What is the difference between the $parse, $interpolate and $compile services?
Last Updated :
20 Nov, 2023
In AngularJS applications, the $parse, $interpolate and $compile services are the most important components as these services are used for data binding. Here, the $parse is mainly responsible for parsing and evaluating the expressions in the context of the application’s scope. The $interpolate handles the interpolation of expressions and the $compile mainly compiles the HTML template and links.
In this article, we will see these concepts in more detail with their syntax, practical implementation, and the differences between these services with unique parameters.
$parse
The $parse service in AngularJS is mainly used to parse and evaluate the expressions that are in the context of the applications’ script. This enables us to dynamically evaluate the strings as AngularJS expressions, this also is useful in the task of custom validation, dynamical updating of data, and also in conditional rendering. $parse is mostly beneficial when we are working with directives, custom templates, or scenarios where we need to dynamically access and manipulate the data in our application.
Syntax
$parse(expression);
Example: The below example demonstrates the usage of $parse in AngularJS. Here, the $parse service is used to evaluate a user-entered Angular expression dynamically. The $parse service converts the input expression into a JavaScript function, which is then executed within a scope, and the result is displayed.
HTML
<!DOCTYPE html>
< html ng-app = "parseExampleApp" >
< head >
< script src =
</ script >
</ head >
< body ng-controller = "parseExampleController"
style="text-align: center;
background-color: #f0f0f0;
padding: 20px;
font-family: Arial, sans-serif;">
< h1 style = "color: green;" >
GeeksforGeeks
</ h1 >
< h3 >$parse Example</ h3 >
< input type = "text"
ng-model = "expression"
placeholder = "Enter Angular Expression"
style="padding: 5px;
width: 300px;
border: 1px solid #ccc;
border-radius: 3px;
margin-top: 10px;">
< button ng-click = "evaluateExpression()"
style="padding: 5px 15px;
background-color: #4CAF50;
color: white;
border: none;
border-radius: 3px;
cursor: pointer;
margin-top: 10px;">
Evaluate
</ button >
< div ng-show = "result !== undefined"
style = "margin-top: 20px;" >
< p >
The result of {{ expression }} is:
</ p >
< p style="font-size: 24px;
color: #4CAF50;">
{{ result }}
</ p >
</ div >
< script >
angular.module('parseExampleApp', [])
.controller('parseExampleController',
['$scope', '$parse', function ($scope, $parse) {
$scope.expression = '5 + 5';
$scope.evaluateExpression = function () {
var parsedExpression = $parse($scope.expression);
var scope = {};
$scope.result = parsedExpression(scope);
};
}]);
</ script >
</ body >
</ html >
|
Output:
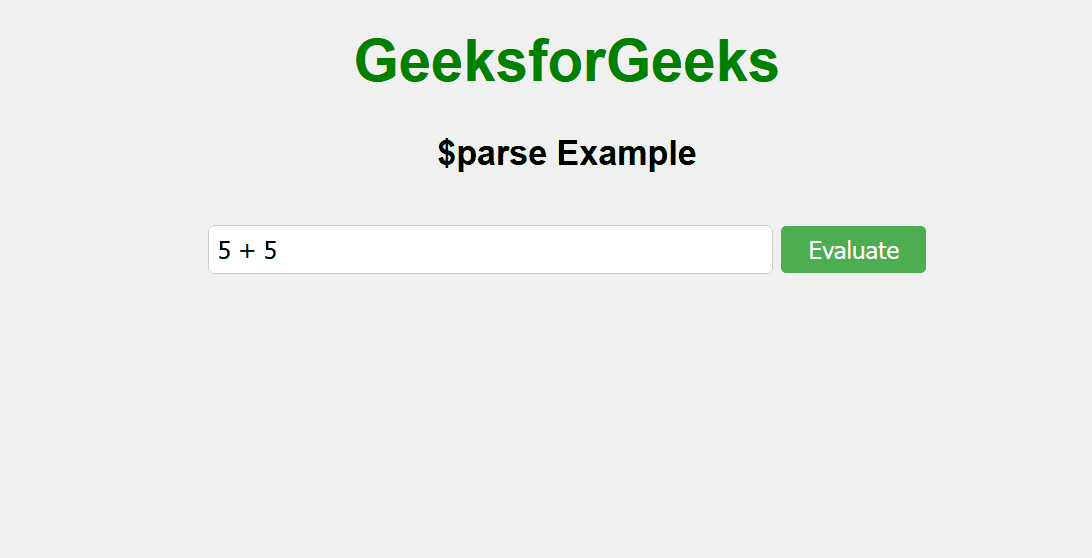
$interpolate
The $interpolate in AngularJS is used for string interpolation within the templates. This allows us to manually insert the dynamic data and the expressions into the HTML template, by creating the dynamic UI. The $interpolate service parses the template, by locating the expressions enclosed in the double curly braces (“{{ }}”) and replacing them with their corresponding values in the scope. $ interpolate makes combining static and dynamic content in templates easier by making the application more interactive.
