What is the difference between parent() and parents() methods in jQuery ?
Last Updated :
22 Jul, 2021
The jQuery parent() and parents() methods return the elements which are ancestors of the DOM. It traverses upwards in the DOM for finding ancestors.
In this article, we will learn about the difference between parent() and parents() methods.
parent() Method: The parent() method traverse only one level before the DOM and returns the element that is the direct parent or nearest 1st ancestor of the selected element using jQuery.
Syntax:
$('selector').parent();
Example 1:
HTML
<!DOCTYPE html>
(ancestor-6 )
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta http-equiv = "X-UA-Compatible" content = "IE=edge" >
< meta name = "viewport"
content = "width=device-width, initial-scale=1.0" >
integrity = "sha256-/xUj+3OJU5yExlq6GSYGSHk7tPXikynS7ogEvDej/m4="
crossorigin = "anonymous" >
</ script >
< style >
h1 {
color: #006600;
}
body {
text-align: center;
}
div {
text-align: center;
padding: 5px;
border: 2px solid black;
border-radius: 5px;
margin: 5px;
}
p{
border: 2px solid black;
margin: 2px;
padding: 5px;
background-color: white;
}
/* The class that turns the div's
background colour to red */
.bg-blue {
background-color: blue;
}
</ style >
</ head >
< body >
< h1 >Geeks For Geeks(ancestor-5)</ h1 >
< div >
DIV-1(ancestor-4)
< div >
DIV-2(ancestor-3)
< div >
DIV-3(ancestor-2)
< div >
DIV-4 Direct parent of p(ancestor-1)
< p id = 'btn' >
This is geeks for geeks(Click
Me to find direct parent)
</ p >
</ div >
</ div >
</ div >
</ div >
< script >
$('p').click(function(){
$('p').parent().addClass('bg-blue');
});
</ script >
</ body >
</ html >
|
Output:
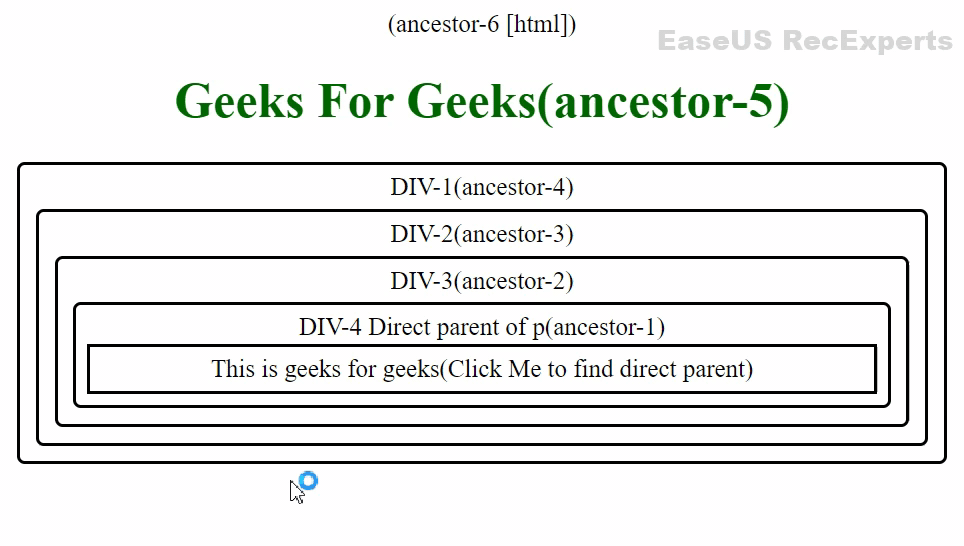
Direct Parent
If we observe this, the class ‘bg-blue‘ is added to the direct parent of p that is DIV-4 and change the background colour to blue as p has a white background colour, it remained white.
Example 2: The following code also shows the parent elements in green color for the child elements.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta http-equiv = "X-UA-Compatible" content = "IE=edge" >
< meta name = "viewport" content = "width=device-width, initial-scale=1.0" >
< title >Geeks for Geeks</ title >
letegrity = "sha256-/xUj+3OJU5yExlq6GSYGSHk7tPXikynS7ogEvDej/m4="
crossorigin = "anonymous" ></ script >
< style >
h2 {
color: #006600;
}
button {
color: white;
background-color: #006600;
width: 100px;
height: 30px;
}
#sublist2 {
color: red;
}
</ style >
</ head >
< body >
< h2 >GeeksforGeeks</ h2 >
< div >
< ul id = "list1" >
< li >
GrandParent
< ol id = "sublist1" >
< li >one</ li >
< li >two</ li >
< li >three</ li >
</ ol >
</ li >
< li >
Parent
< ol >
< li >three</ li >
< li >four</ li >
< li >five</ li >
< ol id = "sublist2" >
< li > Child</ li >
< li >six</ li >
< li >seven</ li >
< li >eight</ li >
</ ol >
</ ol >
</ li >
</ ul >
</ div >
< script >
$('ol#sublist2').parent().css('color', 'green');
</ script >
</ body >
</ html >
|
Output: The green color ordered list is the parent element for the child elements.
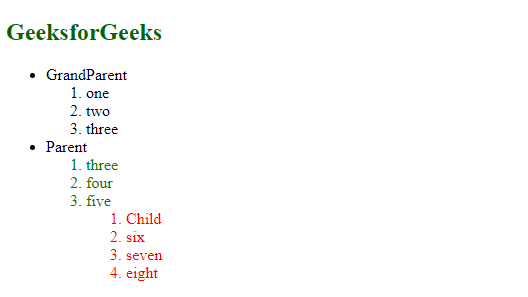
parents() Method: The parents() method traverse all the levels of elements upper than the selected element in the DOM and returns all the element that are ancestors of the selected element using jQuery.
