What is useDeferredValue hook and how to use it?
Last Updated :
12 Dec, 2023
In this article, we’ll be learning about useDeferredValue hook. The `useDeferredValue` hook in React allows you to postpone updating a component of the UI. This can be beneficial for boosting performance when rendering a specific section of the UI is expensive, or when you wish to prioritize rendering other parts of the UI.
The `useDeferredValue` hook is a useful addition to React 18, aimed to improve component rendering performance. It allows you to postpone the rendering of a component until a specific condition is met. This is especially useful when dealing with scenarios involving the display of large datasets or the execution of resource-intensive computations.
Syntax:
const deferredValue = useDeferredValue(value);
Project Structure :
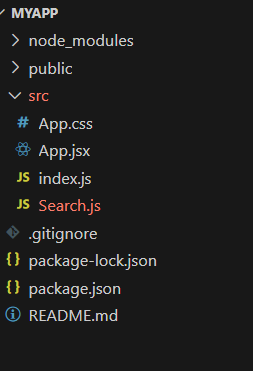
Project Structure
Steps to create react app
Step 1:Â Create a React application using the following command:
npx create-react-app foldername
Step 2: After creating your project folder i.e. foldername, move to it using the following command:
cd foldername
How to use the useDeferredValue hook?
To utilize the `useDeferredValue` hook, simply feed it the value to be deferred. The postponed version of that value will be returned by the hook. This means that when the value changes, the deferred value does not change instantly. It will instead be updated in the background. The rest of the UI will be rendered before the delayed value is changed.
Syntax
import { useState, useDeferredValue } from 'react';
function SearchPage() {
const [query, setQuery] = useState('');
const deferredQuery = useDeferredValue(query);
// ...
}
Note: The delayed value returned is the same as what you entered during the original rendering. During updates, React first tries to re-render with the old value (returning the old value) and then re-render the background with the new value (returning the updated value).
Example : In this example we will see the implementation of how to use deferredValue hook with an example.
Javascript
import {
useDeferredValue,
useEffect,
useState,
} from 'react' ;
import Search from './Search' ;
const ExampleFunction = () => {
const [query, setQuery] = useState( '' );
const deferredQuery = useDeferredValue(query);
return (
<div style={{ margin: "5rem" , }}>
<div className= 'tutorial' >
<input
type= 'text'
value={query}
onChange={(e) => setQuery(e.target.value)}
placeholder= 'Search...'
/>
<Search text={deferredQuery} />
</div>
</div>
);
};
export default ExampleFunction;
|
Javascript
import React from 'react' ;
const SlowList = React.memo(({ text }: { text: string }) => {
const items: React.ReactNode[] = [];
for (let i = 0; i < 250; i++) {
items.push(<SlowItem key={i} text={text} />);
}
return <ul className= 'items' >{items}</ul>;
});
const SlowItem = ({ text }: { text: string }) => {
let startTime = performance.now();
while (performance.now() - startTime < 1) {
}
return <li className= 'item' >Search Result: {text}</li>;
};
export default SlowList;
|
Output:
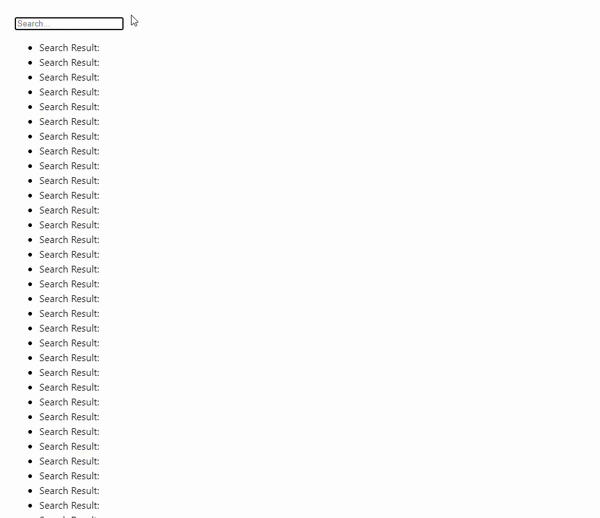
useDeferredValue
Each item in the Search component is purposefully slowed down in this example to demonstrate how `useDeferredValue` allows you to keep the input responsive. When you type into the input, you’ll notice that the list “lags behind” you.
So, in the component hierarchy:
- `ExampleFunction` component manages the state of the search (`query`).
- `Search component` receives the search query as a prop (`text`) and renders the search results.
Use cases of the useDeferredValue hook:
- Showing stale content while fresh content is loading: If you have a component that displays a list of things, you can use useDeferredValue to postpone the list’s presentation until the items have been loaded. This allows the user to see the stale material while the fresh content loads, which might enhance your application’s perceived performance.
- Deferring re-rendering for a part of the UI: If your component has a complicated chart, you may use useDeferredValue to delay rendering the chart until the rest of the component is rendered. This can increase your application’s perceived performance because the user will not have to wait for the chart to render before interacting with the rest of the application.
You can reduce the overall impact on your application and improve its responsiveness by postponing the rendering of certain items until they are actually required. This is especially useful when dealing with sluggish or unpredictable network connections, as it allows you to display stale content while waiting for new data to come.
Conclusion:
Overall, the `useDeferredValue` hook is an effective tool for improving the rendering efficiency of your React application. One can use this type of hook to improve the application’s user experience, minimize its effort, and ensure that it remains quick and responsive even in the face of complex UI elements and huge data sets. So, if you want to take your React application to the next level, give `useDeferredValue` a try!
Share your thoughts in the comments
Please Login to comment...