Syntax
$interpolate(text)(scope);
Example: The below example demonstrates the usage of $interpolate in AngularJS. Here, the $interpolate is used to create a personalized message by dynamically inserting the user’s name and chosen color into a template string. When the “Generate Message” button is clicked, the interpolation function is invoked, replacing expressions like {{ userName }} and {{ userColor }} with the respective user inputs, resulting in a dynamic and styled message.
HTML
<!DOCTYPE html>
< html ng-app = "interpolateExampleApp" >
< head >
< script src =
</ script >
</ head >
< body ng-controller = "interpolateExampleController"
style="text-align: center;
background-color: #f0f0f0;
padding: 20px;
font-family: Arial, sans-serif;">
< h1 style = "color: green;" >
GeeksforGeeks
</ h1 >
< h3 >$interpolate Example</ h3 >
< label for = "name" >
Enter your name:
</ label >
< input type = "text"
id = "name"
ng-model = "userName"
style="padding: 5px;
width: 300px;
border: 1px solid #ccc;
border-radius: 3px;
margin-top: 10px;">< br >
< label for = "color" >
Choose your favorite color:
</ label >
< select id = "color"
ng-model = "userColor"
style="padding: 5px;
width: 100px;
border: 1px solid #ccc;
border-radius: 3px;
margin-top: 10px;">
< option value = "red" >Red</ option >
< option value = "green" >Green</ option >
< option value = "blue" >Blue</ option >
</ select >< br >
< button ng-click = "updateMessage()"
style="padding: 5px 15px;
background-color: #4CAF50;
color: white;
border: none;
border-radius: 3px;
cursor: pointer;
margin-top: 10px;">
Generate Message
</ button >
< div style = "margin-top: 20px;" >
< h2 >Your Personalized Message:</ h2 >
< h1 ng-style = "{color: userColor}" >
{{ personalizedMessage }}
</ h1 >
</ div >
< script >
angular.module('interpolateExampleApp', [])
.controller('interpolateExampleController',
['$scope', '$interpolate', function ($scope, $interpolate) {
$scope.userName = '';
