What is the Difference Between $evalAsync and $timeout in AngularJS?
Last Updated :
27 Nov, 2023
AngularJS is a JavaScript framework, which may be utilized by including it in an HTML web page the usage of a <script> tag. AngularJS enables in extension the HTML attributes with the assistance of directives and binding of data to the HTML with expressions. It provides various tools for handling asynchronous tasks in web applications.
In this article, we will explore the differences between $evalAsync and $timeout in AngularJS and provide examples to illustrate their usage. Two commonly used methods for managing asynchronous operations in AngularJS are $evalAsync and $timeout. While both of these methods allow you to schedule code execution, they serve different purposes and behave differently.
AngularJS $evalAsync
The $evalAsync in AngularJS is a method used to schedule the execution of a function or expression asynchronously within the current digest cycle. It allows you to queue tasks that are closely related to the current application state, such as model updates or DOM modifications. Importantly, it does not introduce artificial delays, ensuring that code is executed in the right context without disrupting the ongoing digest cycle. This is particularly handy in directives, services, or controllers when we need to defer work that should be integrated smoothly into the application’s current state.
Syntax
Here’s a detailed breakdown of its syntax:
$scope.$evalAsync([expression]);
Parameters
- $scope: This is the reference to the AngularJS scope object to which you want to attach the $evalAsync function. The function will execute inside the context of this scope.
- expression (optional): An optional function or JavaScript expression to be executed during the next digest cycle. This function typically contains code that modifies the scope or model.
Example: In this example, we use $evalAsync to schedule a function for execution within the next digest cycle. Inside the function, we update the model ($scope.value) to 50. This ensures that the change is properly digested and reflected in the UI.
HTML
<!DOCTYPE html>
< html >
< head >
< title >$evalAsync Example</ title >
< script src =
</ script >
</ head >
< body ng-app = "evalAsyncApp"
style = "text-align: center" >
< div ng-controller = "EvalAsyncController" >
< h1 style = "color: green" >
GeeksforGeeks
</ h1 >
< h2 >$evalAsync usage</ h2 >
< p >Value: {{ value }}</ p >
< button ng-click = "updateValue()" >
Update Value
</ button >
</ div >
< script >
var evalAsyncApp =
angular.module('evalAsyncApp', []);
evalAsyncApp.controller('EvalAsyncController',
function ($scope) {
$scope.value = 42;
$scope.updateValue = function () {
$scope.$evalAsync(function () {
$scope.value = 50;
});
};
});
</ script >
</ body >
</ html >
|
Outout:
AngularJS $timeout
The $timeout in AngularJS is a method used to delay the execution of a function by a specified amount of time. That is beneficial when we want to introduce delays in our code or execute a function after a certain period, either within or outside the AngularJS digest cycle, like animations or timed operations. An optional parameter, invokeApply, can be set to true to trigger a new digest cycle after the function executes, ensuring that any changes made during the function are reflected in the application’s UI. The $timeout is versatile and permits developers to manage a wide range of asynchronous tasks, making it appropriate for scenarios that require both time delays and updates to the application’s data model or view.
Syntax
Here’s a detailed breakdown of its syntax:
$timeout([fn], [delay], [invokeApply], [pass]);
Parameters
- fn (required): The function to be executed after the specified delay. This function can contain any code you want to run.
- delay (required): The delay time in milliseconds before executing the function. After this delay, the provided function is invoked.
- invokeApply (optional, default: true): A boolean that determines whether to trigger a digest cycle after executing the function. If set to true, it ensures that AngularJS processes any model changes within the function. If set to false, no digest cycle is triggered.
- pass (optional): Any additional arguments you want to pass to the function when it’s executed.
Example: In this example, we use $timeout to delay the execution of a function by 2000 milliseconds (2 second). The function inside $timeout updates the model ($scope.value) to 50.
HTML
<!DOCTYPE html>
< html >
< head >
< title >$timeout Example</ title >
< script src =
</ script >
</ head >
< body ng-app = "timeoutApp"
style = "text-align: center" >
< div ng-controller = "TimeoutController" >
< h1 style = "color: green" >
GeeksforGeeks
</ h1 >
< h2 >$timeout usage</ h2 >
< p >Value: {{ value }}</ p >
< button ng-click = "updateValueWithTimeout()" >
Update Value
</ button >
</ div >
< script >
var timeoutApp =
angular.module('timeoutApp', []);
timeoutApp.controller('TimeoutController',
function ($scope, $timeout) {
$scope.value = 42;
$scope.updateValueWithTimeout = function () {
$timeout(function () {
$scope.value = 50;
}, 2000); // 2-second delay
};
});
</ script >
</ body >
</ html >
|
Output:
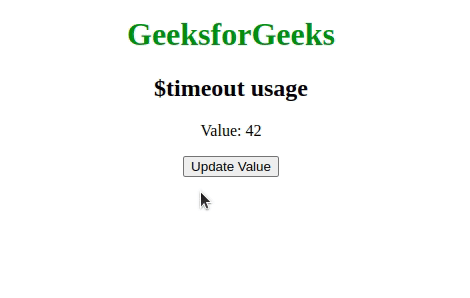
Difference between $evalAsync and $timeout
Ensures that changes to the model are reflected in the UI during the next digest cycle. |
Delays the execution of a function or code for a specified amount of time. |
Executes code in the next available digest cycle. |
Executes code after a specified delay. |
Schedules a function for execution during the next digest cycle. |
Schedules a function for execution after a delay. |
Does not return a promise. |
Returns a promise that can be used for task cancellation. |
Cannot be canceled. |
Can be canceled using the returned promise. |
Ideal for model updates that must immediately reflect in the UI. |
Useful for introducing delays, animation timing, or executing tasks outside the digest cycle. |
Share your thoughts in the comments
Please Login to comment...