Syntax:
$('selector').parents();
Example 1:
HTML
<!DOCTYPE html>
(ancestor-6 )
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta http-equiv = "X-UA-Compatible" content = "IE=edge" >
< meta name = "viewport"
content = "width=device-width, initial-scale=1.0" >
integrity = "sha256-/xUj+3OJU5yExlq6GSYGSHk7tPXikynS7ogEvDej/m4="
crossorigin = "anonymous" >
</ script >
< style >
h1 {
color: #006600;
}
body {
text-align: center;
}
div {
text-align: center;
padding: 5px;
border: 2px solid black;
border-radius: 5px;
margin: 5px;
}
p{
border: 2px solid black;
margin: 2px;
padding: 5px;
background-color: white;
}
/* The class that turns the div's background colour to red */
.bg-blue {
background-color: blue;
}
</ style >
</ head >
< body >
< h1 >Geeks For Geeks(ancestor-5)</ h1 >
< div >
DIV-1(ancestor-4)
< div >
DIV-2(ancestor-3)
< div >
DIV-3(ancestor-2)
< div >
DIV-4 Direct parent of p(ancestor-1)
< p id = 'btn' >
This is geeks for geeks(Click Me to find all
ancestors of p tag)
</ p >
</ div >
</ div >
</ div >
</ div >
< script >
$('p').click(function()
{
$('p').parents().addClass('bg-blue');
});
</ script >
</ body >
</ html >
|
Output:
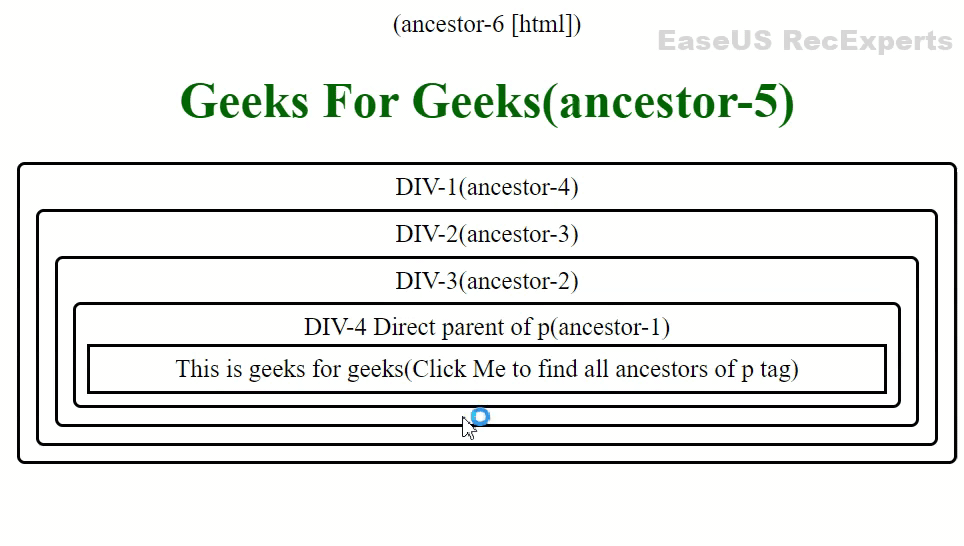
All ancestors
If we observe this, the class ‘bg-blue‘ is added to all the ancestors of p that is DIV-4, DIV-3, DIV-2, DIV-1,h1, HTML tag and change the background color to blue since p has a white background color, it remained white.
Example 2:
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta http-equiv = "X-UA-Compatible" content = "IE=edge" >
< meta name = "viewport"
content = "width=device-width, initial-scale=1.0" >
integrity = "sha256-/xUj+3OJU5yExlq6GSYGSHk7tPXikynS7ogEvDej/m4="
crossorigin = "anonymous" >
</ script >
< style >
h2 {
color: #006600;
}
button {
color: white;
background-color: #006600;
width: 100px;
height: 30px;
}
#sublist2 {
color: red;
}
</ style >
</ head >
< body >
< h2 >GeeksforGeeks</ h2 >
< div >
< ul id = "list1" >
< li >
GrandParent
< ol id = "sublist1" >
< li >one</ li >
< li >two</ li >
< li >three</ li >
</ ol >
</ li >
< li >
Parent
< ol >
< li >three</ li >
< li >four</ li >
< li >five</ li >
< ol id = "sublist2" >
< li > Child</ li >
< li >six</ li >
< li >seven</ li >
< li >eight</ li >
</ ol >
</ ol >
</ li >
</ ul >
</ div >
< script >
$('ol#sublist2').parents().css('color', 'green');
</ script >
</ body >
</ html >
|
Output: All the green-colored ordered lists are the parents for the child elements.
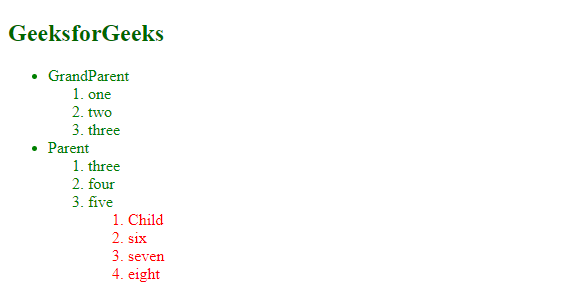
difference between parent() and parents() methods:
parent() Method |
parents() Method |
It only traverses one level up in the DOM of the selected element. |
It traverses all levels up in the DOM of the selected element until the root i.e HTML tag. |
It returns only an element that is the direct parent. |
It returns all elements that are ancestors to the selected element |
Share your thoughts in the comments
Please Login to comment...