$scope.userColor = 'black';
var interpolateFn =
$interpolate(
"Hello, {{ userName }}! Your favorite color is {{ userColor }}.");
$scope.updateMessage = function () {
$scope.personalizedMessage = interpolateFn($scope);
};
}]);
</ script >
</ body >
</ html >
|
Output:
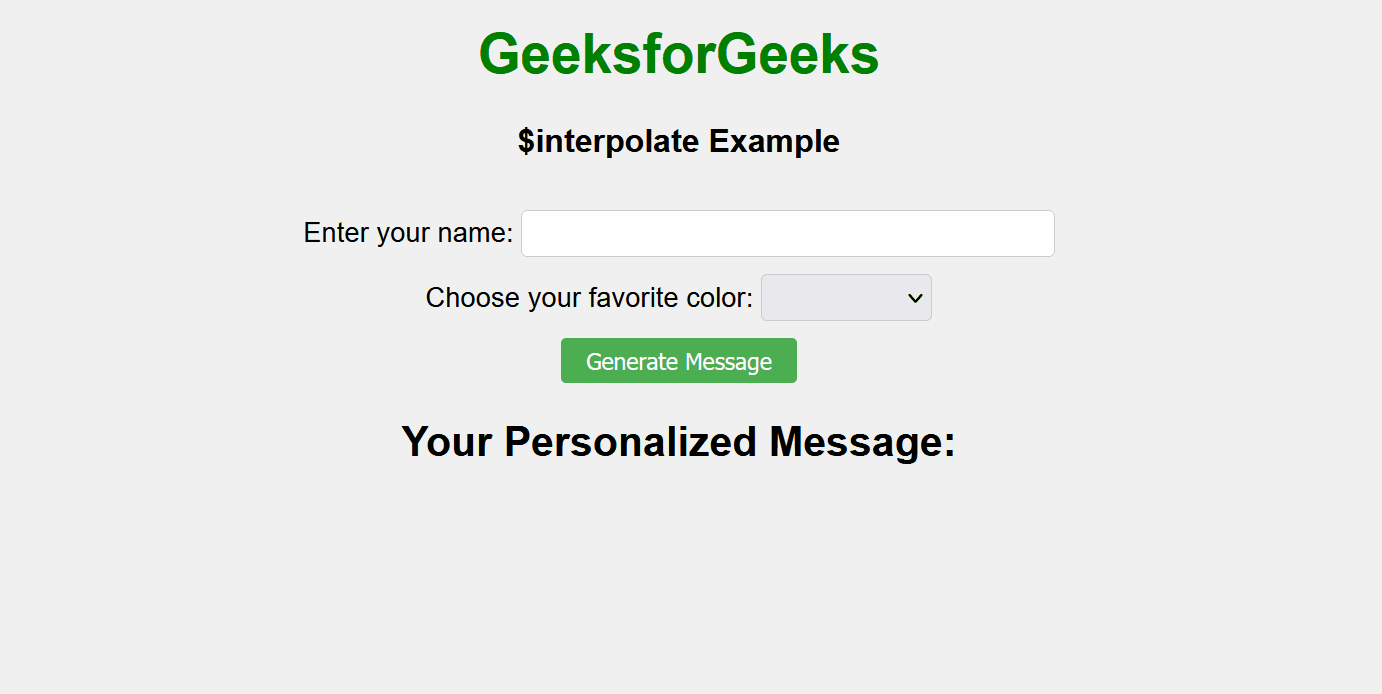
$compile
$compile service in AngularJS is used for the dynamic generating and linking of HTML templates with our applications. Using this service we can take the raw HTML templates and transform them into functions that can be linked with the scope. This enables the dynamic generation and the interaction. The $compile service processes and binds the HTML elements to the application’s scope and makes the application responsive. $compile service also the expressions match the changes and maintain the lifecycle of the directives.
Syntax
$compile(element)(scope);
Example: The below example demonstrates the usage of $compile in AngularJS. Here, the $compile service is used to dynamically create and link HTML templates for each added item. When the “Add Item” button is clicked, a new item template is compiled, and a child scope is created to bind the item’s data. The compiled item is then added to the list, demonstrating how $compile generates dynamic content.
HTML
<!DOCTYPE html>
< html ng-app = "compileExampleApp" >
< head >
< style >
.item {
background-color: #4CAF50;
color: white;
padding: 5px;
margin: 5px;
border-radius: 3px;
}
</ style >
< script src =
</ script >
</ head >
< body ng-controller = "compileExampleController"
style="text-align: center;
background-color: #f0f0f0;
padding: 20px;
font-family: Arial, sans-serif;">
< h1 style = "color: green;" >
GeeksforGeeks
</ h1 >
< h3 >$compile Example</ h3 >
< input type = "text"
ng-model = "newItemText"
placeholder = "Enter a new item"
style="padding: 5px;
width: 300px;
border: 1px solid #ccc;
border-radius: 3px;
margin-top: 10px;">
< button ng-click = "addItem()"
