Why global array has a larger size than the local array?
Last Updated :
15 Dec, 2023
An array in any programming language is a collection of similar data items stored at contiguous memory locations and elements can be accessed randomly using array indices. It can be used to store the collection of primitive data types such as int, float, double, char, etc of any particular type. For example, an array in C/C++ can store derived data types such as structures, pointers, etc. Below is the representation of an array.
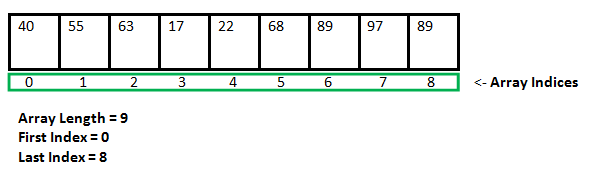
The arrays can be declared and initialized globally as well as locally(i.e., in the particular scope of the program) in the program. Below are examples to understand this concept better.
Program 1: Below is the C++ program where a 1D array of size 107 is declared locally.
C++
#include <bits/stdc++.h>
using namespace std;
int main()
{
const int N = 1e7;
int a[N];
a[0] = 1;
cout << a[0];
return 0;
}
|
Java
import java.io.*;
class GFG{
public static void main(String[] arg)
{
int N = ( int )1e7;
int a[] = {N};
a[ 0 ] = 1 ;
System.out.print(a[ 0 ]);
}
}
|
Python
if __name__ = = "__main__" :
N = 10 * * 5
a = [ 0 ] * N
a[ 0 ] = 1
print (a[ 0 ])
|
C#
using System;
class Program {
static void Main()
{
const int N = 10000000;
int [] a = new int [N];
a[0] = 1;
Console.WriteLine(a[0]);
}
}
|
Javascript
function main() {
const N = 1e7;
const a = new Array(N);
a[0] = 1;
console.log(a[0]);
}
main();
|
Output:
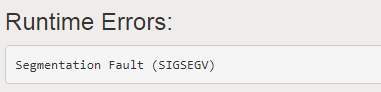
Explanation: In the above program, a segmentation fault error occurs when a 1-D array is declared locally, then the limit of that array size is the order of 105. It is not possible to declare the size of the array as more than 105. In this example, the array of size 107 is declared, hence an error has occurred.
Program 2: Below is the program where a 1-D array of size 105 is initialized:
C++
#include <bits/stdc++.h>
using namespace std;
int main()
{
const int N = 1e5;
int a[N];
a[0] = 1;
cout << a[0];
return 0;
}
|
Java
import java.io.*;
class GFG{
public static void main(String[] arg)
{
int N = 1 ;
int a[] = {N};
a[ 0 ] = 1 ;
System.out.print(a[ 0 ]);
}
}
|
Python3
N = 1e5
a = [N]
a[ 0 ] = 1
print (a[ 0 ])
|
C#
using System;
class GFG
{
public static void Main(String[] arg)
{
int N = 1;
int []a = {N};
a[0] = 1;
Console.Write(a[0]);
}
}
|
Javascript
<script>
var N = 1;
var a = [N];
a[0] = 1;
document.write(a[0]);
</script>
|
Explanation: In the above program, the code compilation is successful, and the output is 1. This is because the 1D array of size 105 is initialized locally and this is valid.
Program 3: Below is the program where a 1-D array of size 107 is declared globally.
C++
#include <bits/stdc++.h>
using namespace std;
const int N = 1e7;
int a[N];
int main()
{
a[0] = 1;
cout << a[0];
return 0;
}
|
Java
import java.io.*;
class GFG
{
public static void main(String[] arg)
{
int N = 1 ;
int a[] = {N};
a[ 0 ] = 1 ;
System.out.print(a[ 0 ]);
}
}
|
Python3
N = 1e7
a = [N]
a[ 0 ] = 1
print (a[ 0 ])
|
C#
using System;
class GFG
{
public static void Main(String[] arg)
{
int N = 1;
int []a = {N};
a[0] = 1;
Console.Write(a[0]);
}
}
|
Javascript
<script>
const N = 1e7;
let a = new Array(N);
a[0] = 1;
document.write(a[0]);
</script>
|
Explanation: In the above code compilation is successful, and the output is 1. This is because a 1-D array of size 107 is declared globally and this is valid.
Note: If a 1-D array of size 108 is declared globally, then again the segmentation fault error will be encountered because there is also a limit for global declaration of the 1-D array and that is, it is possible to only declare a 1-D array globally up to 107 sizes.
Why global array has a larger size than the local array?
- When an array is declared locally, then it always initializes in the stack memory, and generally, stack memory has a size limit of around 8 MB. This size can vary according to different computer architecture.
- When an array is declared globally then it stores in the data segment, and the data segment has no size limit. Hence, when the array is declared of big size (i.e., more than 107) then the stack memory gets full and leads into the stack overflow error, and therefore, a segmentation fault error is encountered. Therefore, for declaring the array of larger size, it is good practice to declare it globally.
Share your thoughts in the comments
Please Login to comment...