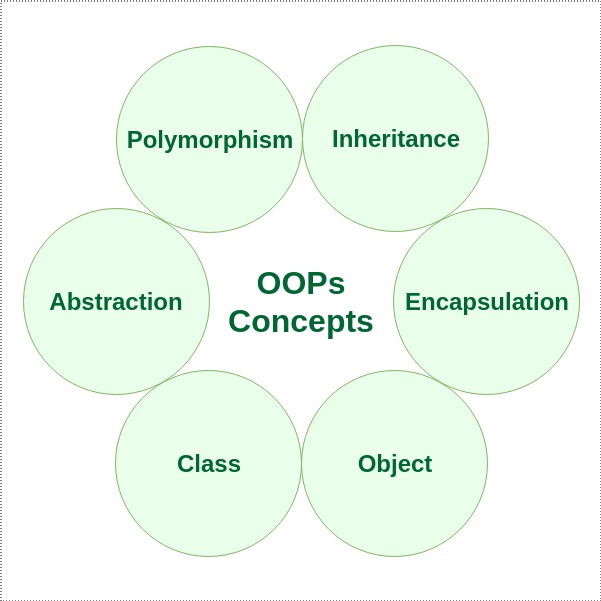
Pillars of OOPs
Steps of Unit Testing
- Identify Units to Test: Break down the code into small, testable units such as functions, methods, or classes. Units should represent logical units of functionality that can be tested independently.
- Write Test Cases: For each unit, write test cases that cover different scenarios and edge cases to verify its behavior. Test cases should include input values, expected outcomes, and assertions to validate the results.
- Set Up Testing Environment: Configure a testing environment that mimics the production environment but is isolated from external dependencies such as databases, APIs, or external services. Use tools like mocking frameworks or dependency injection to simulate external dependencies.
- Implement Tests: Write the test code using a testing framework such as JUnit (for Java), NUnit (for .NET), pytest (for Python), or Jasmine (for JavaScript). Organize test cases into test suites and ensure each is independent and self-contained.
- Run Tests: Execute the unit tests to verify the functionality of the code. Test runners provided by testing frameworks automate the process of running tests and reporting results. Analyze the test output to identify any failures or errors.
- Debug and Refactor: If any tests fail, debug the code to identify the root cause of the failure. Make necessary changes to the code to fix the issues and rerun the tests to ensure they pass. Refactor the code as needed to improve clarity, performance, or maintainability.
- Coverage Analysis: Measure code coverage to determine the percentage of code that is exercised by the unit tests. Aim for high code coverage to ensure thorough testing of the codebase. Tools like JaCoCo, Cobertura, or Istanbul can be used for code coverage analysis.
- Regression Testing: As the codebase evolves, rerun the unit tests regularly to catch regressions introduced by new changes. Continuous integration (CI) tools like Jenkins, Travis CI, or GitHub Actions can automate the process of running tests whenever changes are pushed to the code repository.
- Document and Maintain Tests: Document the purpose and expected behavior of each unit test to aid in understanding and maintaining the codebase. Update tests as needed to reflect changes in requirements or code implementations.
- Integrate with Build Process: Incorporate unit tests into the build process to ensure that all tests pass before deploying the application to production. This helps catch issues early and maintain the overall quality of the software.
By following these steps, developers can effectively test individual units of code to ensure they meet the specified requirements and maintain the overall reliability and robustness of the software.
Challenges of Unit Testing in OOP
Sure, let’s focus solely on detailing the problems associated with unit testing in OOP:
- Tight Coupling: Tight coupling between classes or components makes it challenging to isolate units for testing. When classes are tightly coupled, changes in one class often require changes in multiple other classes. This increases the effort required to set up tests and makes tests more brittle, as modifications in one part of the system can inadvertently affect the behavior of other parts.
- Mocks and Stubs: Managing mocks and stubs can be complex, especially with deeply nested dependencies or interfaces that frequently change. Creating and configuring mocks or stubs for every test case can lead to verbose and difficult-to-maintain test code. Moreover, if mocks or stubs are not set up correctly, they may not accurately simulate the behavior of the actual dependencies, leading to unreliable test results.
- State Management: OOP often relies on stateful objects, which introduces challenges in managing the state between tests. Ensuring that each test sets up its required state and cleans up afterward can be cumbersome, particularly when tests need to be run in a specific order to maintain correctness. Incorrect state management can lead to test pollution, where the outcome of one test affects the results of subsequent tests.
- Inheritance: Inheritance can complicate unit testing, especially when testing subclasses. Changes in parent classes can propagate to subclasses, potentially breaking existing unit tests. Furthermore, testing subclasses in isolation may be challenging, as they inherit behavior from their parent classes, making it difficult to isolate and test specific behaviors unique to the subclass.
- Visibility: OOP encourages encapsulation and information hiding, which can hinder access to private or protected methods and properties for testing purposes. Testing private or protected members often requires workarounds or compromises in encapsulation, which can lead to maintenance issues and potentially compromise the integrity of the tested code. Additionally, relying solely on testing public interfaces may not provide adequate coverage, as certain edge cases or error scenarios may only be accessible through non-public methods or properties.