style="padding: 5px 15px;
background-color: #4CAF50;
color: white;
border: none;
border-radius: 3px;
cursor: pointer;
margin-top: 10px;">
Add Item
</ button >
< div id = "item-list"
style = "margin-top: 20px;" >
</ div >
< script >
angular.module('compileExampleApp', [])
.controller('compileExampleController',
['$scope', '$compile',
function ($scope, $compile) {
$scope.items = [];
$scope.addItem = function () {
var template =
'< div class = "item" >New item: {{ newItemText }}</ div >';
var childScope = $scope.$new();
childScope.newItemText = $scope.newItemText;
var compiledTemplate = $compile(template)(childScope);
var itemList = document.getElementById('item-list');
itemList.appendChild(compiledTemplate[0]);
$scope.newItemText = '';
};
}]);
</ script >
</ body >
</ html >
|
Output:
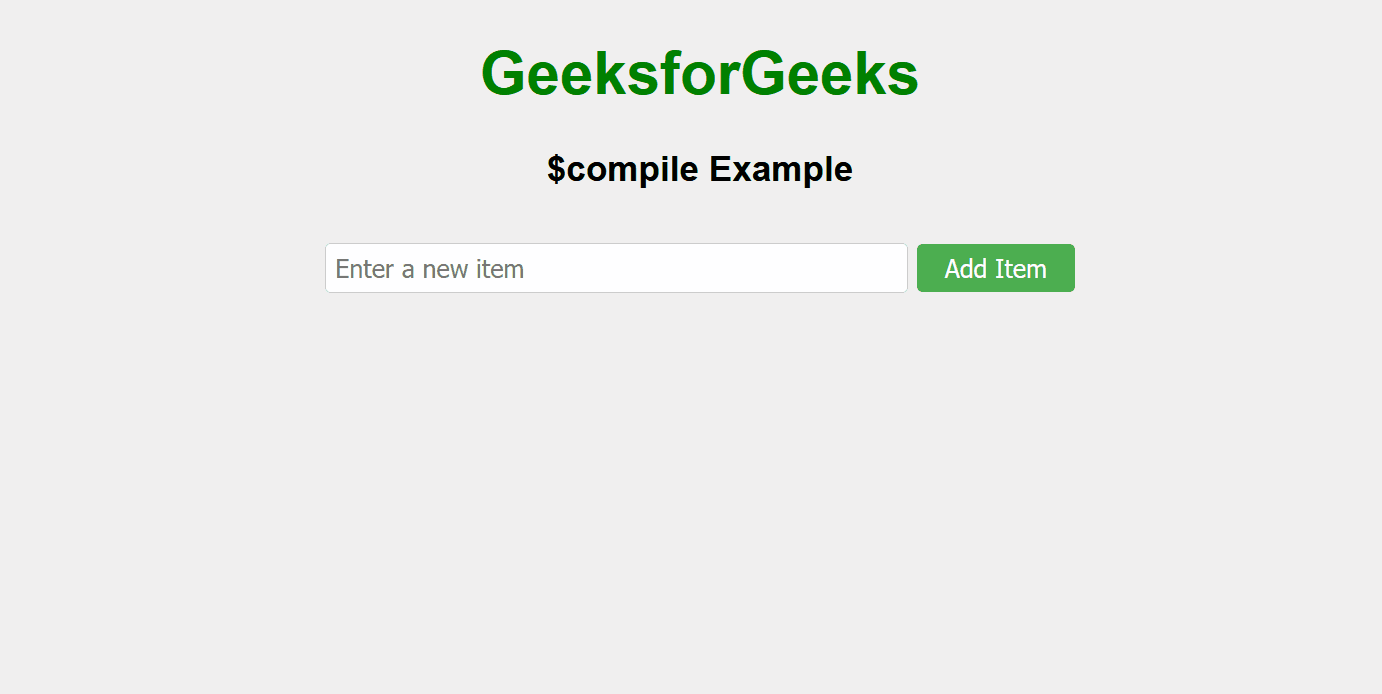
Difference Between the $parse, $interpolate and $compile Services
Basis
|
$parse
|
$interpolate
|
$compile
|
Input Expression |
String representation of an Angular expression |
A string containing expressions enclosed in double curly braces |
HTML or template with potential Angular directives and expressions |
Expression Binding |
Used for dynamic evaluation of expressions |
Interpolates expressions in template strings |
Links HTML templates with scope data and directives |
Directives |
Not directly related to directives |
Not directly related to directives |
Used in creating custom directives and manipulating the DOM |
Dynamically Updates |
No, $parse evaluates expressions but doesn’t update the DOM |
No, $interpolate updates text content but doesn’t compile and update DOM |
Yes, $compile compiles and updates the DOM based on the template |
Scope |
The scope object where the expression is evaluated |
The scope used for interpolation (implicitly based on the directive’s scope) |
The scope to which the compiled template is linked |
Share your thoughts in the comments
Please Login to comment...