Strategies for Overcoming Challenges
Dependency Injection:
- Tight Coupling: By employing dependency injection, classes accept dependencies as parameters rather than creating them internally. This promotes loose coupling by allowing dependencies to be easily substituted with mocks or stubs during testing.
- State Management: Dependency injection facilitates better control over the state of objects by providing the ability to inject different dependencies with varying states for each test case. This helps avoid a shared state between tests and ensures test independence.
- Visibility: Dependency injection encourages designing classes to rely on interfaces rather than concrete implementations, making it easier to access and test public interfaces while maintaining encapsulation.
Test-Driven Development (TDD):
- Tight Coupling: TDD encourages writing tests before writing production code. By defining the desired behavior of a unit first, developers are forced to consider the unit’s interface and dependencies, which often leads to more loosely coupled designs.
- State Management: TDD promotes writing small, isolated tests that focus on specific units of code. This approach inherently encourages better state management, as each test case sets up and tears down its required state independently.
- Inheritance: TDD drives the development of code in small, incremental steps. When using TDD, developers write tests for subclasses as they extend or modify the behavior of parent classes, ensuring that both parent and subclass behavior is thoroughly tested.
Mocking Frameworks:
- Mocks and Stubs: Mocking frameworks such as Mockito, Moq, or Jest provide utilities for creating and managing mocks or stubs. By using these frameworks, developers can easily replace dependencies with mocks or stubs, reducing the complexity of test setup and maintenance.
- Visibility: Mocking frameworks enable testing of private or protected methods and properties by providing ways to mock internal behavior indirectly. While it’s generally recommended to test public interfaces, mocking frameworks offer flexibility in accessing non-public members when necessary.
- By incorporating these strategies into your unit testing practices in OOP, you can effectively address the challenges posed by tight coupling, state management, inheritance, and visibility, leading to more maintainable and reliable tests.
Example of unit testing
C++
#include <iostream>
class Calculator {
public :
int add( int x, int y) { return x + y; }
};
int main()
{
Calculator calc;
int result = calc.add(5, 3);
if (result == 8) {
std::cout << "Addition test passed." << std::endl;
}
else {
std::cout << "Addition test failed." << std::endl;
}
return 0;
}
|
C
#include <stdio.h>
typedef struct {
int (*add)( int x, int y);
} Calculator;
int add( int x, int y) {
return x + y;
}
int main() {
Calculator calc = { add };
int result = calc.add(5, 3);
if (result == 8) {
printf ( "Addition test passed.\n" );
} else {
printf ( "Addition test failed.\n" );
}
return 0;
}
|
Java
public class Calculator {
public int add( int x, int y) { return x + y; }
public static void main(String[] args)
{
Calculator calc = new Calculator();
int result = calc.add( 5 , 3 );
if (result == 8 ) {
System.out.println( "Addition test passed." );
}
else {
System.out.println( "Addition test failed." );
}
}
}
|
Python
class Calculator:
def add( self , x, y):
return x + y
if __name__ = = "__main__" :
calc = Calculator()
result = calc.add( 5 , 3 )
if result = = 8 :
print ( "Addition test passed." )
else :
print ( "Addition test failed." )
|
Output:
Addition test passed.
Conclusion
Unit testing is a vital aspect of software development, but it can be particularly challenging in object-oriented programming due to factors such as dependency management, inheritance, encapsulation, and stateful objects. By understanding these challenges and adopting strategies such as dependency injection, design for testability, mocking frameworks, and test-driven development, developers can overcome the complexities of unit testing in OOP and build robust, maintainable software systems.
FAQ’s
1. Why is unit testing harder in Object-Oriented Programming (OOP) compared to other programming paradigms?
Ans: Unit testing in OOP can be more challenging due to factors such as tight coupling between classes, reliance on stateful objects, and difficulties in accessing private or protected members for testing purposes.
2. What is tight coupling, and how does it impact unit testing in OOP?
Ans: Tight coupling refers to strong dependencies between classes or components, making it difficult to isolate units for testing. In OOP, tight coupling can lead to challenges in substituting dependencies with mocks or stubs during testing, as changes in one class may require changes in multiple other classes.
3. How does state management affect unit testing in OOP?
Ans: OOP often relies on stateful objects, which can complicate unit testing. Managing the state between tests is crucial to ensure test independence and correctness. Poor state management can lead to test pollution, where the outcome of one test affects the results of subsequent tests.
4. How can developers ensure effective unit testing in an OOP environment?
Ans: Developers can ensure effective unit testing in OOP by adopting best practices such as writing modular and testable code, designing classes with testability in mind, and maintaining a comprehensive suite of unit tests that cover various scenarios and edge cases. Regular refactoring and code reviews can also help improve the testability and maintainability of codebases.
Share your thoughts in the comments
Please Login to comment...